java读取文件或是文件流的代码,涵盖了读取jar文件中的文件流,网络文件流等,有些读取方式为了防止编码转换带来的问题,采取了动态byte[]的方式读取,源码如下 :
import java.io.BufferedInputStream;
import java.io.File;
import java.io.BufferedOutputStream;
import java.io.IOException;
import java.io.FileInputStream;
import java.io.FileOutputStream;public class Util ...{
public Util() ...{
}
/** *//**
* 读取源文件内容
* @param filename String 文件路径
* @throws IOException
* @return byte[] 文件内容
*/
public static byte[] readFile(String filename) throws IOException ...{ File file =new File(filename);
if(filename==null || filename.equals(""))
...{
throw new NullPointerException("无效的文件路径");
}
long len = file.length();
byte[] bytes = new byte[(int)len]; BufferedInputStream bufferedInputStream=new BufferedInputStream(new FileInputStream(file));
int r = bufferedInputStream.read( bytes );
if (r != len)
throw new IOException("读取文件不正确");
bufferedInputStream.close(); return bytes;
}
/** *//**
* 将数据写入文件
* @param data byte[]
* @throws IOException
*/
public static void writeFile(byte[] data,String filename) throws IOException ...{
File file =new File(filename);
file.getParentFile().mkdirs();
BufferedOutputStream bufferedOutputStream=new BufferedOutputStream(new FileOutputStream(file));
bufferedOutputStream.write(data);
bufferedOutputStream.close();}
/** *//**
* 从jar文件里读取class
* @param filename String
* @throws IOException
* @return byte[]
*/
public byte[] readFileJar(String filename) throws IOException ...{
BufferedInputStream bufferedInputStream=new BufferedInputStream(getClass().getResource(filename).openStream());
int len=bufferedInputStream.available();
byte[] bytes=new byte[len];
int r=bufferedInputStream.read(bytes);
if(len!=r)
...{
bytes=null;
throw new IOException("读取文件不正确");
}
bufferedInputStream.close();
return bytes;
}/** *//**
* 读取网络流,为了防止中文的问题,在读取过程中没有进行编码转换,而且采取了动态的byte[]的方式获得所有的byte返回
* @param bufferedInputStream BufferedInputStream
* @throws IOException
* @return byte[]
*/
public byte[] readUrlStream(BufferedInputStream bufferedInputStream) throws IOException ...{
byte[] bytes = new byte[100];
byte[] bytecount=null;
int n=0;
int ilength=0;
while((n=bufferedInputStream.read(bytes))>=0)
...{
if(bytecount!=null)
ilength=bytecount.length;
byte[] tempbyte=new byte[ilength+n];
if(bytecount!=null)
...{
System.arraycopy(bytecount,0,tempbyte,0,ilength);
} System.arraycopy(bytes,0,tempbyte,ilength,n);
bytecount=tempbyte; if(n<bytes.length)
break;
}
return bytecount;
}}
1、按字节读取文件内容
2、按字符读取文件内容
3、按行读取文件内容4、随机读取文件内容
public
class
ReadFromFile {
/**
* 以字节为单位读取文件,常用于读二进制文件,如图片、声音、影像等文件。
*/
public
static
void
readFileByBytes(String fileName) {
File file
=
new
File(fileName);
InputStream in
=
null
;
try
{
System.out.println(
"
以字节为单位读取文件内容,一次读一个字节:
"
);
//
一次读一个字节
in
=
new
FileInputStream(file);
int
tempbyte;
while
((tempbyte
=
in.read())
!=
-
1
) {
System.out.write(tempbyte);
}
in.close();
}
catch
(IOException e) {
e.printStackTrace();
return
;
}
try
{
System.out.println(
"
以字节为单位读取文件内容,一次读多个字节:
"
);
//
一次读多个字节
byte
[] tempbytes
=
new
byte
[
100
];
int
byteread
=
0
;
in
=
new
FileInputStream(fileName);
ReadFromFile.showAvailableBytes(in);
//
读入多个字节到字节数组中,byteread为一次读入的字节数
while
((byteread
=
in.read(tempbytes))
!=
-
1
) {
System.out.write(tempbytes,
0
, byteread);
}
}
catch
(Exception e1) {
e1.printStackTrace();
}
finally
{
if
(in
!=
null
) {
try
{
in.close();
}
catch
(IOException e1) {
}
}
}
}
/**
* 以字符为单位读取文件,常用于读文本,数字等类型的文件
*/
public
static
void
readFileByChars(String fileName) {
File file
=
new
File(fileName);
Reader reader
=
null
;
try
{
System.out.println(
"
以字符为单位读取文件内容,一次读一个字节:
"
);
//
一次读一个字符
reader
=
new
InputStreamReader(
new
FileInputStream(file));
int
tempchar;
while
((tempchar
=
reader.read())
!=
-
1
) {
//
对于windows下,\r\n这两个字符在一起时,表示一个换行。
//
但如果这两个字符分开显示时,会换两次行。
//
因此,屏蔽掉\r,或者屏蔽\n。否则,将会多出很多空行。
if
(((
char
) tempchar)
!=
'
\r
'
) {
System.out.print((
char
) tempchar);
}
}
reader.close();
}
catch
(Exception e) {
e.printStackTrace();
}
try
{
System.out.println(
"
以字符为单位读取文件内容,一次读多个字节:
"
);
//
一次读多个字符
char
[] tempchars
=
new
char
[
30
];
int
charread
=
0
;
reader
=
new
InputStreamReader(
new
FileInputStream(fileName));
//
读入多个字符到字符数组中,charread为一次读取字符数
while
((charread
=
reader.read(tempchars))
!=
-
1
) {
//
同样屏蔽掉\r不显示
if
((charread
==
tempchars.length)
&&
(tempchars[tempchars.length
-
1
]
!=
'
\r
'
)) {
System.out.print(tempchars);
}
else
{
for
(
int
i
=
0
; i
<
charread; i
++
) {
if
(tempchars[i]
==
'
\r
'
) {
continue
;
}
else
{
System.out.print(tempchars[i]);
}
}
}
}
}
catch
(Exception e1) {
e1.printStackTrace();
}
finally
{
if
(reader
!=
null
) {
try
{
reader.close();
}
catch
(IOException e1) {
}
}
}
}
/**
* 以行为单位读取文件,常用于读面向行的格式化文件
*/
public
static
void
readFileByLines(String fileName) {
File file
=
new
File(fileName);
BufferedReader reader
=
null
;
try
{
System.out.println(
"
以行为单位读取文件内容,一次读一整行:
"
);
reader
=
new
BufferedReader(
new
FileReader(file));
String tempString
=
null
;
int
line
=
1
;
//
一次读入一行,直到读入null为文件结束
while
((tempString
=
reader.readLine())
!=
null
) {
//
显示行号
System.out.println(
"
line
"
+
line
+
"
:
"
+
tempString);
line
++
;
}
reader.close();
}
catch
(IOException e) {
e.printStackTrace();
}
finally
{
if
(reader
!=
null
) {
try
{
reader.close();
}
catch
(IOException e1) {
}
}
}
}
/**
* 随机读取文件内容
*/
public
static
void
readFileByRandomAccess(String fileName) {
RandomAccessFile randomFile
=
null
;
try
{
System.out.println(
"
随机读取一段文件内容:
"
);
//
打开一个随机访问文件流,按只读方式
randomFile
=
new
RandomAccessFile(fileName,
"
r
"
);
//
文件长度,字节数
long
fileLength
=
randomFile.length();
//
读文件的起始位置
int
beginIndex
=
(fileLength
>
4
)
?
4
:
0
;
//
将读文件的开始位置移到beginIndex位置。
randomFile.seek(beginIndex);
byte
[] bytes
=
new
byte
[
10
];
int
byteread
=
0
;
//
一次读10个字节,如果文件内容不足10个字节,则读剩下的字节。
//
将一次读取的字节数赋给byteread
while
((byteread
=
randomFile.read(bytes))
!=
-
1
) {
System.out.write(bytes,
0
, byteread);
}
}
catch
(IOException e) {
e.printStackTrace();
}
finally
{
if
(randomFile
!=
null
) {
try
{
randomFile.close();
}
catch
(IOException e1) {
}
}
}
}
/**
* 显示输入流中还剩的字节数
*/
private
static
void
showAvailableBytes(InputStream in) {
try
{
System.out.println(
"
当前字节输入流中的字节数为:
"
+
in.available());
}
catch
(IOException e) {
e.printStackTrace();
}
}
public
static
void
main(String[] args) {
String fileName
=
"
C:/temp/newTemp.txt
"
;
ReadFromFile.readFileByBytes(fileName);
ReadFromFile.readFileByChars(fileName);
ReadFromFile.readFileByLines(fileName);
ReadFromFile.readFileByRandomAccess(fileName);
}
}
5、将内容追加到文件尾部
public
class
AppendToFile {
/**
* A方法追加文件:使用RandomAccessFile
*/
public
static
void
appendMethodA(String fileName, String content) {
try
{
//
打开一个随机访问文件流,按读写方式
RandomAccessFile randomFile
=
new
RandomAccessFile(fileName,
"
rw
"
);
//
文件长度,字节数
long
fileLength
=
randomFile.length();
//
将写文件指针移到文件尾。
randomFile.seek(fileLength);
randomFile.writeBytes(content);
randomFile.close();
}
catch
(IOException e) {
e.printStackTrace();
}
}
/**
* B方法追加文件:使用FileWriter
*/
public
static
void
appendMethodB(String fileName, String content) {
try
{
//
打开一个写文件器,构造函数中的第二个参数true表示以追加形式写文件
FileWriter writer
=
new
FileWriter(fileName,
true
);
writer.write(content);
writer.close();
}
catch
(IOException e) {
e.printStackTrace();
}
}
public
static
void
main(String[] args) {
String fileName
=
"
C:/temp/newTemp.txt
"
;
String content
=
"
new append!
"
;
//
按方法A追加文件
AppendToFile.appendMethodA(fileName, content);
AppendToFile.appendMethodA(fileName,
"
append end. \n
"
);
//
显示文件内容
ReadFromFile.readFileByLines(fileName);
//
按方法B追加文件
AppendToFile.appendMethodB(fileName, content);
AppendToFile.appendMethodB(fileName,
"
append end. \n
"
);
//
显示文件内容
ReadFromFile.readFileByLines(fileName);
}
}
引言:
关于java IO流的操作是非常常见的,基本上每个项目都会用到,每次遇到都是去网上找一找就行了,屡试不爽。上次突然一个同事问了我java文件的读取,我一下子就懵了第一反应就是去网上找,虽然也能找到,但自己总感觉不是很踏实,所以今天就抽空看了看java IO流的一些操作,感觉还是很有收获的,顺便总结些资料,方便以后进一步的学习...
IO流的分类:
1、根据流的数据对象来分:
高端流:所有的内存中的流都是高端流,比如:InputStreamReader
低端流:所有的外界设备中的流都是低端流,比如InputStream,OutputStream
如何区分:所有的流对象的后缀中包含Reader或者Writer的都是高端流,反之,则基本上为低端流,不过也有例外,比如PrintStream就是高端流2、根据数据的流向来分:
输出流:是用来写数据的,是由程序(内存)--->外界设备
输入流:是用来读数据的,是由外界设备--->程序(内存)
如何区分:一般来说输入流带有Input,输出流带有Output 3、根据流数据的格式来分:
字节流:处理声音或者图片等二进制的数据的流,比如InputStream
字符流:处理文本数据(如txt文件)的流,比如InputStreamReader
如何区分:可用高低端流来区分,所有的低端流都是字节流,所有的高端流都是字符流4、根据流数据的包装过程来分:
原始流:在实例化流的对象的过程中,不需要传入另外一个流作为自己构造方法的参数的流,称之为原始流。
包装流:在实例化流的对象的过程中,需要传入另外一个流作为自己构造方法发参数的流,称之为包装流。
如何区分:所以的低端流都是原始流,所以的高端流都是包装流IO流对象的继承关系(如下图):
下面来看一些具体的代码例子:
按字节来读取文件
复制代码
public class ReadFromFile {
/**
* 以字节为单位读取文件,常用于读二进制文件,如图片、声音、影像等文件。
*/
public static void readFileByBytes(String fileName) {
File file = new File(fileName);
InputStream in = null;
try {
System.out.println("以字节为单位读取文件内容,一次读一个字节:");
// 一次读一个字节
in = new FileInputStream(file);
int tempbyte;
while ((tempbyte = in.read()) != -1) {
System.out.print(tempbyte);
}
in.close();
} catch (IOException e) {
e.printStackTrace();
return;
}
try {
System.out.println("以字节为单位读取文件内容,一次读多个字节:");
// 一次读多个字节
byte[] tempbytes = new byte[100];
int byteread = 0;
in = new FileInputStream(fileName);
ReadFromFile.showAvailableBytes(in);
// 读入多个字节到字节数组中,byteread为一次读入的字节数
while ((byteread = in.read(tempbytes)) != -1) {
System.out.print(tempbytes, 0, byteread);
}
} catch (Exception e1) {
e1.printStackTrace();
} finally {
if (in != null) {
try {
in.close();
} catch (IOException e1) {
}
}
}
}
按字符来读取文件
复制代码
/**
* 以字符为单位读取文件,常用于读文本,数字等类型的文件
*/
public static void readFileByChars(String fileName) {
File file = new File(fileName);
Reader reader = null;
try {
System.out.println("以字符为单位读取文件内容,一次读一个字符:");
// 一次读一个字符
reader = new InputStreamReader(new FileInputStream(file));
int tempchar;
while ((tempchar = reader.read()) != -1) {
// 对于windows下,\r\n这两个字符在一起时,表示一个换行。
// 但如果这两个字符分开显示时,会换两次行。
// 因此,屏蔽掉\r,或者屏蔽\n。否则,将会多出很多空行。
if (((char) tempchar) != '\r') {
System.out.print((char) tempchar);
}
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
try {
System.out.println("以字符为单位读取文件内容,一次读多个字符:");
// 一次读多个字符
char[] tempchars = new char[30];
int charread = 0;
//由于要以字符来读取,所以需要套上字符流
reader = new InputStreamReader(new FileInputStream(fileName));
// 读入多个字符到字符数组中,charread为一次读取字符数
while ((charread = reader.read(tempchars)) != -1) {
// 同样屏蔽掉\r不显示
if ((charread == tempchars.length)
&& (tempchars[tempchars.length - 1] != '\r')) {
System.out.print(tempchars);
} else {
for (int i = 0; i < charread; i++) {
if (tempchars[i] == '\r') {
continue;
} else {
System.out.print(tempchars[i]);
}
}
}
} } catch (Exception e1) {
e1.printStackTrace();
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e1) {
}
}
}
}
按行来读取文件
复制代码
/**
* 以行为单位读取文件,常用于读面向行的格式化文件
*/
public static void readFileByLines(String fileName) {
File file = new File(fileName);
BufferedReader reader = null;
try {
System.out.println("以行为单位读取文件内容,一次读一整行:");
reader = new BufferedReader(new FileReader(file));
String tempString = null;
int line = 1;
// 一次读入一行,直到读入null为文件结束
while ((tempString = reader.readLine()) != null) {
// 显示行号
System.out.println("line " + line + ": " + tempString);
line++;
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e1) {
}
}
}
}
将一个文件的内容写入另一个文件(按行来写)
复制代码
public class FileTest {
public static void main(String[] args) {
File file=new File("c:\\test.txt");
BufferedReader read=null;
BufferedWriter writer=null;
try {
writer=new BufferedWriter(new FileWriter("c:\\zwm.txt"));
} catch (IOException e1) {
e1.printStackTrace();
}
try {
read=new BufferedReader(new FileReader(file));
String tempString = null;
while((tempString=read.readLine())!=null){
writer.append(tempString);
writer.newLine();//换行
writer.flush();//需要及时清掉流的缓冲区,万一文件过大就有可能无法写入了
}
read.close();
writer.close();
System.out.println("文件写入完成...");
} catch (IOException e) {
e.printStackTrace();
}
}
}
java 接收tcp java 接收文件流
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
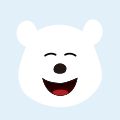
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java 接口请求与文件流处理
JAVA 请求响应中的文件流处理方法
Java 文件流 java