编写一个脚本。菜单为:
1、创建用户
2、创建目录
3、创建文件
4、修改目录文件
5、修改文件权限
6、删除目录或者文件
7、退出
#!/bin/bash
#write by lijun
#Date 2014-07-15
#======================================================
#function check_error
#======================================================
function ch_error(){
if [ $? -eq 0 ]
then
echo "succeed!"
else
echo "failed...."
fi
}
#=====================================================
#1.function creat_users
#======================================================
function c_users(){
echo "Please input user's name"
read name1
useradd $name1
ch_error
echo "return main"
sleep 2
main
}
#======================================================
#2.function creat_directory
#======================================================
function c_dir(){
echo "Please input the path like:[/xx/xx/d_name]"
read path1
mkdir -p $path1
ch_error
echo "return main"
sleep 2
main
}
#=====================================================
#3.function creat_file
#====================================================
function c_file(){
echo "Please input the path like:[/xx/xx/f_name]"
read path2
touch $path2
ch_error
echo "return main"
sleep 2
main
}
#=====================================================
#4.funtion change_directory_name
#=====================================================
function change_dir_name(){
echo "Please input the path like:[/xx/xx/d_name] and the new name **the same path except new name**"
read path3 path4
mv $path3 $path4
ch_error
echo "return main"
sleep 2
main
}
#=====================================================
#5.function chmod_directory
#=====================================================
function chmod_dir(){
echo "Please input the path like:[/xx/xx/d_name] and the permission like:[755]"
read path5 perm
chmod $perm $path3
ch_error
echo "return main"
sleep 2
main
}
#=====================================================
#6.function delete_directory_or_file
#=====================================================
function delete_d_or_f(){
echo "Please input the path like :[xx/xx/directory_name or file_name]"
read path6
rm -rf $path6
ch_error
echo "return main"
sleep 2
main
}
#=============================================================================
main()
{
clear
echo "=============菜单============="
echo " "
echo "1.创建用户"
echo " "
echo "2.创建目录"
echo " "
echo "3.创建文件"
echo " "
echo "4.修改目录文件"
echo " "
echo "5.修改目录权限"
echo " "
echo "6.删除目录或者文件"
echo " "
echo "7.退出"
echo ""
read -p "请输入要选择的功能的序列号(1-7):" a
case $a in
1)
clear
c_users;;
2)
clear
c_dir;;
3)
clear
c_file;;
4)
clear
change_dir_name;;
5)
clear
change_dir;;
6)
clear
delete_d_or_f;;
7)
exit;;
*)
echo "You input the wrong chioce!!";;
esac
}
main
创建删除用户,目录等脚本
原创
©著作权归作者所有:来自51CTO博客作者浅眸回川的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:shell安装samba服务
下一篇:mysql数据库备份脚本
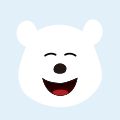
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
WHMCS批量删除有一定特征的用户的脚本
WHMCS如果遭遇恶意注册,那么你可以通过本文中的代码来进行账户的清理
php 上传 API WHMCS -
Linux创建用户、删除用户、切换用户等操作详解
一直在玩liunx系统,但是却一直没静下心来看下增删用户、用户组、用户目录等操作,今天有时间一起看下这些操作,大家有想学习的可以看下去也。
linux 服务器 linux用户管理 用户名 配置文件 -
shell脚本创建/删除Oracle用户、表空间
#!/bin/bash<<INFOAUTHOR:运维@小兵DATE:2021-09-13DESCRIBE:如创建/删除用户、表空间、授权SYSTEM:Cent
oracle 创建/删除Oracle用户 sql 表空间 bash -
用户批量删除脚本
考核内容test[]echo-efor循环思路用户不为root时不可执行删除命令输入为空时提示输入为不存在时提示删除用户成立时删除不成立时用户不存在编辑文件echo-e字体颜色设置,可查询字体颜色表普通用户时文件为空时文件不存在时查看删除列表的批量用户成立时不成立时
linux用户批量删除脚本 shell用户批量删除脚本; test[] echo -e for循环