#include <iostream>
#include <map>
#include <utility>
#include <list>
#include <future>
#include <thread>
using namespace std;
void threadIdTest() { // 获取当前线程id
std::thread::id threadId = std::this_thread::get_id();
std::cout << "threadId: " << threadId << std::endl;
}
int threadFunction(int var) {
std::chrono::milliseconds duration(5000); // 5s.
std::cout << "var: " << var << std::endl;
std::this_thread::sleep_for(duration);
return 50;
}
void threadFunctionTest() {
std::packaged_task<int(int)> task(threadFunction); // 通过 packaged_task 来包装函数 threadFunction;
std::thread currentThread(std::ref(task), 2); // 执行 线程任务 task, 第二个参数为线程入口函数的参数; (另外ref代表引用task对象)
currentThread.join(); // 卡住线程, 直到任务执行结束
std::cout << "task end..." << std::endl;
}
//void threadFunctionFuture(std::future<int> &tmpf)
void threadFunctionFuture(std::shared_future<int>& tempFuture) {
std::cout << "threadFunctionFuture begin, threadId: " << std::this_thread::get_id() << std::endl;
auto result = tempFuture.get(); // 只能get一次, 因为get本身是移动语义
std::cout << "threadFunctionFuture end, result: " << result << std::endl;
}
void threadFunctionFutureTest() {
std::packaged_task<int(int)> threadTask(threadFunction);
// std::future<int> result = threadTask.get_future(); // 应该是相当于把, 把任务上锁同时返回?
// if (result.valid()) {
// std::cout << "1 yes" << std::endl; // bingo
// } else {
// std::cout << "1 no" << std::endl;
// }
//
std::shared_future<int> result_s = std::move(result); // 这句话执行完, result为空;
if (result.valid()) {
std::cout << "2 yes" << std::endl;
} else {
std::cout << "2 no" << std::endl; // bingo
}
//
// std::shared_future<int> result_s = result.share(); // 这句话执行完, result依旧为空;
// if (result.valid()) {
// std::cout << "3 yes" << std::endl;
// } else {
// std::cout << "3 no" << std::endl; // bingo
// }
std::shared_future<int> result_s = threadTask.get_future();
std::thread currentThread(threadFunctionFuture, std::ref(result_s));
currentThread.join(); // 从现象来看, 线程会一直守护在这.
}
int main() {
// threadIdTest();
// threadFunctionTest();
threadFunctionFutureTest();
return 0;
}
量化交易之C++篇 - 多线程(future、share_future)
原创ErwinSmith 博主文章分类:C/C++ ©著作权
©著作权归作者所有:来自51CTO博客作者ErwinSmith的原创作品,请联系作者获取转载授权,否则将追究法律责任
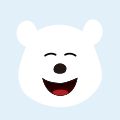
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
量化交易开发之基本语法(三)
本教程则是以量化的情景从零讲解python编程,所以将更适合想学做量化策略的人。
数据 变量名 python -
量化交易开发之函数API(四)
我们讲解一下python中的函数知识
API 数据 python -
量化交易之C++篇 - 谓词
#include <iostream>
#include ios 匿名函数 -
C++之future和promise
future和promise的作用是在不同线程之间传递数据。使用指针也可以完成数据的传递,但是指针非
c++ #include 数据 封装 -
量化交易之C++篇 - 位运算
位运算
c++ 开发语言 算法 ios #include