#include <stdio.h> #include <stdlib.h> #include <assert.h> #define MAX 1000 char getline(char line[], int limit)//读写 { int ch = 0; int i = 0; while (((ch = getchar()) != '\n') && (ch != EOF) && (--limit)) { line[i++] = ch; } if (ch == '\n') line[i++] = ch; line[i] = '\0'; return i; } char* my_strstr(char * line, char *p) { assert(line); assert(p); //int len1 = strlen(line); //int len2 = strlen(p); //if (len1 < len2) //return NULL; /*while (*line) { int i = 0; while (1) { if (p[i] == '\0') return line; if (p[i] != line[i]) break; i++; } line++; } return NULL;*/ int i = 0; int j = 0; while (line[i]) { if (line[i] == p[j]) { i++; j++; } else { i = i - j + 1; j = 0; } if (p[j] == '\0') break; } } int main() { char line[MAX]; char *p = "ould"; getline(line,MAX); my_strstr(line, p); while (getline(line, MAX)>0) { if (my_strstr(line, p) != NULL) { printf("%s", line); } } system("pause"); return 0; }
输入中包含特定模式或字符串的各行打印出来
原创
©著作权归作者所有:来自51CTO博客作者numo的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:杨氏矩阵
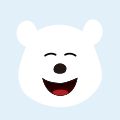
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
java检测字符串是否包含数字和字母
java检测字符串是否包含数字和字母的方法。
git 字符串 System java -
java中字符串拼接的多种方式
java中字符串拼接的多种方式
java 字符串拼接 -
python输入打印出来 python打印出none
1.问题描述 在使用python打印输出验证结果是否符合预期时,有时候会多出一个None。 程序示例:def get_my_info(): print('my name
python输入打印出来 python 开发语言 调用函数