#include <iostream>
using namespace std;
typedef int elemType;
//#definde Maxsize 10
class seqStack{
public :
int maxSize;
int top;
elemType * array;
public :
/*init the stack*/
void initStack(int maxsize){
this->maxSize=maxsize;
this->array=new elemType[maxSize];
this->top=-1;
}
/*constructor*/
seqStack(int maxsize){
this->initStack(maxsize);
}
/*destrucotr*/
~seqStack(){
delete []array;
}
/*is full?*/
bool isStackFull(){
if(this->top==this->maxSize-1)
return true;
else
return false;
}
/*is empty?*/
bool isStackEmpty(){
if(this->top==-1)
return true;
else
return false;
}
/*push the element into the stack,
if the stack is full ,return fasle;
else return true;
*/
bool push(elemType element){
if(this->isStackFull())
return false;
this->top++;
this->array[this->top]=element;
return true;
}
/*
pop the top element from the stack;
if the stack is empty ,return false;
else return true.
*/
bool pop(elemType& topElement){
if(this->isStackEmpty())
return false;
topElement=this->array[this->top];
this->top--;
return true;
}
/*get the current size of the stack */
int getSize(){
return this->top+1;
}
/*get the top element
if the stack is empty ,return false ;
else true;
*/
bool getTop(elemType& topElement){
if(this->isStackEmpty())
return false;
topElement=this->array[this->top];
return true;
}
};
int main()
{
seqStack seqstack(10);
seqstack.push(2);
seqstack.push(3);
seqstack.push(4);
elemType element;
int size;
cout<<"push 2 , 3, 4"<<endl;
size=seqstack.getSize();
cout<<"size is : "<<size<<endl;
seqstack.pop(element);
cout<<"pop the top , top is : "<<element<<endl;
size=seqstack.getSize();
cout<<"size is : "<<size<<endl;
seqstack.getTop(element);
cout<<"get the top is :"<<element<<endl;
cout << "Hello world!" << endl;
return 0;
}
顺序栈的基本操作code_legend
原创
©著作权归作者所有:来自51CTO博客作者legend05070911的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:C 程序可移植性_legend
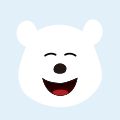
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】C语言实现顺序栈
【数据结构】第三章——栈、队列和数组详细介绍通过C语言实现顺序栈
数据结构 C语言 顺序栈 -
共享栈的基本操作code_legend
/*一个
共享栈 数组 ios #define -
栈之顺序栈基本操作
#include #include #define MaxSize 100//顺序栈的存储结构typedef char ElemType;t
栈 顺序栈 typedef 出栈 Stack -
栈的定义及基本操作实现(顺序栈)
相信大家小时后一定玩过玩具枪吧,在我们装子弹时玩具枪的子弹只能从弹夹的一端进并且从同一端出来,
数据结构 链表 算法 线性表 赋值