public class Test {
public static void main(String[] args) {
SimpleCalculator s=new SimpleCalculator();
String methord="80*(1+0.5)"; //test
double d=s.evaluate(methord );
System.out.println(d);
}
}
import java.util.Scanner;
import java.util.Stack;
public class SimpleCalculator {
/**
* Evaluate an arithmetic expression, and return the result as a double.
*
* @param input
* the expression to evaluate.
* @return the evaluated result.
*/
public double evaluate(String input) {
initialize();
this.scanner = new Scanner(input);
this.scanner
.useDelimiter("\\s+|(?=[.0-9])(?<![.0-9])|(?![.0-9])(?<=[.0-9])|(?![.0-9])(?<![.0-9])");
Token currentToken = nextToken();
Token t = null;
while (null != currentToken) {
switch (currentToken.getKind()) {
case NUMBER:
// Simply push number tokens onto the evaluation stack.
this.eval.push(currentToken.getValue());
break;
case LPAREN:
// Simply push left parenthesis tokens onto the operator stack.
this.ops.push(currentToken);
break;
case RPAREN:
// Until a left parenthesis pops off the operator stack, keep
// poping operators and execute them.
// If the stack becomes empty without a matching left
// parenthesis,
// the expression must have syntax errors.
for (t = this.ops.pop(); TokenKind.LPAREN != t.getKind(); t = this.ops
.pop()) {
if (ops.empty())
throw new Error("Syntax Error: unmatched parenthesis");
doOperation(t);
}
break;
default:
// For binary arithmetic operators, keep poping operators whose
// binding power
// is less or equal to the current token's and execute them;
// after that push
// the current token onto the operator stack.
if (!ops.empty()) {
for (t = this.ops.pop(); currentToken.getKind()
.getBindingPower() < t.getKind().getBindingPower(); t = this.ops
.pop()) {
doOperation(t);
if (this.ops.empty()) {
t = null;
break;
}
}
}
if (null != t)
ops.push(t);
ops.push(currentToken);
break;
}
// reinitialize
currentToken = nextToken();
}
// execute remaining operators on stack
while (!ops.empty()) {
t = this.ops.pop();
doOperation(t);
}
// the result is on the top of evaluation stack,
// pop it off and return the result.
return this.eval.pop();
}
/*
* Initialize the evaluation and operator stacks.
*/
private void initialize() {
if (null == this.eval)
this.eval = new Stack<Double>();
if (null == this.ops)
this.ops = new Stack<Token>();
this.eval.clear();
this.ops.clear();
}
/*
* Return the next token from the input expression. The token returned will
* be associated with its numeric value, if and only if the token is a
* number.
*/
private Token nextToken() {
Token t = null;
if (this.scanner.hasNextDouble()) {
t = new Token(TokenKind.NUMBER, this.scanner.nextDouble());
} else if (this.scanner.hasNext()) {
String s = this.scanner.next("[-+*/()]");
if ("+".equals(s)) {
t = new Token(TokenKind.ADD);
} else if ("-".equals(s)) {
t = new Token(TokenKind.SUBTRACT);
} else if ("*".equals(s)) {
t = new Token(TokenKind.MULTIPLY);
} else if ("/".equals(s)) {
t = new Token(TokenKind.DIVIDE);
} else if ("(".equals(s)) {
t = new Token(TokenKind.LPAREN);
} else if (")".equals(s)) {
t = new Token(TokenKind.RPAREN);
}
}
return t;
}
/*
* Execute a binary arithmetic operation. Pop the top two values off the
* evaluation stack, do the operation, and then push the result back onto
* the evaluation stack.
*/
private void doOperation(Token t) {
double y = this.eval.pop();
double x = this.eval.pop();
double temp = t.getKind().doOperation(x, y);
this.eval.push(temp);
}
/*
* Tokenizer for the input expression.
*/
private Scanner scanner;
/*
* Evaluation stack.
*/
private Stack<Double> eval;
/*
* Operator stack, for converting infix expression to postfix expression.
*/
private Stack<Token> ops;
public static void main(String[] args) {
if (args.length < 1) {
System.err.println("Usage: java SimpleCalculator <expression>");
System.exit(1);
}
SimpleCalculator calc = new SimpleCalculator();
double result = calc.evaluate(args[0]);
System.out.println(result);
}
}
enum TokenKind {
// operators
ADD(1) {
public double doOperation(double x, double y) {
return x + y;
}
},
SUBTRACT(2) {
public double doOperation(double x, double y) {
return x - y;
}
},
MULTIPLY(3) {
public double doOperation(double x, double y) {
return x * y;
}
},
DIVIDE(4) {
public double doOperation(double x, double y) {
return x / y;
}
},
// punctuation
LPAREN(0), RPAREN(0),
// number
NUMBER(0);
TokenKind(int bindingPower) {
this.bindingPower = bindingPower;
}
public int getBindingPower() {
return this.bindingPower;
}
public double doOperation(double x, double y) {
return Double.NaN; // dummy, operation not supported
}
private int bindingPower;
}
class Token {
public Token(TokenKind kind) {
this(kind, Double.NaN);
}
public Token(TokenKind kind, double value) {
this.kind = kind;
this.value = value;
}
public TokenKind getKind() {
return this.kind;
}
public double getValue() {
return this.value;
}
private TokenKind kind;
private double value;
}
java的字符串表达式执行 java 字符串表达式求值
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
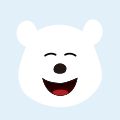
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
java 正则表达式匹配数字去掉前面的字符
java 正则表达式匹配数字去掉前面的字符
字符串 System 正则表达式 -
课时45:表达式_表达式_字符串表达式
这一节,我们从 基础知识、简单实践、小结 三个方面来学习。实践1-内容比较判断。
linux 服务器 运维 字符串 用户名 -
java 字符串求和 java 字符串表达式求值
一、表达式的组成 1、数字 2、运算符:+ - / * ^ % = 3、圆括号 4、变量二、运算符优先级 由高到低分别为:+-(正负号)、^、*/%、+-、= 优先级相等的运算符按照从
java 字符串求和 java exception string token