// OrderData.h
#pragma once
#include <memory>
#include <iostream>
using namespace std;
#include <onepiece/models/OnePieceEnum.h>
class OrderData {
public:
OrderData() = default;
virtual ~OrderData() {
if (this->m_instrumentID) this->m_instrumentID.reset();
if (this->m_exchangeID) this->m_exchangeID.reset();
if (this->m_orderRef) this->m_orderRef.reset();
if (this->m_exchangeInstrument) this->m_exchangeInstrument.reset();
};
inline double LimitPrice() { return this->m_limitPrice; }
inline void setLimitPrice(const double limitPrice) { this->m_limitPrice = limitPrice; }
inline const shared_ptr<string> InstrumentID() { return this->m_instrumentID; }
inline void setInstrumentID(const shared_ptr<string> instrumentID) { this->m_instrumentID = instrumentID; }
inline const shared_ptr<string> ExchangeID() { return this->m_exchangeID; }
inline void setExchangeID(const shared_ptr<string> exchangeID) { this->m_exchangeID = exchangeID; }
inline const shared_ptr<string> ExchangeInstrument() { return this->m_exchangeInstrument; }
inline void setExchangeInstrument(const shared_ptr<string> exchangeInstrument) { this->m_exchangeInstrument = exchangeInstrument; }
inline int Lots() { return this->m_lots; }
inline void setLots(const int lots) { this->m_lots = lots; }
inline OPDirection Direction() { return this->m_direction; }
inline void setDirection(const OPDirection direction) { this->m_direction = direction; }
inline OPOpenClose CombOffsetFlag() { return this->m_combOffsetFlag; }
inline void setCombOffsetFlag(const OPOpenClose combOffsetFlag) { this->m_combOffsetFlag = combOffsetFlag; }
inline const shared_ptr<string> OrderRef() { return this->m_orderRef; }
inline void setOrderRef(shared_ptr<string> orderRef) { this->m_orderRef = orderRef; }
inline OPOrderStatus OrderStatus() { return this->m_orderStatus; }
inline void setOrderStatus(OPOrderStatus orderStatus) { this->m_orderStatus = orderStatus; }
private:
double m_limitPrice;
int m_lots;
shared_ptr<string> m_instrumentID;
shared_ptr<string> m_exchangeID;
shared_ptr<string> m_exchangeInstrument;
shared_ptr<string> m_orderRef;
OPDirection m_direction;
OPOpenClose m_combOffsetFlag;
OPOrderStatus m_orderStatus;
};
using OrderUPtr = unique_ptr<OrderData>;
using OrderSPtr = shared_ptr<OrderData>;
量化交易之One Piece篇 - OrderData.h - 订单模型
原创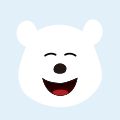
-
量化交易开发之基本语法(三)
本教程则是以量化的情景从零讲解python编程,所以将更适合想学做量化策略的人。
数据 变量名 python -
量化交易开发之函数API(四)
我们讲解一下python中的函数知识
API 数据 python -
量化交易开发之初识量化(一)
本系列课程将开启手把手保姆级实战课程,开发属于你自己的量化策略!!!
量化交易 策略因子 实战教学 -
量化交易之One Piece篇 - OnePieceEnum.h
【代码】量化交易之One Piece篇 - OnePieceEnum.h。
one piece #pragma -
量化交易之One Piece篇 - MarketCTP.h
【代码】量化交易之One Piece篇 - MarketCTP.h。
c++ linux one piece #include ios -
量化交易之One Piece篇 - 模板 - ITraderApi.h
【代码】量化交易之One Piece篇 - 模板 - ITraderApi.h。
one piece c++ #include #pragma Data -
量化交易之One Piece篇 - 模板 - ICoreListener.h
【代码】量化交易之One Piece篇 - 模板 - ICoreListener.h。
one piece c++ #include #pragma -
量化交易之One Piece篇 - 模板 - IPluginContext.h
【代码】量化交易之One Piece篇 - 模板 - IPluginContext.h。
c++ one piece #include ios #pragma -
量化交易之One Piece篇 - 模板 - IMarketApi.h
【代码】量化交易之One Piece篇 - 模板 - IMarketApi.h。
c++ one piece #pragma #include -
量化交易之One Piece篇 - TradeData.h - 成交回报模型
【代码】量化交易之One Piece篇 - TradeData - 成交回报模型。
c++ #include #pragma -
量化交易之One Piece篇 - one_piece_server.py(stable版)
【代码】量化交易之One Piece篇 - one_piece_server.py(stable版)
python 开发语言 GAP json 启动脚本 -
量化交易之One Piece篇 - 生成主力合约文件(.h文件)
【代码】量化交易之One Piece篇 - 生成主力合约文件。
onepiece #include ios #pragma -
量化交易之One Piece篇 - spdlog - 示例demo
【代码】量化交易之One Piece篇 - spdlog - 示例demo。
c++ 算法 开发语言 #include -
量化交易之One Piece篇 - AccountModel - 用来登录的账户模型
【代码】量化交易之One Piece篇 - AccountModel - 用来登录的账户模型。
算法 模型 json #include Parse -
量化交易之One Piece篇 - ContractData.h & ContractData.cc - 合约模型
【代码】量化交易之One Piece篇 - ContractData.h & ContractData.cc - 合约模型。
算法 one piece 模型 Data #include -
量化交易之One Piece篇 - StatusInfoFieldData.h - 错误信息的模型
【代码】量化交易之One Piece篇 - StatusInfoFieldData.h - 错误信息的模型。
算法 c++ 模型 #pragma #include