#include<iostream>
#include<vector>
#include<iterator>
#include<algorithm>
#include<time.h>
using namespace std;
template<class T>
class CMP //抽象操作符类
{
public:
virtual bool operator()(const T&one,const T&other)=0; //纯虚函数,重载操作符()
};
template<class T>
class LessThan:public CMP<T> //小于操作符类
{
public:
bool operator()(const T&one,const T&other)
{
return one<other;
}
};
template<class T>
class GreatThan:public CMP<T>
{
public:
bool operator()(const T&one,const T&other)
{
return one>other;
}
};
template<class Iterator,class T> //待排序元素的类型
class Sort //抽象排序类
{
protected:
Iterator array; //指向待排序区间的开始指针
public:
virtual void operator()(const Iterator &beg,const Iterator &end,CMP<T> &cmp)=0;
};
template<class Iterator,class T>
class QuickSort:public Sort<Iterator,T>
{
private:
int low,high; //指向待排序区间开始和结束
int Partition(int low,int high,CMP<T>&cmp); //将区间[low...high]划分成[low...pivot],[pivot+1...high]
int RandPos(int low,int high); //在low...high之间返回一个随机的位置
void Exchange(int i,int j); //交换位置i和位置j的两个元素
void Sort_Function(int low,int high,CMP<T>&cmp); //将区间[low...high]归并排序
public:
void operator()(const Iterator &beg,const Iterator &end,CMP<T> &cmp)
{
low=0;
high=end-beg-1;
array=beg;
Sort_Function(low,high,cmp);
}
};
template<class Iterator,class T>
int QuickSort<Iterator,T>::RandPos(int low,int high)
{
int n=high-low+1;
srand((unsigned)time(0));
int offset=rand()%n;
if(offset==n) offset--;
int pos=low+offset;
if(pos<=high && pos>=low) return pos;
else return low;
}
template<class Iterator,class T>
void QuickSort<Iterator,T>::Exchange(int i,int j)
{
T tmp;
tmp=array[i];
array[i]=array[j];
array[i]=tmp;
}
template<class Iterator,class T>
int QuickSort<Iterator,T>::Partition(int low,int high,CMP<T> &cmp)
{
int pos=RandPos(low,high);
Exchange(low,pos);
int i,j;
i=low;
j=high;
T tmp=array[low];
while(i<j)
{
while(i<j && array[j]>tmp) j--;
if(i<j) array[i++]=array[j];
while(i<j && array[i]<tmp) i++;
if(i<j) array[j--]=array[i];
}
array[i]=tmp;
return i;
}
template<class Iterator,class T>
void QuickSort<Iterator,T>::Sort_Function(int low,int high,CMP<T> &cmp)
{
if(low<high)
{
int pivot=Partition(low,high,cmp);
Sort_Function(low,pivot-1,cmp);
Sort_Function(pivot+1,high,cmp);
}
}
template<class Iterator,class T>
void Quick_Sort(const Iterator &beg,const Iterator &end,CMP<T>&cmp)
{
QuickSort<Iterator,T> ms;
ms(beg,end,cmp);
}
void main()
{
vector<int>a;
srand((unsigned)time(0));
const int n=88;
int x;
for(int i=0;i<n;i++)
{
x=rand()%n;
a.push_back(x);
}
Quick_Sort<vector<int>::iterator,int>(a.begin(),a.end(),GreatThan<int>());
//Merge_Sort<vector<int>::iterator,int>(a.begin(),a.end(),LessThan<int>());
copy(a.begin(),a.end(),ostream_iterator<int>(cout," "));
cout<<endl;
}
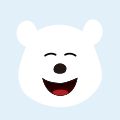
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
排序算法之计数排序的优化
排序算法之计数排序的优化
数组 计数排序 最小值 -
算法导论7 快速排序和随机化快速排序
《算法导论》第7章内容核心思想是分治,把最后的元素x放在中间,使得[i] =
python 算法 ci 随机化 算法导论 -
随机化乱搞♂
一些可以随机化的题
随机化 #define git #include c++