struct free_throws
{
std::string name;
int made;
int attempt;
float percent;
};
void display(const free_throws & ft);
void set_pc(free_throws & ft);
free_throws & accumulate(free_throws & target, const free_throws & source);
int main()
{
//partial initialization - remain members set to 0
free_throws one = { "一",13,14 };
free_throws two = { "二",10,16 };
free_throws three = { "三",7,9 };
free_throws four = { "四",5,9 };
free_throws five = { "五",6,14 };
free_throws team = { "六",0,0 };
//no initialization
free_throws dup;
set_pc(one);
display(one);
accumulate(team, one);
display(team);
display(accumulate(team, two));
accumulate(accumulate(team, three), four);
display(team);
dup = accumulate(team, five);
std::cout << "Displaying team:\n";
display(team);
std::cout << "Displaying dup after assignment:\n";
display(dup);
set_pc(four);
accumulate(dup, five) = four;
std::cout << "Displaying dup after ill-advised assignment:\n";
display(dup);
system("pause");
return 0;
}
void display(const free_throws & ft)
{
using std::cout;
cout << "Name: " << ft.name << std::endl;
cout << " Made: " << ft.made << '\t';
cout << "Attempt: " << ft.attempt << '\t';
cout << "Percent: " << ft.percent << std::endl;
}
void set_pc(free_throws & ft)
{
if (ft.attempt != 0)
ft.percent = 100.0f * float(ft.made) / float(ft.attempt);
else
ft.percent = 0;
}
free_throws & accumulate(free_throws & target, const free_throws & source)
{
target.attempt += source.attempt;
target.made += source.made;
set_pc(target);
return target;
}
一个简单的C++函数引用结构的例子
原创
©著作权归作者所有:来自51CTO博客作者一叶孤舟渡的原创作品,请联系作者获取转载授权,否则将追究法律责任
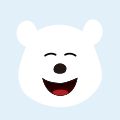
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
C++入门-命名空间、引用、函数重载
C++入门知识,包括命名空间,引用,函数重载等。
命名空间 函数重载 传引用 C++ -
C\C++ Thread-一个简单的多线程例子
多线程thread例子。
UG二次开发 NX二次开发 二次开发 c++ #include -
C++——一个简单的多文件具体例子
(From九天雁翎)Person.h#include #include class Person{
#include const成员函数 构造函数 -
Android中调用C++函数的一个简单Demo
这里我不想多解释什么,对于什么JNI和NDK的相关内容大家自己去百度或谷
android java 文件拷贝 so文件 bundle