public static List getListBySql(String sql, Class cls){
List list = new ArrayList();
Connection connection =null;
Statement stmt =null;
ResultSet rs =null;
try {
connection = getConnection();
stmt = connection.createStatement();
rs = stmt.executeQuery(sql);
while (rs.next()) {
Object obj = getObject(rs, cls);
list.add(obj);
}
}catch (Exception e) {
e.printStackTrace();
String sWord = " sql:" + sql;
sWord += " 错误信息:" + e.getLocalizedMessage();
PayMd5Utils.logResult(logpath,sWord);
throw new RuntimeException("#执行出错:"+e.getLocalizedMessage());
}finally{
closeResultSet(rs);
closeStatement(stmt);
closeConnection(connection);
}
return list;
}
private static Object getObject(ResultSet rs, Class cls) throws SQLException, IllegalArgumentException, IllegalAccessException, InstantiationException {
Object object = null;
Field[] fields = cls.getDeclaredFields();
ResultSetMetaData metaData = rs.getMetaData();
int columnCount = metaData.getColumnCount();
for (int i = 1; i <= columnCount; i++) {
String columnName = metaData.getColumnName(i);
Field field = getField(fields, columnName);
if (field != null) {
if (object==null) {
object=cls.newInstance();
}
field.setAccessible(true);
Object value = rs.getObject(field.getName());
setFieldValue(object, value, field);
}
}
return object;
}
private static Field getField(Field[] fields, String columnName) {
for (Field field : fields) {
if (columnName.toUpperCase().equals(field.getName().toUpperCase())) {
return field;
}
}
return null;
}
private static void setFieldValue(Object obj, Object value, Field field)
throws IllegalArgumentException, IllegalAccessException {
if (value == null) {
return;
}
if (field.getType() == Long.class) {
field.set(obj, StringUtil.toLong(value));
} else if (field.getType() == Double.class) {
field.set(obj, StringUtil.toDouble(value));
} else if (field.getType() == Integer.class) {
field.set(obj, StringUtil.toInteger(value));
} else if (field.getType() == Date.class) {
field.set(obj, new Date());
} else {
field.set(obj, StringUtil.toString(value));
}
}
mongdb java封装工具类
转载文章标签 mongdb java封装工具类 java 封装查询sql SQL 数据 sql 文章分类 Java 后端开发
上一篇:linuxrpc用不了怎么办
下一篇:java实习生bi试题
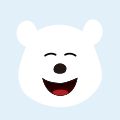
-
封装一个工具类,拒绝重复代码!
封装一个工具类,拒绝重复代码!
List 字段 工具类 -
SwiftyStoreKit 封装工具类
封装意义: struct和class的区别: 成员属性私有化: 优点1,控制成员的读写:设置name可读可写:#include<iostream>
c++ java 算法 Powered by 金山文档 构造函数