//--------------------------------------------------------------------------- #pragma hdrstop //--------------------------------------------------------------------------- #pragma argsused #include<string> #include<iostream> using namespace std; struct TTime { int m_year; int m_month; int m_day; int m_time; int m_minite; int m_second; int m_millsecond; TTime() { m_year = 0; m_month = 0; m_day = 0; m_time = 0; m_minite = 0; m_second = 0; m_millsecond = 0; } }; int idayTime[12] = {31,28,31,30,31,30,31,31,30,31,30,31}; double GetSecondTime(const TTime &tSrcTime) { double dDayTime = 0; for(int iIndex = 1; iIndex < tSrcTime.m_month; iIndex ++) { dDayTime = dDayTime + idayTime[iIndex -1]; //闰年多一天 if( ((0 == iIndex % 4)&&(0 != iIndex % 100 ))||( 0 == iIndex % 400 )) { dDayTime = dDayTime + 1; } } //转换到秒 dDayTime = (dDayTime + tSrcTime.m_day) * 24 * 3600; dDayTime = dDayTime + tSrcTime.m_time* 3600 + tSrcTime.m_minite * 60 + tSrcTime.m_second + tSrcTime.m_millsecond / 1000; return dDayTime; } void DiffTime(const TTime &tSrcTime,double &dDiffTime) { double dYearDiff = tSrcTime.m_year - 2000; double dyearTime = 0; double dBetweenTime = 0; //2000年以后 if(dYearDiff > 0) { for(int iIndex = 2000; iIndex < tSrcTime.m_year; iIndex ++) { if((0 == iIndex % 4 && 0 != iIndex % 400) || 0 == iIndex % 400) { dyearTime = dyearTime + 366; } else { dyearTime = dyearTime + 365; } } //转换成秒 dyearTime = dyearTime * 24 * 3600; dBetweenTime = GetSecondTime(tSrcTime); dDiffTime = dyearTime + dBetweenTime; } else { //2000年之前 for(int iIndex = tSrcTime.m_year; iIndex < 2000; iIndex ++) { if((0 == iIndex % 4 && 0 != iIndex % 400) || 0 == iIndex % 400) { dyearTime = dyearTime + 366; } else { dyearTime = dyearTime + 365; } //转换成秒 dyearTime = dyearTime * 24 * 3600; dBetweenTime = GetSecondTime(tSrcTime); dDiffTime = dyearTime - dBetweenTime; } } } int GetDiffTime(string &srcCompare,string &stcCompareTwo,double &iItem,double &dDiff) { TTime m_firstTime; TTime m_secondTime; m_firstTime.m_year = atoi(srcCompare.substr(0,4).c_str()); m_firstTime.m_month = atoi(srcCompare.substr(4,2).c_str()); m_firstTime.m_day = atoi(srcCompare.substr(6,2).c_str()); m_firstTime.m_time = atoi(srcCompare.substr(8,2).c_str()); m_firstTime.m_minite = atoi(srcCompare.substr(10,2).c_str()); m_firstTime.m_second = atoi(srcCompare.substr(12,2).c_str()); m_firstTime.m_millsecond = atoi(srcCompare.substr(14,2).c_str()); double dDiffFirstTime; DiffTime(m_firstTime,dDiffFirstTime); m_secondTime.m_year = atoi(stcCompareTwo.substr(0,4).c_str()); m_secondTime.m_month = atoi(stcCompareTwo.substr(4,2).c_str()); m_secondTime.m_day = atoi(stcCompareTwo.substr(6,2).c_str()); m_secondTime.m_time = atoi(stcCompareTwo.substr(8,2).c_str()); m_secondTime.m_minite = atoi(stcCompareTwo.substr(10,2).c_str()); m_secondTime.m_second = atoi(stcCompareTwo.substr(12,2).c_str()); m_secondTime.m_millsecond = atoi(stcCompareTwo.substr(14,2).c_str()); double dDiffSecondTime; DiffTime(m_secondTime,dDiffSecondTime); double dTimeValue = abs(dDiffFirstTime - dDiffSecondTime); dDiff = dTimeValue / iItem; if(strcmp(srcCompare.c_str(),stcCompareTwo.c_str()) < 0 ) { dDiff = - dDiff; } } int main(int argc, char* argv[]) { string srcCompare= "19991223123000"; string dstCompare= "19991223123000"; double iItem = 3600; double iDiff; GetDiffTime(srcCompare,dstCompare,iItem,iDiff); cout<<"diff time is"<<iDiff<<endl; char c; c = getchar(); return 0; } //---------------------------------------------------------------------------
C++求两个时间的时间差
原创
©著作权归作者所有:来自51CTO博客作者584851044的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:Perl多线程生成一些数据
下一篇:大数相乘
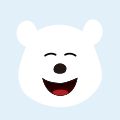
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
C++获得毫秒级的时间差
下面的代码支持Windows和Linux,但是要求编译器必须支持C++11。#include <iostream>#include <chrono>using std
C++ 时间差 毫秒 #include linux -
unix_timestamp计算年份差 unix时间戳 2038
在若日历时间存放在带符号的3 2位整型数中,那么到哪一年它将溢出? 32位有符号整数,其实真正有用的只有31位,所以可以存储的时间是2^31秒,那么是多少年了,可以用如下公式 粗略计算! y = 2^31/(365*
unix_timestamp计算年份差 存储 嵌入式 integer unix