1 package collection;
2
3 import java.util.ArrayList;
4 import java.util.List;
5
6 /**
7 * 自己实现一个ArrayList,帮助理解底层结构
8 * @author Nicholas
9 *
10 */
11 public class SxArrayList{
12 private Object[] elementData;
13 private int size;
14
15 public int size(){
16 return size;
17 }
18 public SxArrayList(){
19 this(10);//默认长度为10
20
21 }
22
23 public SxArrayList(int initialCapacity){
24 if(initialCapacity<0){
25 try {
26 throw new Exception();
27 } catch (Exception e) {
28 e.printStackTrace();
29 }
30 }
31 elementData = new Object[initialCapacity];
32 }
33
34 public boolean isEmpty(){
35 return size==0;
36 }
37
38 public void add(Object obj){
39 //数组扩容和数据的拷贝
40 ensureCapacity();
41 elementData[size++] = obj;
42 }
43
44 public Object get(int index){
45 rangeCheck(index);
46 return elementData[index];
47 }
48
49 public void remove(int index){
50 rangeCheck(index);
51 //删除指定位置的对象
52 int numMoved = size-index-1;
53 if(numMoved>0){
54 System.arraycopy(elementData, index+1, elementData, size, numMoved);;
55 }
56 elementData[--size] = null;
57 }
58
59 public void remove(Object obj){
60 for(int i=0;i<size;i++){
61 if(get(i).equals(obj)){//调用的是equals不是==
62 remove(obj);
63 }
64 }
65 }
66
67 private void rangeCheck(int index){
68 if(size<0||index>=size){
69 try {
70 throw new Exception();
71 } catch (Exception e) {
72 e.printStackTrace();
73 }
74 }
75 }
76
77 public Object set(int index,Object obj){
78 rangeCheck(index);
79 Object oldValue = elementData[index];
80 elementData[index] = obj;
81 return oldValue;
82 }
83
84 public void add(int index,Object obj){
85 rangeCheck(index);
86 ensureCapacity();
87 System.arraycopy(elementData, index, elementData, index+1, size-index);
88 elementData[index] = obj;
89 size++;
90 }
91
92 private void ensureCapacity(){
93 if(size == elementData.length){
94 Object[] newArray = new Object[size*2+1];
95 System.arraycopy(elementData, 0, newArray, 0, elementData.length);
96 elementData = newArray;
97 }
98 }
99
100 public static void main(String[] args) {
101 SxArrayList list = new SxArrayList(3);
102 list.add("3333");
103 list.add("4444");
104 list.add("1111");
105 list.add("2222");
106 System.err.println(list.size);
107 System.err.println(list.get(3));
108 }
109
110 }
102.自己实现ArrayList
原创
©著作权归作者所有:来自51CTO博客作者心流灬灬的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:linux常用的软件更新命令
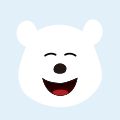
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Acwing -102. 最佳牛围栏(二分)
农夫约翰的农场由N块田地组成,每块地里都有一定数量的牛,其数量不会少于1
ci i++ #include