#!/bin/bash
#
#删除账户在账户管理中相对复杂,至少包含四个步骤:
#① 、获取正确的待删除账户名;
#② 、杀死正在系统上运行的属于该账户的进程;
#③ 、确认系统中属于该账户的所有文件;
#④ 、删除该用户账户
function get_answer {
#
unset ANSWER
ASK_COUNT=0
#
while [ -z "$ANSWER" ]
do
ASK_COUNT=$[ $ASK_COUNT + 1 ]
case $ASK_COUNT in
2)
echo "请输入正确的用户名!"
;;
3)
echo "最后一次,请输入正确的用户名!"
;;
4) echo
echo "用户名不正确"
echo "退出程序!!!"
exit
;;
esac
#
echo
if [ -n "$LINE2" ]
then
echo $LINE1
echo -e $LINE2 " \c"
else
echo -e $LINE1 " \c"
fi
#
read -t 30 ANSWER
done
#
unset LINE1
unset LINE2
#
}
#End of get_answer function
#-------------------------------------------------------------------------------------------------------
function process_answer {
#
case $ANSWER in
y|Y|YES|yes|Yes|yEs|yeS|YEs|yES)
;;
*)
echo
echo $EXIT_LINE1
echo $EXIT_LINE2
echo
exit
;;
esac
#
unset EXIT_LINE1
unset EXIT_LINE2
#
}
#End of process_answer function
#-------------------------------------------------------------------------------------------------------
echo "Step #1 - Determine User Account name to Delete!!"
echo
LINE1="Please enter the username of the user "
LINE2="account you wish to delete from system:"
get_answer
USER_ACCOUNT=$ANSWER
# 检查用户输入是否是正确的用户账户
#
LINE1="Is $USER_ACCOUNT the user account(输入的用户账户) "
LINE2="you wish to delete from the system? [y|n]"
get_answer
#
#
#调用process_answer函数
#
# if user answer anything but "yes",exit script
#
EXIT_LINE1="Because the account,$USER_ACCOUNT,is not "
EXIT_LINE2="The one you wish to delete,we are leaving the script..."
process_answer
##检查用户输入账户是否存在
USER_ACCOUNT_RECORD=$(cat /etc/passwd |grep -w $USER_ACCOUNT)
#
if [ $? -eq 1 ]
#如果返回值为1,即$USER_COUNT不存在,退出脚本
then
echo
echo "Account,$USER_ACCOUNT,not found."
echo "Leaving the script..."
echo
exit
fi
#
echo
echo "I found this record:"
echo $USER_ACCOUNT_RECORD
#
LINE1="Is this the correct User Account?[y|n]"
get answer
#调用process_answer函数
#
# if user answer anything but "yes",exit script
#
EXIT_LINE1="Because the account,$USER_ACCOUNT,is not "
EXIT_LINE2="The one you wish to delete,we are leaving the script..."
process_answer
#查找用户账户是否存在运行进程
#
echo
echo "Step #2 - Find process on system belonging to user account"
echo
#
ps -u $USER_ACCOUNT >/dev/null
#
case $? in
1) #账户无运行进程
echo "该账户无运行中进程。"
;;
0) #账户存在运行中进程
#脚本询问用户是否需要kill掉进程
#
echo "$USER_ACCOUNT has the following processes running:"
echo
ps -u $USER_ACCOUNT
#
LINE1="Would you like me kill the process(es)? [y|n]"
get answer
#
case $ANSWER in
y|Y|YES|yes|Yes|yEs|yeS|YEs|yES)
echo
echo "Killing off process(es)..."
COMMAND_1="ps -u $USER_ACCOUNT --no-heading"
COMMAND_3="xargs -d \\n /usr/bin/sudo /bin/kill -9"
$COMMAND_1 |gawk '{print $1}'| $COMMAND_3
echo
echo "Process(es) Killed."
;;
*)
echo
echo "Will not kill the process(es)"
echo
;;
esac
;;
esac
#-------------------------------------------------------------------
# 创建用户文件报告
echo
echo "Step #3 - Find files on system belonging to user account"
echo
echo "Creating a report of all files owned by $USER_ACCOUNT"
echo
echo "It is recommended that you backup/archive these files."
echo "and then do one of two things:"
echo " 1) Delete the files"
echo " 2) Change the files, ownership to a current user account"
echo
echo "Please wait.this may take a while..."
#
REPORT_DATE=$(date +%y%m%d)
REPORT_FILE=$USER_ACCOUNT"_Files"$REPORT_DATE".rpt"
#
find / -user $USER_ACCOUNT >$REPORT_FILE 2>/dev/null
echo
echo "Report is commplete."
echo "Name of report: $REPORT_FILE"
echo "Location of Report: $(pwd) "
echo
#-------------------------------------------------------------------------------------------
#删除用户账户
echo
echo "Step #4 - Remove user account"
echo
LINE1="Remove $USER_ACCOUNT's account from system? [y|n]"
get_answer
#
#调用process_answer函数
#
# if user answer anything but "yes",exit script
#
EXIT_LINE1="Since you do not wish to remove the user account, "
EXIT_LINE2="$USER_ACCOUNT at this time,exiting the script..."
process_answer
userdel $USER_ACCOUNT
echo
echo "User account,$USER_ACCOUNT,has been removed"
echo
#
exit
删除用户账户(用户管理脚本)
转载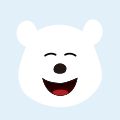
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
mysql中如何删除已经写好的代码
1、安装前须知 版本:mysql-5.7.24 平台:Linux 环境:Centos 72、安装前的必要检查和准备(不
mysql中如何删除已经写好的代码 linux删除mysql mysql bc linux