public
class
Test1
{
/*
* 正则表达式:对字符串的常见操作:
* 1.匹配:
* 其实是用的就是string类中的matches(匹配)方法。
* 2.切割
* 其实就是使用的String中的split方法
* 3.替换
* 其实就是 使用的就是String中的replaceAll()方法。。
* 4.获取。
* 1.将正则规则进行对象的封装。
* Pattern p = Pattern.compile("a*b");
* 2.通过正则对象的matcher方法字符串相关联。获取要对字符串操作的匹配对象Matcher
Matcher m = p.matcher("aaaaab");
3.通过Matcher匹配器对象的方法对字符串进行操作。
boolean b = m.matches();
*/
public static void main(String[] args)
{
// TODO Auto-generated method stub
// function1();
// function2();
// function3();
function4();
}
/* 4.获取。
* 1.将正则规则进行对象的封装。
* Pattern p = Pattern.compile("a*b");
* 2.通过正则对象的matcher方法字符串相关联。获取要对字符串操作的匹配对象Matcher
Matcher m = p.matcher("aaaaab");
3.通过Matcher匹配器对象的方法对字符串进行操作。
boolean b = m.matches();*/
public static void function4()
{
String str = "yangchao shi zhengzai haoahao xuexi";
String regex = "\\b[a-z]{3}\\b";//\b 单词边界
//1.将正则封装成对象。
Pattern p = Pattern.compile(regex);
//2.通过正则对象获取匹配器对象。
Matcher m = p.matcher(str);
//通过Matcher匹配器对象的方法对字符串进行操作。
while(m.find())
{
System.out.println(m.group());
}
}
/* 3.替换
* 其实就是 使用的就是String中的replaceAll()方法。。*/
public static void function3()
{
String str = "yangchaoeeeeeeeezhangsanrrrrrrlisi";
String regex = "(.)\\1+";
str = str.replaceAll(regex, "#");//yangchao#zhangsan#lisi
System.out.println(str);
String tel = "18629233301";
tel = tel.replaceAll("(\\d{3})\\d{4}(\\d{4})", "$1****$2");//186****3301
System.out.println(tel);
}
/* * 2.切割
* 其实就是使用的String中的split方法 */
public static void function2()
{
//切割(用空格切割)
String str = "yangchao liuyuan zhangsan lixi";
//定义切割规则
String regex = " +";
String[] names = str.split(regex);
for(String name: names)
{
System.out.println(name);
}
}
/* 1.匹配:
* 其实是用的就是string类中的matches(匹配)方法。*/
public static void function1()
{
//匹配手机号 是否正确。
String str = "18629233301";
//定义规则
String regex = "1[358][0-9]{9}";
regex = "1[358]\\d{9}";
//开始进行判断
boolean b = str.matches(regex);
System.out.println(str+" "+b);
}
}
----------------------------------------------------------------------------------------------------------------------------------------
对邮箱地址的验证。。。。。。
public static void function3()
{
//邮箱地址的验证:
String mail = "yangchao@sina.com";
String regex = "[a-zA-Z0-9]+@[a-zA-Z0-9]+(\\.[a-zA-Z]{1,3})+";
boolean b = mail.matches(regex);
System.out.println(mail+ " "+ b);
}
将ip 进行排序
public static void function2()
{
/*
* ip排序
* 192.23.44.30 127.0.0.1 3.3.3.3.3 103.23.33.44
*/
String ip = "192.23.44.30 127.0.0.1 3.3.3.3 103.23.33.44";
//1.为了让切割够可以按照字符串顺序比较,只要让ip的每一段的位数相同。
//开始不0 每一段都补上2个0.。。。
ip = ip.replaceAll("(\\d+)", "00$1");
System.out.println(ip);//00192.0023.0044.0030 00127.000.000.001 003.003.003.003 00103.0023.0033.0044
//每一段保留数据3位
ip = ip.replaceAll("0*(\\d{3})", "$1");
System.out.println(ip);//192.023.044.030 127.000.000.001 003.003.003.003 103.023.033.044
//2.将ip切出
String[] ips = ip.split(" +");
TreeSet<String> set = new TreeSet<String>();
for(String p : ips)
{
System.out.println(p);
set.add(p);
}
System.out.println("-----------------------------");
for(String p: set)
{
System.out.println(p.replaceAll("0*(\\d+)", "$1"));
}
}
治疗结巴。。。。。。。。。。。。。。。。。。。。。。。
public static void function1()
{
String str = "我我我我我。。。。叫叫。。。杨杨杨杨杨杨。。。。。。。。超超超超超超。。。。";
//1.将字符串中的。去掉 用替换。
str = str.replaceAll("\\。+", "");
System.out.println(str);
//2.替换叠词
str = str.replaceAll("(.)\\1+", "$1");//.为通配符
System.out.println(str);
}
====================================================================================
/*
* 爬取 网络中的 邮箱地址。
*/
public static List<String> getMail2() throws MalformedURLException, IOException
{
URL url = new URL("http:local:8080/mail.html");
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(url.openStream()));
List<String> list = new ArrayList<String>();
//对读取到的数据进行规则匹配,从中获取符合规则的数据。
String regex = "\\w+@\\w+(\\.\\w+)+";//定义规则: \w 单词字符:[a-zA-Z_0-9]
Pattern p = Pattern.compile(regex);//封装成对象将 规则。。
String line=null;
while((line=bufferedReader.readLine()) != null)
{
Matcher m = p.matcher(line);
while(m.find())
{
//将符合的数据 存储到 集合中。
list.add(m.group());
}
}
return list;
}
/*
* 爬取 文件 中的 邮箱地址。。
*/
public static List<String> getMail() throws FileNotFoundException, IOException
{
//读取源文件
BufferedReader bufferedReader = new BufferedReader(new FileReader("C:\\Users\\Administrator\\Desktop\\黑马\\mail.txt"));
List<String> list = new ArrayList<String>();
//对读取到的数据进行规则匹配,从中获取符合规则的数据。
String regex = "\\w+@\\w+(\\.\\w+)+";//定义规则: \w 单词字符:[a-zA-Z_0-9]
Pattern p = Pattern.compile(regex);//封装成对象将 规则。。
String line=null;
while((line=bufferedReader.readLine()) != null)
{
Matcher m = p.matcher(line);
while(m.find())
{
//将符合的数据 存储到 集合中。
list.add(m.group());
}
}
return list;
}
java pattern 匹配 java匹配字符串match
转载文章标签 java pattern 匹配 Java正则表达式字符串操作 System 字符串 数据 文章分类 Java 后端开发
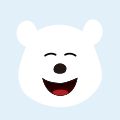
-
Java-长字符串加密
加密:为你的长字符串提供最高级别的保护!!!
加密算法 JAVA -
Java设置JSON字符串参数编码
本文详细介绍了如何在Java中创建JSON字符串以及在Java中设置JSON字符串参数编码的方法。
json 字符串 JSON Java -
java中字符串拼接的多种方式
java中字符串拼接的多种方式
java 字符串拼接 -
java中matcher匹配多个 java匹配字符串match
从今天开始,小编准备用几期的文章给大家介绍一下流传已久的正则表达式,揭开其神秘面纱,小伙伴们准备好了吗? 正则表达式是一个特殊的字符序列,它能帮助你方便的检查一个字符串是否与某种模式匹配。 Python 中的 re 模块,提供 Perl 风格的正则表达式模式,使 Python 语言拥有全部的正则表达式功能,也提供了与这些方法功能完全一致的函数,这些函
java中matcher匹配多个 python re 匹配多行 不是 group by 表达式 不是group by 表达式