package com.shrimpking.t2;
import javax.sound.midi.Soundbank;
import java.util.BitSet;
/**
* Created by IntelliJ IDEA.
*
* @Author : Shrimpking
* @create 2024/9/11 11:43
*/
public class Test1
{
public static final int INSERT_FLAG = 1; //0001
public static final int UPDATE_FLAG = 2; //0010
public static final int DELETE_FLAG = 4; //0100
public static final int SELECT_FLAG = 8; //1000
public static void main(String[] args)
{
//同时拥有插入和删除权限,可以进行 或操作
int permission = INSERT_FLAG | DELETE_FLAG;
System.out.println(Integer.toBinaryString(permission)); //0101
// 0101 & 0001 -> 0001
if((permission & INSERT_FLAG) == INSERT_FLAG){
System.out.println("可以插入");
}else{
System.out.println("无插入权限");
}
// 0101 & 0010 -> 0000
if((permission & UPDATE_FLAG) == UPDATE_FLAG){
System.out.println("可以修改");
}else {
System.out.println("无修改权限");
}
// 0101 & 0100 -> 0100
if((permission & DELETE_FLAG) == DELETE_FLAG){
System.out.println("可以删除");
}else {
System.out.println("无删除权限");
}
// 0101 & 1000 -> 0000
if((permission & SELECT_FLAG) == SELECT_FLAG){
System.out.println("可以查询");
}else {
System.out.println("无查询权限");
}
//去掉删除权限 ,取反后再 与操作
permission = permission & ~DELETE_FLAG;
System.out.println(Integer.toBinaryString(permission)); // 0001
System.out.println("-------------------------");
//使用BitSet 实现相同功能
BitSet bs = new BitSet();
// 0 插入, 1 修改 ,2 删除, 3 查询
bs.set(0);
bs.set(2);
if(bs.get(0) == true){
System.out.println("有插入权限");
}
if(bs.get(1) == true){
System.out.println("有修改权限");
}
if(bs.get(2) == true){
System.out.println("有删除权限");
}
if(bs.get(3) == true){
System.out.println("有查询权限");
}
//去掉权限
bs.clear(2);
bs.set(2,false);
System.out.println(bs);
}
}
BitSet类的理解( &操作的一点感悟)
原创
©著作权归作者所有:来自51CTO博客作者虾米大王的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:CreateStream
下一篇:UseHashMap
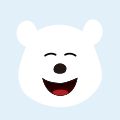
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
工作3年的一点感悟
日子过的真快,还记得3年前自己刚刚从培训机构学了4个月出来找工作的样子。每天睁开眼以后就是投简历,等电话,去
慕课网 前端开发 jquery