Qt时间轴QTimeLine的基本用法
原创
©著作权归作者所有:来自51CTO博客作者luoyayun361的原创作品,请联系作者获取转载授权,否则将追究法律责任
概述
QTimeLine类提供用于控制动画的时间表,通常用于定期调用插槽来为GUI控件创建动画。简单来说,就是可以通过 QTimeLine 来快速的实现动画效果,其原理就是在指定的时间内发出固定帧率的信号,通过连接该信号去改变目标控件的值,由于时间断帧率高,所以整体看起来就是连续的动画效果。
以上说得可能有些抽象,下面结合实例和用法步骤来看看。
用法
将使用步骤总结为以下几点:
- 1.创建 QTimeLine对象的时候传入时间值,单位是毫秒,该时间就是动画运行的时间;
- 2.设置帧率范围,通过setFrameRange()进行设置,该值表示在规定的时间内将要执行多少帧;
- 3.连接frameChanged()信号,并在槽中对需要实现动画的控件进行赋值,如QProgressBar的setValue().
- 4.调用 start()开始执行时间轴动作。
当 QTimeLine调用 start()后将进入运行状态,并会发出frameChanged()信号,而连接该信号的槽中将会不断的对目标控件进行相应的动作赋值,从而实现动画效果。可以通过调用setUpdateInterval()来指定更新间隔。完成后,QTimeLine进入NotRunning状态,并发出finished().
简单示例
progressBar = new QProgressBar(this);
progressBar->setRange(0, 100);
progressBar->move(100,100);
// Construct a 1-second timeline with a frame range of 0 - 100
QTimeLine *timeLine = new QTimeLine(1000, this);
timeLine->setFrameRange(0, 100);
connect(timeLine, SIGNAL(frameChanged(int)), progressBar, SLOT(setValue(int)));
// Clicking the push button will start the progress bar animation
QPushButton * pushButton = new QPushButton(tr("Start animation"), this);
connect(pushButton, SIGNAL(clicked()), timeLine, SLOT(start()));
当点击按钮后,progressBar 将会在1000ms 内进行100次setValue(),这里的timeLine->setFrameRange(0, 100);表示将执行100帧,也就是说会发出100次frameChanged信号,通过连接该信号去改变progressBar的值。
默认情况下,时间轴从开始到结束运行一次,此时必须再次调用start()才能从头开始重新启动。要进行时间线循环,您可以调用setLoopCount(),并在完成之前传递时间轴应该运行的次数。通过调用setDirection(),方向也可以改变,可以让时间线向后运行。也可以通过调用setPaused()来暂停和取消暂停时间轴的运行。对于交互式控件,提供了setCurrentTime()函数,该函数直接设置时间线的时间位置。
再来看一个示例:
m_pWidget = new QWidget(this);
m_pWidget->resize(50,50);
m_pWidget->setStyleSheet("background-color:red;");
m_pWidget->move(0,100);
m_pWidget->show();
QTimeLine *timeLine = new QTimeLine(1000, this);
timeLine->setFrameRange(0, 200);
connect(timeLine,&QTimeLine::frameChanged,this,[=](int frame){
m_pWidget->move(1*frame,100);
});
connect(timeLine,&QTimeLine::finished,this,[=](){
if(timeLine->direction() == 0){
timeLine->setDirection(QTimeLine::Backward);
}
else{
timeLine->setDirection(QTimeLine::Forward);
}
timeLine->start();
});
QPushButton * pushButton = new QPushButton(tr("Start animation"), this);
connect(pushButton, SIGNAL(clicked()), timeLine, SLOT(start()));
效果:
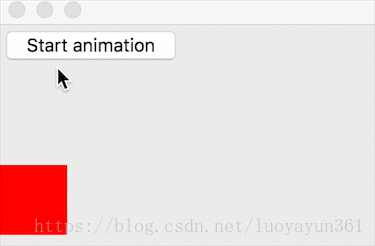
这里通过连接信号finished,当一次动画结束后再调用setDirection来改变动画方向。
更多用法,查看 Qt 帮助文档 http://doc.qt.io/qt-5/qtimeline.html