TextEdit::TextEdit(QWidget *parent)
: QMainWindow(parent)
{
setToolButtonStyle(Qt::ToolButtonFollowStyle);
setupFileActions();
setupEditActions();
setupTextActions();
{
QMenu *helpMenu = new QMenu(tr("Help"), this);
menuBar()->addMenu(helpMenu);
helpMenu->addAction(tr("About"), this, SLOT(about()));
helpMenu->addAction(tr("About &Qt"), qApp, SLOT(aboutQt()));
}
textEdit = new QTextEdit(this);
connect(textEdit, SIGNAL(currentCharFormatChanged(QTextCharFormat)),
this, SLOT(currentCharFormatChanged(QTextCharFormat)));
connect(textEdit, SIGNAL(cursorPositionChanged()),
this, SLOT(cursorPositionChanged()));
setCentralWidget(textEdit);
textEdit->setFocus();
setCurrentFileName(QString());
QFont textFont("Helvetica");
textFont.setStyleHint(QFont::SansSerif);
textEdit->setFont(textFont);
fontChanged(textEdit->font());
colorChanged(textEdit->textColor());
alignmentChanged(textEdit->alignment());
connect(textEdit->document(), SIGNAL(modificationChanged(bool)),
actionSave, SLOT(setEnabled(bool)));
connect(textEdit->document(), SIGNAL(modificationChanged(bool)),
this, SLOT(setWindowModified(bool)));
connect(textEdit->document(), SIGNAL(undoAvailable(bool)),
actionUndo, SLOT(setEnabled(bool)));
connect(textEdit->document(), SIGNAL(redoAvailable(bool)),
actionRedo, SLOT(setEnabled(bool)));
setWindowModified(textEdit->document()->isModified());
actionSave->setEnabled(textEdit->document()->isModified());
actionUndo->setEnabled(textEdit->document()->isUndoAvailable());
actionRedo->setEnabled(textEdit->document()->isRedoAvailable());
connect(actionUndo, SIGNAL(triggered()), textEdit, SLOT(undo()));
connect(actionRedo, SIGNAL(triggered()), textEdit, SLOT(redo()));
actionCut->setEnabled(false);
actionCopy->setEnabled(false);
connect(actionCut, SIGNAL(triggered()), textEdit, SLOT(cut()));
connect(actionCopy, SIGNAL(triggered()), textEdit, SLOT(copy()));
connect(actionPaste, SIGNAL(triggered()), textEdit, SLOT(paste()));
connect(textEdit, SIGNAL(copyAvailable(bool)), actionCut, SLOT(setEnabled(bool)));
connect(textEdit, SIGNAL(copyAvailable(bool)), actionCopy, SLOT(setEnabled(bool)));
#ifndef QT_NO_CLIPBOARD
connect(QApplication::clipboard(), SIGNAL(dataChanged()), this, SLOT(clipboardDataChanged()));
#endif
QString initialFile = ":/example.html";
const QStringList args = QCoreApplication::arguments();
if (args.count() == 2)
initialFile = args.at(1);
if (!load(initialFile))
fileNew();
}
void TextEdit::closeEvent(QCloseEvent *e)
{
if (maybeSave())
e->accept();
else
e->ignore();
}
void TextEdit::setupFileActions()
{
QToolBar *tb = new QToolBar(this);
tb->setWindowTitle(tr("File Actions"));
addToolBar(tb);
QMenu *menu = new QMenu(tr("&File"), this);
menuBar()->addMenu(menu);
QAction *a;
QIcon newIcon = QIcon::fromTheme("document-new", QIcon(rsrcPath + "/filenew.png"));
a = new QAction( newIcon, tr("&New"), this);
a->setPriority(QAction::LowPriority);
a->setShortcut(QKeySequence::New);
connect(a, SIGNAL(triggered()), this, SLOT(fileNew()));
tb->addAction(a);
menu->addAction(a);
a = new QAction(QIcon::fromTheme("document-open", QIcon(rsrcPath + "/fileopen.png")),
tr("&Open..."), this);
a->setShortcut(QKeySequence::Open);
connect(a, SIGNAL(triggered()), this, SLOT(fileOpen()));
tb->addAction(a);
menu->addAction(a);
menu->addSeparator();
actionSave = a = new QAction(QIcon::fromTheme("document-save", QIcon(rsrcPath + "/filesave.png")),
tr("&Save"), this);
a->setShortcut(QKeySequence::Save);
connect(a, SIGNAL(triggered()), this, SLOT(fileSave()));
a->setEnabled(false);
tb->addAction(a);
menu->addAction(a);
a = new QAction(tr("Save &As..."), this);
a->setPriority(QAction::LowPriority);
connect(a, SIGNAL(triggered()), this, SLOT(fileSaveAs()));
menu->addAction(a);
menu->addSeparator();
}
Qt实现编辑器
精选 原创
©著作权归作者所有:来自51CTO博客作者LazyUpdate的原创作品,请联系作者获取转载授权,否则将追究法律责任
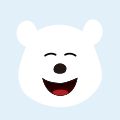
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
2024Typora编辑器最新免费激活方式
2024Typora编辑器最新免费激活方式,最新激活教程
typora markdown typora免费激活教程 -
【Qt】简单QT文本编辑器
00. 目录文章目录00. 目录01. 概述02. 开发环境03. 程序设计04. 软件测试05. 预留06. 附录01. 概述实现基本的文本编辑功能
Qt Qt6 文本编辑器 Qt文本编辑器 QTextEdit -
QT实现代码编辑器
qt实现代码编辑器
Qt QPlainTextEdit 代码编辑器 -
海龟编辑器python 海龟编辑器python编辑器代码
Python被誉为现今人工智能第一语言,适合9岁以上孩子进行编程入门学习。英语是通向全球的语言,编程是通向未来的语言,加德老师与大家一起开启未来世界的大门。课前回顾小朋友们,在上次教学推文中,加德老师带大家进行了Python的海龟作图操作,我们使用了turtle库这个海龟画笔盒绘制了很多令人惊叹的图形。相信小朋友们已经掌握了最基本的海龟作图技能。另外上次的第二节课程,加德老师收到了很多家长发来孩子
海龟编辑器python python turtle代码大全 python代码画皮卡丘 python大作业 python海龟作图不用循环 -
python文件编辑器 python编辑器在哪
Python作为近几年来最为优秀的编程语言之一,受到了很多程序员的追捧,现在我教大家如何打开Python的代码编辑器
python文件编辑器 如何进入python程序代码编辑环境 Python 搜索 输入法