package com.sunhang.phonebook;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;public class PhoneBook {
//存放联系人的容器
List<Contacts> list = new ArrayList<Contacts>();
/**
* 增加联系人
*/
public void newContacts() throws ParseException{
try{
Scanner sc = new Scanner(System.in);
System.out.println("输入姓名:");
String name = sc.next();
System.out.println("输入电话:");
String num = sc.next();
System.out.println("输入生日:");
String birth = sc.next();
System.out.println("输入email:");
String email = sc.next();
System.out.println("输入地址:");
String location = sc.next();
System.out.println("输入关系:");
String relation = sc.next();
//将数据保存在联系人对象当中
Contacts c = new Contacts();
c.setName(name);
c.setNum(num);
c.setBirth(birth);
c.setEmail(email);
c.setLocation(location);
c.setRelation(relation);
list.add(c);
System.out.println("联系人保存成功");
}catch(NumberFormatException e){
System.out.println("编号必须为数字");
}
}
/**
* 删除联系人
*/
public void delContacts(){
try{
Scanner sc = new Scanner(System.in);
System.out.println("输入要删除的编号: ");
String sid = sc.next();
int id = Integer.parseInt(sid);
//判断用户是否存在
int index = -1;
for (int i = 0; i < list.size(); i++) {
if (id == list.get(i).getId()) {
index = i;
break;
}
}
if (index == -1) {
System.out.println("输入的联系人编号不存在,请重新输入.");
}else {
//删除集合中的联系人
list.remove(index);
System.out.println("联系人删除成功.");
}
}catch(NumberFormatException e){
System.out.println("编号必须为数字");
}
}
/**
* 查看联系人
*/
public void showContacts(){
Scanner sc = new Scanner(System.in);
System.out.println("输入要查看的联系人编号: ");
String sid = sc.next();
int id = Integer.parseInt(sid);
//判断联系人是否存在
int index = -1;
for (int i = 0; i < list.size(); i++) {
if (id == list.get(i).getId()) {
index = i;
break;
}
}
if (index == -1) {
System.out.println("输入的联系人不存在,请重新输入: ");
}else {
System.out.println("编号: " + list.get(index).getId() +
"姓名: " + list.get(index).getName() +
"电话: " + list.get(index).getNum());
}
}
/**
* 查看全部联系人
*/
public void showContactsAll(){
if (list.isEmpty()) {
System.out.println("没有联系人,请添加");
}else{
for (Contacts i : list) {
System.out.println("编号: " + i.getId() +
"姓名: " + i.getName());
}
}
}
/**
* 查看联系人详细信息
*/
public void showContactsDetils(){
Scanner sc = new Scanner(System.in);
System.out.println("请输入要查看的联系人编号: ");
String sid = sc.next();
int id = Integer.parseInt(sid);
//判断联系人是否存在
int index = -1;
for (int i = 0; i < list.size(); i++) {
if (id == list.get(i).getId()) {
index = i;
break;
}
}
if (index == -1) {
System.out.println("输入的联系人不存在,请重新输入: ");
}else {
System.out.println(list.get(index));
}
}}
package com.sunhang.phonebook;
import java.util.Scanner;
public class CeShiCeng {
public static void main(String[] args) {
PhoneBook pb = new PhoneBook();
System.out.println("-------欢迎使用航哥电话本-------");
while(true){
System.out.println("**************************");
System.out.println("* 请选择功能选项: *");
System.out.println("* 1.新建联系人 *");
System.out.println("* 2.删除联系人 *");
System.out.println("* 3.查看联系人 *");
System.out.println("* 4.查看所有联系人 *");
System.out.println("* 5.查看联系人详细信息 *");
System.out.println("* 0.退出 *");
System.out.println("***************************");
Scanner sc = new Scanner(System.in);
try{
int key = sc.nextInt();
switch (key) {
case 0:
System.out.println("谢谢使用,再见!");
return;
case 1:
pb.newContacts();
break;
case 2:
pb.delContacts();
break;
case 3:
pb.showContacts();
break;
case 4:
pb.showContactsAll();
break;
case 5:
pb.showContactsDetils();
break;
default:
System.out.println("输入的数字有误,请重新输入!");
break;
}
}catch(Exception e){
System.out.println("请输入数字...");
}
}
}
}
package com.sunhang.phonebook;
import java.util.Date;
public class Contacts {
private int id;
private String name;
private String num;
private String birth;
private String location;
private String email;
private String relation;
public int getId() {
return id;
} public void setId(int id) {
this.id = id;
} public String getName() {
return name;
} public void setName(String name) {
this.name = name;
} public String getNum() {
return num;
} public void setNum(String num) {
this.num = num;
} public String getBirth() {
return birth;
} public void setBirth(String birth) {
this.birth = birth;
} public String getLocation() {
return location;
} public void setLocation(String location) {
this.location = location;
} public String getEmail() {
return email;
} public void setEmail(String email) {
this.email = email;
} public String getRelation() {
return relation;
} public void setRelation(String relation) {
this.relation = relation;
} public String toString(){
return "ID: " + id + "姓名: " + name +
"电话: " + num + "生日: " +
"email: " + email + "地址: " + location +
"关系: " + relation;
}
}
java 造数据 固定电话 java电话本
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
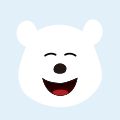
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
电话本
(这个是我们c++作业) 此电话本可完成以下功能:(1) 实现简单电话本功能,用姓名来搜索电话号码;(2) 用户输入姓名,保存 用户输
电话 string iterator file insert