// .h
#ifndef LONGBEACH_SIGNALS_SIGLASTTRADEDQUANTITY_H
#define LONGBEACH_SIGNALS_SIGLASTTRADEDQUANTITY_H
#include <longbeach/core/ptime.h>
#include <longbeach/clientcore/PriceProvider.h>
#include <longbeach/clientcore/PriceSizeProviderSpec.h>
#include <longbeach/clientcore/TickProvider.h>
#include <longbeach/signals/Signal.h>
#include <longbeach/signals/SignalSpec.h>
namespace longbeach {
namespace signals {
template <typename T> int sgn(T val) {
return (T(0) < val) - (val < T(0));
}
class TradedQuantity
{
public:
TradedQuantity( const TradeTick& trade_tick, const IBookPtr book, const double last_best_bid, const double last_best_ask, const double last_midprice, const boost::optional<double> notional_price );
const bool isExpired( const timeval_t expiration_time ) const { return (m_tradeTime <= expiration_time);}
const bool inWindow(const timeval_t current_time, ptime_duration_t window_duration) const {
timeval_t cutoff_time = current_time - window_duration;
return (m_tradeTime >= cutoff_time && m_tradeTime<=current_time);
}
const int32_t getTradedQuantity() const { return m_tradedQuantity;};
const timeval_t getTradeTime() const { return m_tradeTime;};
private:
timeval_t m_tradeTime;
int32_t m_tradedQuantity;
};
class RollingWindow
{
public:
RollingWindow( longbeach::ptime_duration_t window_length, double smoothing_factor );
virtual ~RollingWindow(){};
virtual void update(TradedQuantity traded_quantity);
virtual double getSignal() const;
void reset();
void expireTradedQuantities(const timeval_t current_time);
protected:
double smooth( double old_value, double new_value, double smoothing_factor);
longbeach::ptime_duration_t m_length;
double m_signal;
private:
std::vector<TradedQuantity> m_tradedQuantities;
double m_expireSmoothingFactor;
double m_smoothExpiryAdjustment;
};
class SigLastTradedQuantity: public SignalStateImpl, private IClockListener, private ITickListener, private IBookListener
{
public:
SigLastTradedQuantity( const instrument_t& instr, const std::string &desc, ClientContextPtr cc, ClockMonitorPtr spCM, const std::vector<longbeach::ptime_duration_t>& vWindowDurations,
const double expire_smoothing_factor, IBookPtr spBook, ITickProviderCPtr spTickProvider, ReturnMode returnMode );
/// Destructor
virtual ~SigLastTradedQuantity();
const std::vector<longbeach::ptime_duration_t>& getWindowDurations() const { return m_vWindowDurations; }
private:
// IClockListener interface
virtual void onWakeupCall( const timeval_t& ctv, const timeval_t& swtv, int reason, void* pData);
// IBookListener interface
/// Invoked when the subscribed Book changes.
/// The levelChanged entries are negative if there is no change, or a 0-based depth.
virtual void onBookChanged( const IBook* pBook, const Msg* pMsg, int32_t bidLevelChanged, int32_t askLevelChanged );
/// Invoked when the subscribed Book is flushed.
virtual void onBookFlushed( const IBook* pBook, const Msg* pMsg ) {};
/// This will be called when the ITickProvider's receives a new TradeTick.
/// That last trade tick is passed.
/// Spurious calls are allowed. tp will never be NULL.
virtual void onTickReceived( const ITickProvider* tp, const TradeTick& tick );
// we don't care about this
virtual void onTickVolumeUpdated( const ITickProvider* tp, uint64_t totalVolume ) {};
void reset();
virtual void recomputeState() const;
virtual void updateState() const ;
protected:
ClockMonitorPtr m_spCM;
IBookPtr m_spBook;
ITickProviderCPtr m_spTickProvider;
// Subscription m_spBookSub, m_spTickProviderSub;
std::vector<ptime_duration_t> m_vWindowDurations;
boost::optional<double> m_lotSize;
std::vector<RollingWindow*> m_rollingWindows;
double m_currentBestBidPrice;
double m_currentBestAskPrice;
double m_currentMidPrice;
double m_lastBestBidPrice;
double m_lastBestAskPrice;
double m_lastMidPrice;
timeval_t m_lastBookUpdateTime;
ReturnMode m_returnMode;
};
LONGBEACH_DECLARE_SHARED_PTR( SigLastTradedQuantity );
/// SignalSpec for SigLastTradedQuantity
class SigLastTradedQuantitySpec : public SignalSpec
{
public:
LONGBEACH_DECLARE_SCRIPTING();
SigLastTradedQuantitySpec(): m_expireSmoothingFactor( 0.20 ) {};
SigLastTradedQuantitySpec(const SigLastTradedQuantitySpec &other);
virtual instrument_t getInstrument() const { return m_inputBook->getInstrument(); }
virtual ISignalPtr build(SignalBuilder *builder) const;
virtual void checkValid() const;
virtual void hashCombine(size_t &result) const;
virtual bool compare(const ISignalSpec *other) const;
virtual void print(std::ostream &o, const LuaPrintSettings &ps) const;
virtual void getDataRequirements(IDataRequirements *rqs) const;
virtual SigLastTradedQuantitySpec *clone() const;
IBookSpecPtr m_inputBook;
source_t m_tickSource;
std::vector<longbeach::ptime_duration_t> m_vWindowDurations;
double m_expireSmoothingFactor;
ReturnMode m_returnMode;
};
LONGBEACH_DECLARE_SHARED_PTR( SigLastTradedQuantitySpec );
class WindowAtTime
{
public:
WindowAtTime( const double window_total, const timeval_t sample_time): m_total(window_total), m_time(sample_time) {};
const double getTotal() const { return m_total;};
const timeval_t getTime() const { return m_time;};
private:
double m_total;
timeval_t m_time;
};
class BaselineRollingWindow: public RollingWindow
{
public:
BaselineRollingWindow( longbeach::ptime_duration_t window_length, double expire_smoothing_factor, longbeach::ptime_duration_t sample_period, uint32_t history_length, double smoothing_factor );
virtual ~BaselineRollingWindow(){};
virtual void update(TradedQuantity traded_quantity);
virtual double getSignal() const;
private:
uint32_t m_numberOfHistorySamples;
longbeach::ptime_duration_t m_samplePeriod;
std::vector<TradedQuantity> m_tradedQuantities;
double m_smoothingFactor;
double m_smoothedTotalAtStart;
std::deque<WindowAtTime> m_sampledHistory;
std::deque<WindowAtTime> m_recentHistory;
};
class SigBaselineLastTradedQuantity: public SigLastTradedQuantity
{
public:
SigBaselineLastTradedQuantity( const instrument_t& instr, const std::string &desc, ClientContextPtr cc, ClockMonitorPtr spCM,
const std::vector<longbeach::ptime_duration_t>& vWindowDurations,
const uint32_t window_history_length, const uint32_t windows_to_sample,
const double cutoff, const double expire_smoothing_factor,
const double smoothing_factor, IBookPtr spBook, ITickProviderCPtr spTickProvider);
/// Destructor
virtual ~SigBaselineLastTradedQuantity() {};
private:
virtual void updateState() const;
private:
double m_cutoff;
};
LONGBEACH_DECLARE_SHARED_PTR( SigBaselineLastTradedQuantity );
/// SignalSpec for SigBaselineLastTradedQuantity
class SigBaselineLastTradedQuantitySpec : public SignalSpec
{
public:
LONGBEACH_DECLARE_SCRIPTING();
SigBaselineLastTradedQuantitySpec()
: m_numberOfHistorySamples( 0 )
, m_cutoff(0.0)
, m_windowsToSample( 0 )
, m_expireSmoothingFactor( 0.20 )
, m_smoothingFactor( 0.12 )
{};
SigBaselineLastTradedQuantitySpec(const SigBaselineLastTradedQuantitySpec &other);
virtual instrument_t getInstrument() const { return m_inputBook->getInstrument(); }
virtual ISignalPtr build(SignalBuilder *builder) const;
virtual void checkValid() const;
virtual void hashCombine(size_t &result) const;
virtual bool compare(const ISignalSpec *other) const;
virtual void print(std::ostream &o, const LuaPrintSettings &ps) const;
virtual void getDataRequirements(IDataRequirements *rqs) const;
virtual SigBaselineLastTradedQuantitySpec *clone() const;
IBookSpecPtr m_inputBook;
source_t m_tickSource;
std::vector<longbeach::ptime_duration_t> m_vWindowDurations;
uint32_t m_numberOfHistorySamples;
double m_cutoff;
uint32_t m_windowsToSample;
double m_expireSmoothingFactor;
double m_smoothingFactor;
};
LONGBEACH_DECLARE_SHARED_PTR( SigBaselineLastTradedQuantitySpec );
} // namespace signals
} // namespace longbeach
#endif // LONGBEACH_SIGNALS_SIGLASTTRADEDQUANTITY_H
量化交易之HFT篇 - long beach - SigLastTradedQuantity.h
原创
©著作权归作者所有:来自51CTO博客作者ErwinSmith的原创作品,请联系作者获取转载授权,否则将追究法律责任
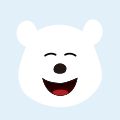
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
量化交易开发之基本语法(三)
本教程则是以量化的情景从零讲解python编程,所以将更适合想学做量化策略的人。
数据 变量名 python -
量化交易开发之函数API(四)
我们讲解一下python中的函数知识
API 数据 python -
量化交易开发之初识量化(一)
本系列课程将开启手把手保姆级实战课程,开发属于你自己的量化策略!!!
量化交易 策略因子 实战教学 -
量化交易之HFT篇 - long beach - SigBookBias.h
【代码】量化交易之HFT篇 - long beach - SigBookBias.h。
c++ 开发语言 #include #define ide -
量化交易之HFT篇 - long beach - SigMACD.h
【代码】量化交易之HFT篇 - long beach - SigMACD.h。
c++ hft #include ide #ifndef -
量化交易之HFT篇 - long beach - SigMa.h
【代码】量化交易之HFT篇 - long beach - SigMa.h。
c++ hft #include ide #ifndef -
量化交易之HFT篇 - long beach - SigKalmanFilter.h
【代码】量化交易之HFT篇 - long beach - SigKalmanFilter.h。
hft c++ ide #include #ifndef -
量化交易之HFT篇 - long beach - SigDiff.h
【代码】量化交易之HFT篇 - long beach - SigDiff.h。
hft ide #include #ifndef -
量化交易之HFT篇 - long beach - SigBook.h
【代码】量化交易之HFT篇 - long beach - SigBook.h。
hft ide hg #include -
量化交易之HFT篇 - long beach - SampleAndHoldSignal.h
【代码】量化交易之HFT篇 - long beach - SampleAndHoldSignal.h。
hft #include #ifndef #define -
量化交易之HFT篇 - long beach - SigBookBiasL2.h
【代码】量化交易之HFT篇 - long beach - SigBookBiasL2.h。
hft #include ide #ifndef -
量化交易之HFT篇 - long beach - SigMACD.cc
【代码】量化交易之HFT篇 - long beach - SigMACD.cc。
c++ hft List ide #include -
量化交易之HFT篇 - long beach - SigKalmanFilter.cc
【代码】量化交易之HFT篇 - long beach - SigKalmanFilter.cc。
c++ hft List #include lua -
量化交易之HFT篇 - long beach - SigBook.cc
【代码】量化交易之HFT篇 - long beach - SigBook.cc。
hft #include hg ide -
量化交易之HFT篇 - long beach - SigMa.cc
【代码】量化交易之HFT篇 - long beach - SigMa.cc。
hft c++ List #include ide -
量化交易之HFT篇 - long beach - SampleAndHoldSignal.cc
【代码】量化交易之HFT篇 - long beach - SampleAndHoldSignal.cc。
c++ 哈希算法 开发语言 lua #include -
量化交易之HFT篇 - long beach - SigDiff.cc
【代码】量化交易之HFT篇 - long beach - SigDiff.cc。
hft ide #include List -
量化交易之HFT篇 - long beach - SigBookBias.cc
【代码】量化交易之HFT篇 - long beach - SigBookBias.cc。
c++ 开发语言 lua #include ide -
量化交易之HFT篇 - long beach - SigBookBiasL2.cc
【代码】量化交易之HFT篇 - long beach - SigBookBiasL2.cc。
c++ java ajax #include ide -
python certifi是什么模块
通过例程你能看到python的内置类型和types对应的类型。本文文档是汇总。例程是新编写,稍微多,耐心看。types模块 发布日期 2018/6/14 修改日期 2018/11/17 https://
python certifi是什么模块 生成器 python 类方法