Java反射实现XML数据拷贝到Bean
原创
©著作权归作者所有:来自51CTO博客作者xiweicheng1987的原创作品,请联系作者获取转载授权,否则将追究法律责任
import java.io.StringReader;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
public class Xml2Beans {
private Xml2Beans() {
}
/**
* xml2BeanList
* @param beanInfoXmlList String
* @param beanCls Class<? extends Object>
* @return List<Object>
* @throws Exception
*/
public static List<Object> xml2BeanList(String beanInfoXmlList,
Class<? extends Object> beanCls) throws Exception {
beanInfoXmlList = "<root>" + beanInfoXmlList + "</root>";
List<Map<String, String>> beanInfoList = xmlParse(beanInfoXmlList);
List<Object> beanList = new ArrayList<Object>();
Method[] methods = beanCls.getDeclaredMethods();
List<Method> methodList = new ArrayList<Method>();
for (Method method : methods) {
if (method.getModifiers() == Modifier.PUBLIC
&& method.getParameterTypes().length == 1
&& method.getName().startsWith("set")) {
methodList.add(method);
}
}
for (Map<String, String> beanInfo : beanInfoList) {
try {
Object obj = getObject(beanInfo, beanCls.newInstance(),
methodList);
beanList.add(obj);
} catch (Exception e) {
e.printStackTrace();
throw e;
}
}
return beanList;
}
//getObject
private static Object getObject(Map<String, String> beanInfo,
Object beanObj, List<Method> methodList) throws Exception {
for (Method method : methodList) {
String methodName = method.getName();
String setPropName = methodName.substring(3).toLowerCase();
if (beanInfo.containsKey(setPropName)) {
try {
String propVal = beanInfo.get(setPropName);
Object param = getParamVal(
method.getParameterTypes()[0].getName(), propVal);
method.invoke(beanObj, param);
} catch (Exception e) {
e.printStackTrace();
throw e;
}
}
}
return beanObj;
}
//getParamVal
private static Object getParamVal(String typeName, String propVal) throws Exception {
if (typeName.equals(Integer.TYPE.getName())
|| typeName.equals(Integer.class.getName())) {
int intVal = 0;
try {
intVal = Integer.parseInt(propVal);
} catch (NumberFormatException e) {
e.printStackTrace();
throw e;
}
return new Integer(intVal);
} else if (typeName.equals(Boolean.TYPE.getName())
|| typeName.equals(Boolean.class.getName())) {
if (propVal.equals("true") || propVal.equals("1")) {
return Boolean.TRUE;
} else {
return Boolean.FALSE;
}
} else if (typeName.equals(BigDecimal.class.getName())) {
int intVal = 0;
try {
intVal = Integer.parseInt(propVal);
} catch (NumberFormatException e) {
e.printStackTrace();
throw e;
}
return new BigDecimal(intVal);
} else {
return propVal;
}
}
//xmlParse
private static List<Map<String, String>> xmlParse(String beansXml) throws Exception {
List<Map<String, String>> beanList = new ArrayList<Map<String, String>>();
try {
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
dbf.setIgnoringComments(true);
dbf.setIgnoringElementContentWhitespace(true);
DocumentBuilder db = dbf.newDocumentBuilder();
StringReader sr = new StringReader(beansXml);
Document document = db.parse(new InputSource(sr));
Element element = document.getDocumentElement();
NodeList beans = element.getChildNodes();
for (int i = 0; i < beans.getLength(); i++) {
Node bean = beans.item(i);
if (bean.getNodeType() == Node.TEXT_NODE) {
continue;
}
NodeList props = bean.getChildNodes();
Map<String, String> propMap = new HashMap<String, String>();
for (int j = 0; j < props.getLength(); j++) {
Node prop = props.item(j);
String propName = prop.getNodeName().toLowerCase();
String propValue = prop.getTextContent();
if (!propMap.containsKey(propName)) {
propMap.put(propName, propValue);
}
}
beanList.add(propMap);
}
} catch (Exception e) {
e.printStackTrace();
throw e;
}
return beanList;
}
}
上一篇:Flex中的正则表达式(二)
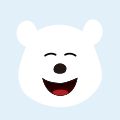
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章