python 运行后出现core dump产生core.**文件,可通过gdb来调试
Using GDB with a core dump
having found build/python/core.22608, we can now launch GDB:
gdb programname coredump
i.e.
gdb/usr/bin/python2 build/python/core.22608A lot of information might scroll by.
At the end, you're greeted by the GDB prompt:
(gdb)
Getting a backtrace
Typically, you'd just get a backtrace (or shorter, bt). A backtrace is simply the hierarchy of functions that were called.
(gdb)bt
[...] skipped,
Frame #2 and following definitely look like they're part of the Python implementation -- that sounds bad, because GDB doesn't itself know how to debug python, but luckily, there's an extension to do that. So we can try to use py-bt:
(gdb) py-bt
If we get a undefined command error, we must stop here andmake sure that the python development package is installed (python-devel on Redhatoids, python2.7-dev on Debianoids); for some systems, you should append the content of /usr/share/doc/{python-devel,python2.7-dev}/gdbinit[.gz] to your ~/.gdbinit, and re-start gdb.
The output of py-bt now states clearly which python lines correspond to which stack frame (skipping those stack frames that are hidden to python, because they are in external libraries or python-implementation routines)
...
分析程序的性能可以归结为回答四个基本问题:
正运行的多快
速度瓶颈在哪里
内存使用率是多少
内存泄露在哪里
用 time 粗粒度的计算时间
$ time python yourprogram.py
real 0m1.028s
user 0m0.001s
sys 0m0.003s
上面三个输入变量的意义在文章 stackoverflow article 中有详细介绍。简单的说:
real - 表示实际的程序运行时间
user - 表示程序在用户态的cpu总时间
sys - 表示在内核态的cpu总时间
通过sys和user时间的求和,你可以直观的得到系统上没有其他程序运行时你的程序运行所需要的CPU周期。
若sys和user时间之和远远少于real时间,那么你可以猜测你的程序的主要性能问题很可能与IO等待相关。
使用计时上下文管理器进行细粒度计时
我们的下一个技术涉及访问细粒度计时信息的直接代码指令。这是一小段代码,我发现使用专门的计时测量是非常重要的:
timer.py
importtimeclassTimer(object):def __init__(self, verbose=False):
self.verbose=verbosedef __enter__(self):
self.start=time.time()returnselfdef __exit__(self, *args):
self.end=time.time()
self.secs= self.end -self.start
self.msecs= self.secs * 1000 #millisecs
ifself.verbose:print 'elapsed time: %f ms' % self.msecs
为了使用它,使用 Python 的 with 关键字和 Timer 上下文管理器来包装你想计算的代码。当您的代码块开始执行,它将照顾启动计时器,当你的代码块结束的时候,它将停止计时器。
这个代码片段示例:
from timer importTimerfrom redis importRedis
rdb=Redis()
with Timer() as t:
rdb.lpush("foo", "bar")print "=> elasped lpush: %s s" %t.secs
with Timer() as t:
rdb.lpop("foo")print "=> elasped lpop: %s s" % t.secs
我经常将这些计时器的输出记录到文件中,这样就可以观察我的程序的性能如何随着时间进化。
使用分析器逐行统计时间和执行频率
Robert Kern有一个称作line_profiler的不错的项目,我经常使用它查看我的脚步中每行代码多快多频繁的被执行。
想要使用它,你需要通过pip安装该python包:
pip install line_profiler
安装完成后,你将获得一个新模块称为 line_profiler 和 kernprof.py 可执行脚本。
为了使用这个工具,首先在你想测量的函数上设置 @profile 修饰符。不用担心,为了这个修饰符,你不需要引入任何东西。kernprof.py 脚本会在运行时自动注入你的脚本。
primes.py
@profiledefprimes(n):if n==2:return [2]elif n<2:return[]
s=range(3,n+1,2)
mroot= n ** 0.5half=(n+1)/2-1i=0
m=3
while m <=mroot:ifs[i]:
j=(m*m-3)/2s[j]=0while j
s[j]=0
j+=m
i=i+1m=2*i+3
return [2]+[x for x in s ifx]
primes(100)
一旦你得到了你的设置了修饰符 @profile 的代码,使用 kernprof.py 运行这个脚本。
kernprof.py -l -v fib.py
-l 选项告诉 kernprof 把修饰符 @profile 注入你的脚本,-v 选项告诉 kernprof 一旦你的脚本完成后,展示计时信息。这是一个以上脚本的类似输出:
Wrote profile results to primes.py.lprof
Timer unit: 1e-06s
File: primes.py
Function: primes at line2Totaltime: 0.00019s
Line # Hits Time Per Hit%Time Line Contents==============================================================
2@profile3def primes(n):4 1 2 2.0 1.1 if n==2:5 return [2]6 1 1 1.0 0.5 elif n<2:7return []8 1 4 4.0 2.1 s=range(3,n+1,2)9 1 10 10.0 5.3 mroot = n ** 0.5
10 1 2 2.0 1.1 half=(n+1)/2-1
11 1 1 1.0 0.5 i=0
12 1 1 1.0 0.5 m=3
13 5 7 1.4 3.7 while m <=mroot:14 4 4 1.0 2.1 ifs[i]:15 3 4 1.3 2.1 j=(m*m-3)/2
16 3 4 1.3 2.1 s[j]=0
17 31 31 1.0 16.3 while j
<
<
测试电脑性能的python 测试电脑性能的代码
转载文章标签 测试电脑性能的python python测试电脑性能的代码 python 修饰符 GDB 文章分类 Python 后端开发
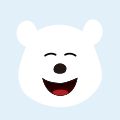
-
linux如何登录hdfs查看文件
HDFS shell 命令1、HDFS Shell 命令分类1.1文件系统操作命令(hdfs dfs) 解决文件的添加、删除、查看、移动等操作问题 1.2文件系统管理命令(hdfs dfsadmin) 解决数据节点详情查看、文件目录配额、安全模式等管理操作问题2、hdfs dfs 常用命令hdfs dfs 与 hadoop fs 对等,只是一个先后推荐使用的区别,目前推荐使用 hdfs dfs。
linux如何登录hdfs查看文件 hdfs shell命令 hadoop core-hdfs hdfs html