#define is unsafe
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 255 Accepted Submission(s): 153
Problem Description
Have you used #define in C/C++ code like the code below?
#include <stdio.h>
#define MAX(a , b) ((a) > (b) ? (a) : (b))
int main()
{
printf("%d\n" , MAX(2 + 3 , 4));
return 0;
}
Run the code and get an output: 5, right?
You may think it is equal to this code:
#include <stdio.h>
int max(a , b) { return ((a) > (b) ? (a) : (b)); }
int main()
{
printf("%d\n" , max(2 + 3 , 4));
return 0;
}
But they aren't.Though they do produce the same anwser , they work in two different ways.
The first code, just replace the MAX(2 + 3 , 4) with ((2 + 3) > (4) ? (2 + 3) : 4), which calculates (2 + 3) twice.
While the second calculates (2 + 3) first, and send the value (5 , 4) to function max(a , b) , which calculates (2 + 3) only once.
What about MAX( MAX(1+2,2) , 3 ) ?
Remember "replace".
First replace: MAX( (1 + 2) > 2 ? (1 + 2) : 2 , 3)
Second replace: ( ( ( 1 + 2 ) > 2 ? ( 1 + 2 ) : 2 ) > 3 ? ( ( 1 + 2 ) > 2 ? ( 1 + 2 ) : 2 ) : 3).
The code may calculate the same expression many times like ( 1 + 2 ) above.
So #define isn't good.In this problem,I'll give you some strings, tell me the result and how many additions(加法) are computed.
Input
The first line is an integer T(T<=40) indicating case number.
The next T lines each has a string(no longer than 1000), with MAX(a,b), digits, '+' only(Yes, there're no other characters).
In MAX(a,b), a and b may be a string with MAX(c,d), digits, '+'.See the sample and things will be clearer.
Output
For each case, output two integers in a line separated by a single space.Integers in output won't exceed 1000000.
Sample Input
6
MAX(1,0)
1+MAX(1,0)
MAX(2+1,3)
MAX(4,2+2)
MAX(1+1,2)+MAX(2,3)
MAX(MAX(1+2,3),MAX(4+5+6,MAX(7+8,9)))+MAX(10,MAX(MAX(11,12),13))
Sample Output
1 0
2 1
3 1
4 2
5 2
28 14
/*
hdoj 3350 栈的模拟题
MAX(1+1,2)+MAX(2,3)
读入整个字符串:
遇到MAX跳过,
1.遇到'(','+' 入符号栈
2.遇到数字 入数字栈
3,遇到','表示max的一半已经进入
判断符号栈顶是否为'+', 是,就要弹出2个数字操作+
4,遇到')',表示max结束,就要操作比较大小
但是左边仍然有可能有+的操作 ,
所以,先要判断符号栈顶是否为'+', 是,就要弹出2个数字操作+
然后开始比较大小:
注意这里默认的','前后的+操作为0,
若有一方为1,且是比较大的数,则操作是需要乘以2的
MAX(1+2,2)==>max(3,1)==>3 操作了2次+,同下:
( 1 + 2 ) > 2 ? ( 1 + 2 ) : 2
最后结束 仍然要判断符号栈顶是否有+操作,就是max中间的+
*/
#include<iostream>
#include<stdio.h>
#include<string.h>
#include<stack>
using namespace std;
struct node{
int x,count;
};
stack<node> ss;
stack<char> oper;
char str[1001];
int main()
{
int t,len,i,sum;
node tmp,tmp1,tmp2;
scanf("%d",&t);
while(t--)
{
scanf("%s",str);
len=strlen(str);
for(i=0;i<len;i++)
{
if(str[i]>='0'&&str[i]<='9')
{
sum=0;
while(str[i]>='0'&&str[i]<='9')
{
sum=sum*10+str[i]-'0';
i++;
}
i--;
tmp.x=sum;
tmp.count=0;
ss.push(tmp);
}
else if(str[i]=='('||str[i]=='+')
{
oper.push(str[i]);
}
else if(str[i]==',')
{
while(!oper.empty()&&oper.top()=='+')
{
tmp1=ss.top();
ss.pop();
tmp2=ss.top();
ss.pop();
tmp1.x+=tmp2.x;
tmp1.count+=tmp2.count+1;
ss.push(tmp1);
oper.pop();
}
}
else if(str[i]==')')
{
while(!oper.empty()&&oper.top()=='+')
{
tmp1=ss.top();
ss.pop();
tmp2=ss.top();
ss.pop();
tmp1.x+=tmp2.x;
tmp1.count+=tmp2.count+1;
ss.push(tmp1);
oper.pop();
}
oper.pop();//弹出 )
tmp2=ss.top();
ss.pop();
tmp1=ss.top();
ss.pop();
if(tmp1.x>tmp2.x)//tmp1 保留最大的
tmp1.count=tmp1.count*2+tmp2.count;
else
{
tmp1.x=tmp2.x;
tmp1.count=tmp1.count+tmp2.count*2;
}
ss.push(tmp1);
}
}
while(!oper.empty()&&oper.top()=='+')//完之后,还可能存在操作符没运算,
//但只可能是‘+’
{
tmp1=ss.top();
ss.pop();
tmp2=ss.top();
ss.pop();
tmp1.x+=tmp2.x;
tmp1.count+=tmp2.count+1;
ss.push(tmp1);
oper.pop();
}
printf("%d %d\n",ss.top().x,ss.top().count);
ss.pop();
}
return 0;
}
HDU 3350 #define is unsafe 栈的模拟题
原创
©著作权归作者所有:来自51CTO博客作者我想有个名字的原创作品,请联系作者获取转载授权,否则将追究法律责任
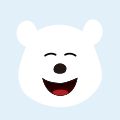
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
一场关于栈的面试----最小栈的实现
一场关于栈的面试----最小栈的实现
最小值 出栈 入栈 -
【Docker技术栈】
Docker 技术栈
Docker -
HDU 1302 The Snail(模拟题)
Problem DescriptionA snail is at the
HDU 1302 The Snail 模拟题 #include -
HDU 1008 Elevator(模拟题)
ElevatorTime Limit: 2000/1000 MS (Java/Others) Memory Li
HDU 1008 Elevator 模拟题 #include -
HDU 1006 Tick and Tick( 模拟题)
Tick and TickTime
HDU 1006 Tick and Tick #include 初始化