发布订阅设计模式-3- 面向对象设计模式
原创
©著作权归作者所有:来自51CTO博客作者wg_iGBFcBFB的原创作品,请联系作者获取转载授权,否则将追究法律责任
/***
*
* 创建多个事件池
* + 每个事件池是独立的,存放自己订阅的方法
* + 但是也可以具备共同的方法 on/off/fire
* => 面向对象中的类和实例
*
*/
(function(){
class Sub{
// 实例私有属性
pond = [];
// 原型公共方法
on(func){
let pond = this.pond;
!pond.includes(func) ? pond.push(func) : null;
}
off(func){
let pond = this.pond;
pond.forEach((item,index)=>{
if(item === func){
pond[index] = null;
}
})
}
fire(...params){
let pond = this.pond;
for(let i=0;i<pond.length;i++){
let itemFunc = pond[i];
if(typeof itemFunc !== "function"){
// 移除 null
pond.splice(i,1);
i--;
continue;
}
itemFunc(...params);
}
}
}
window.subscribe = function sub(){
return new Sub;
}
})();
//====
// 测试
const fn1 = (x,y)=>{
console.log('fn1',x,y);
}
const fn2 = ()=>{
console.log('fn2');
sub1.off(fn1);
sub1.off(fn2);
}
const fn3 = ()=>{
console.log('fn3');
}
const fn4 = ()=>{
console.log('fn4');
}
const fn5 = (x,y)=>{
console.log('fn5',x,y);
}
let sub1 = subscribe(),
sub2 = subscribe();
sub1.on(fn1)
sub1.on(fn2)
sub1.on(fn3)
sub1.on(fn4)
sub1.on(fn5)
sub2.on(fn1)
sub2.on(fn3)
document.body.onclick = function () {
// 通知事件池的方法执行
sub1.fire(10,20);
sub2.fire(100,200);
}
下一篇:事件-事件对象详解
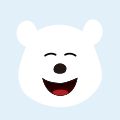
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
设计模式-策略模式
设计模式之策略模式
策略模式 设计模式 -
【设计模式】我这样学习设计模式-发布订阅者模式
虽然你可能还不熟悉 发布-订阅者 模式,但你肯定已经用过它了。发布-订阅者模式在前端领域可谓是无处不在。
前端 设计模式 html 消息队列 数组 -
【设计模式】692- TypeScript 设计模式之发布-订阅模式
观察者模式和发布-订阅模式的核心区别是啥?一起来看看
观察者模式 事件总线 应用程序 -
设计模式--观察者模式(发布订阅模式)
Java工程源码 类图 定义 定义对象间一种一对多的依赖关系,使得每当一个对象改变状态,则所有依赖于它的对象都会得到通知并被自动
java 设计模式 观察者模式 责任链模式 多级 -
设计模式 - 面向对象设计原则
5、依赖倒置原则抽象不要依赖细节,细节应该依赖抽象。换句话说:针对接口编程,而不是针
项目管理 工作 面向对象设计 配置文件 抽象类 -
javascript 设计模式 -- 发布/订阅模式
直接上代码: index.html : .
html jquery 缓存 i++ 数据