package com.lgb.app.socket;
import android.content.Context;
import io.netty.bootstrap.Bootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioSocketChannel;
import io.netty.handler.codec.protobuf.ProtobufDecoder;
import io.netty.handler.codec.protobuf.ProtobufEncoder;
import io.netty.handler.codec.protobuf.ProtobufVarint32FrameDecoder;
import io.netty.handler.codec.protobuf.ProtobufVarint32LengthFieldPrepender;
import io.netty.handler.timeout.IdleStateHandler;
public class LgbClient {
private EventLoopGroup group;
private Context mContext;
public void connect(int port, String host, Context context) {
this.mContext = context;
System.out.println("客户端socket服务正在启动...");
group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
//.option(ChannelOption.SO_BACKLOG, 2048)
.option(ChannelOption.TCP_NODELAY, true)
.option(ChannelOption.SO_KEEPALIVE, true)
.handler(new ChildChannelHandler());
ChannelFuture f = b.connect(host, port).sync();
if (f.isSuccess()) {
System.out.println("客户端socket服务启动成功...");
}
f.channel().closeFuture().sync();
System.out.println("客户端socket链路关闭...");
} catch (Exception e) {
e.printStackTrace();
} finally {
System.out.println("客户端socket shutdownGracefully...");
group.shutdownGracefully();
try {
Thread.sleep(60*1000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
new LgbClient().connect(Constant.PORT, Constant.IP,this.mContext);
}
}
private class ChildChannelHandler extends ChannelInitializer<SocketChannel> {
@Override
protected void initChannel(SocketChannel arg0) throws Exception {
arg0.pipeline().addLast(new ProtobufVarint32FrameDecoder());
arg0.pipeline().addLast(new ProtobufDecoder(LgbMessage.Message.getDefaultInstance()));
arg0.pipeline().addLast(new ProtobufVarint32LengthFieldPrepender());
arg0.pipeline().addLast(new ProtobufEncoder());
arg0.pipeline().addLast("idlehandler", new IdleStateHandler(60, 3, 0));//心跳监测
arg0.pipeline().addLast(new LgbClientHandler(mContext));
}
}
}
Client
原创
©著作权归作者所有:来自51CTO博客作者wx6302e02ec3673的原创作品,请联系作者获取转载授权,否则将追究法律责任
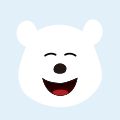
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Linux编译socket.io-client-cpp
Linux socket.io build.
socket.io Linux Build socket.io-client.cpp c++ -
elasticsearch java client中QueryBuilders的geoIntersectionQuery、geoWithinQuery、geoDisjointQuery的解释
解释geo类方法
elasticsearch es java client -
BGP进阶学习之client-to-client
默认情况下BGP会使用Client-to-client,当客户端建立了full-mesh时,就会出现重复路由反射,这是不必要的,可以关系这个属性。
职场 BGP 休闲 client-to-client -
client compiler架构 client-based
C/S又称Client/Server或客户/服务器模式。服务器通常采用高性能的PC、工作站或小型机,并采用大型数据库系统,如Oracle、Sybase、Informix或 SQL Server。客户端需要安装专用的客户端软件。 B/S是Brower/Server的缩写,客户
client compiler架构 sqlserver informix 服务器 sybase