//#include<iostream> //#include<string> //using namespace std; // //class Botany //{ //public: // Botany(const string& name="") // :_name(name) // { // s_Count++; // } // Botany(const Botany& b) // :_name(b._name) // { // s_Count++; // } // Botany& operator=(const Botany& b) // { // if (this != &b) // { // _name = b._name; // } // return *this; // } // ~Botany() // { // --s_Count; // cout << "~Botany()" << endl; // } // virtual void Display() // { // cout << "name"<<_name << endl; // } //protected: // string _name; // static int s_Count; //}; //int Botany::s_Count = 0; // //class Tree:virtual public Botany //{ //public: // Tree(const string& s, int hight, int age) // :Botany(s) // , _hight(hight) // , _age(age) // { // // } // Tree(Tree& t) // :Botany(t._name) // , _hight(t._hight) // , _age(t._age) // { // // } // Tree& operator=(const Tree& t) // { // if (this != &t) // { // _name = t._name; // _hight = t._hight; // _age = t._age; // } // return *this; // } // ~Tree() // { // cout << "~Tree()" << endl; // } // virtual void Display() // { // Botany::Display(); // cout << "hight:" << _hight << endl; // cout << "age:" << _age << endl; // } //protected: // int _hight; // int _age; // //}; //class Flower :virtual public Botany //{ //public: // Flower(const string& name, const string& color) // :Botany(name) // , _color(color) // { // // } // Flower(Flower& f) // :Botany(f._name) // , _color(f._color) // { // // } // Flower& operator=(const Flower& f) // { // if (this != &f) // { // _name = f._name; // _color = f._color; // } // return *this; // } // ~Flower() // { // cout << "~Flower()" << endl; // } // virtual void Display() // { // Botany::Display(); // cout << "color" << _color << endl; // } //protected: // string _color; //}; //class MicheliaAlba:public Tree, public Flower //{ //public: // MicheliaAlba(const string& name, int hight, int age,const string& color,const string& kind) // :Botany(name) // ,Tree(name, hight, age) // , Flower(name, color) // , _kind(kind) // { // // } // MicheliaAlba(const MicheliaAlba& m) // :Botany(m._name) // , Tree(m._name, m._hight,m._age) // , Flower(m._name, m._color) // , _kind(m._kind) // { // // } // MicheliaAlba& operator=(const MicheliaAlba& m) // { // if (this != &m) // { // _name = m._name; // _hight = m._hight; // _age = m._age; // _color = m._color; // _kind = m._kind; // // } // return *this; // } // ~MicheliaAlba() // { // cout << "~MicheliaAlba()" << endl; // } // virtual void Display() // { // Tree::Display(); // Flower::Display(); // cout << "kind" << _kind << endl; // } //protected: // string _kind; //}; //void Test1() //{ // MicheliaAlba m("白兰花",11,2,"红", "小"); // m.Display(); // // Botany b("草"); // b.Display(); // // Tree pine("松树", 15, 27); // pine.Display(); // // Flower f("玫瑰花", "红"); // f.Display(); // // //} //void Test2() //{ // Botany b1("草"); // b1.Display(); // // MicheliaAlba m1("白兰花", 11, 2, "红", "小"); // m1.Display(); // // MicheliaAlba m2("白兰花",13, 4, "白", "大"); // m2.Display(); // // Botany* b = &b1; // b->Display(); // // b=(Botany*)&m1; // b->Display();//多态 // //} //void Test3() //{ // Botany b1("草"); // b1.Display(); // // MicheliaAlba m1("白兰花", 11, 2, "红", "小"); // m1.Display(); // // b1 = m1; // b1.Display();//以草输出:白兰花 //} //int main() //{ // Test3(); // system("pause"); // return 0; //} //
植物类(多态和继承)
原创
©著作权归作者所有:来自51CTO博客作者小止1995的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:带头尾节点的双向链表
下一篇:打印虚表
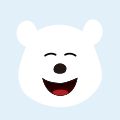
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
python多态继承
python继承
构造函数 多重继承 Python -
python 继承和多态
在已有类的基础上创建新类,这其中的一种做法就是让一个类从
python 父类 子类 抽象类 -
java 动态的继承不同的类 java动物类继承和多态
&n
java 动态的继承不同的类 c/c++ java 父类 子类