//这是前台的代码
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/";
%><!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<base href="<%=basePath%>">
<title>My JSP 'index.jsp' starting page</title>
<meta http-equiv="pragma" content="no-cache">
<meta http-equiv="cache-control" content="no-cache">
<meta http-equiv="expires" content="0">
<meta http-equiv="keywords" content="keyword1,keyword2,keyword3">
<meta http-equiv="description" content="This is my page">
<!--
<link rel="stylesheet" type="text/css" href="styles.css">
-->
<script type="text/javascript">
/*onload = function (){
//这是判断浏览器是IE还是FireFox
if(navigator.appName == 'Netscape'){
alert('这是火狐浏览器');
}else if(navigator.appName == 'Microsoft Internet Explorer')
{
alert('这是IE浏览器');
}
if(window.XMLHttpRequest)//判断浏览器是否属于Mozilla,Sofari
{
alert("firefox");
}
else if(window.ActiveXObject)//判断浏览器是否属于IE
{
alert('这是IE浏览器');
}
}*/
//这是最传统的ajax
//第一步:创建核心对象
onload = function(){
var xmlRequest = null;
if (window.XMLHttpRequest)
{// code for IE7+, Firefox, Chrome, Opera, Safari
xmlRequest=new XMLHttpRequest();
}
else if(window.ActiveXObject)
{// code for IE6, IE5
xmlRequest=new ActiveXObject("Microsoft.XMLHTTP");
}
if(!xmlRequest)
{
alert("创建核心对象错误!!!");
return fasle;
}
//第二部:打开并发送请求
//1、这是get发送请求的方式,并且还带有参数
/*
第一个参数是请求的方式,最好的大写,第二个参数是请求的路径(这里的路径千万不要用到${pageContext,request.contextPath),也不要
在前面加上'/',例如:/test?p=test&namecaohuan,这也会访问不到路径,第三个参数表示是异步进行还是同步进行,XMLHttpRequest
对象如果要用于AJAX的话其open()方法的第三个参数必须设置为 true,一般我们ajx都是进行异步的操作,所以一般是true
*/
/* xmlRequest.open('GET','test?p=test&name=caohuan',true);
//发送请求,get发送请求并带有参数send中并不带有参数,只有post请求才带有参数
xmlRequest.send();*/
//2、这是post发送请求,并且带有参数
xmlRequest.open('POST','test?p=test',true);
//注意这里post发送请求带有参数的形式,要用post发送请求带有参数,必须要加上下面的第一行的代码,否则后台根本接收不到参数
xmlRequest.setRequestHeader("Content-type","application/x-www-form-urlencoded");
xmlRequest.send("name=caohuan&id=2");
//第三部:接受返回数据
xmlRequest.onreadystatechange = function(){
//判断请求是否发送成功,并且返回数据,这里注意readyState的state的s是大写,我经常犯这种错误
if(xmlRequest.readyState == 4 && xmlRequest.status == 200)
{
//responseText这是接受字符窜的数据,responseXML是接受xml格式的数据
var value = xmlRequest.responseText;
alert(value);
}
}
}
</script>
</head>
<body>
</body>
</html>
//这是后台的代码
package cn.itcast.servlets;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;public class AjaxTestServlet extends HttpServlet {
@Override
protected void service(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
request.setCharacterEncoding("UTF-8");
response.setContentType("text/html;charset=utf-8");
String p = request.getParameter("p");
if("test".equals(p))
{
doTest(request, response);
}
}
protected void doTest(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String name = request.getParameter("name");
String id = request.getParameter("id");
response.getWriter().print(name+"-->" +id);
}
}
ajax传统的方式制作ajax
原创
©著作权归作者所有:来自51CTO博客作者mb64e5cca07d15c的原创作品,请联系作者获取转载授权,否则将追究法律责任
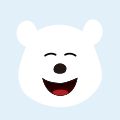
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
ajax 的 get 方式
因为如果使用ajax 的 get 方式提交数据到后台controll
ajax 映射地址 提交数据 -
Ajax请求以及发送Ajax请求的方式
Ajax请求以及发送Ajax请求的方式
封装 jQuery 初始化 -
传统 Ajax 已死,Fetch 永生
传统 Ajax 已死,Fetch 永生
ecmascript ajax -
Ajax请求post方式
Ajax请求post方式得到post.php返回的数据前端代码如下:<!DOCTYPE html><html lang="en"><head> <meta ch
ajax html javascript html5 php -
javascript 创建ajax 创建ajax的多种方式
两种Ajax方法 Ajax是一种用于快速创建动态网页的技术,他通过在后台与服务
javascript 创建ajax 数据 ajax xml