#include <stdio.h> #include <string.h> #include <stdlib.h> #define MAXSIZE 8 typedef int dataType; typedef struct Stack { dataType date[MAXSIZE]; int top; }Stack; void initStack(Stack *s)//初始化堆栈函数 { s->top=-1; //初始化top指向堆栈的最底部 memset(s->date,0,sizeof(dataType)*MAXSIZE);//将堆栈空间初始化为0(可省略该步骤) } void push(Stack *s,int e) { if (s->top==MAXSIZE-1) { return; } s->top++; s->date[s->top]=e; } void pop(Stack *s,int *e) { if (s->top==-1) { return ; } *e=s->date[s->top];//通过传址的方式获取堆栈中最顶层的数据 s->top--; } int main(void) { Stack myTest; int temp; initStack(&myTest); printf("Push Order:\n"); for (int i=0; i<8; i++) { printf("%d ",i); push(&myTest, i); } printf("\n"); printf("Pop Order:\n"); for (int i=0; i<8; i++) { pop(&myTest, &temp); printf("%d ",temp); } printf("\n"); return 0; }
C数组模拟堆栈
原创
©著作权归作者所有:来自51CTO博客作者NetworkAD的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:C语言中strtok使用方法与原理,以及自实现函数功能
下一篇:螺旋数字程序
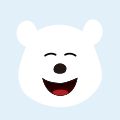
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
CF1523C. Compression and Expansion(堆栈模拟)
题目链接题意:你有一个数字串,开始为空,每轮你可以进行下面两个操作中的一个:从末尾删除若干个数字(可以为 0
数据结构 算法 java python c++ -
使用数组实现堆栈
使用数组实现堆栈
php 职场 数组 休闲