js-继承
原创wg_iGBFcBFB 博主文章分类:js高级相关 ©著作权
©著作权归作者所有:来自51CTO博客作者wg_iGBFcBFB的原创作品,请联系作者获取转载授权,否则将追究法律责任
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
// 1 原型继承
// function Parent(){
// this.x = 100
// }
// Parent.prototype.getX = function getX(){
// return this.x;
// }
// function Child(){
// this.y = 200
// }
// Child.prototype = new Parent; // 原型继承
// Child.prototype.getY = function getY(){
// return this.y;
// }
// let C1 = new Child;
// console.log(C1)
// 2 call 继承 (只能继承父类中私有的,不能继承父类中公共的)
// function Parent(){
// this.x = 100
// }
// Parent.prototype.getX = function getX(){
// return this.x;
// }
// function Child(){
// Parent.call(this)
// this.y = 200
// }
// Child.prototype.getY = function getY(){
// return this.y;
// }
// let C1 = new Child;
// console.log(C1)
// 3 寄生组合继承
// function Parent(){
// this.x = 100
// }
// Parent.prototype.getX = function getX(){
// return this.x;
// }
// function Child(){
// Parent.call(this)
// this.y = 200
// }
// // Child.prototype.__proto__ = Parent.prototype;
// Child.prototype = Object.create(Parent.prototype);
// Child.prototype.constructor = Child;
// Child.prototype.getY = function getY(){
// return this.y;
// }
// let C1 = new Child;
// console.log(C1)
/**
* Es6 的类和继承 类似 寄生组合继承
*/
class Parent{
constructor(){
this.x = 100;
}
// Parent.prototype.getX
getX(){
return this.x;
}
}
class Child extends Parent{
constructor(){
super();
this.y = 200;
}
// Person.prototype.getY
getY(){
return this.y;
}
}
let c2 = new Child;
console.log(c2);
</script>
</body>
</html>
上一篇:文档_增删改 * append(content) prepend(content) before(content) after(content) empty() remove()
下一篇:事件绑定与解绑
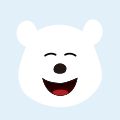
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
python多态继承
python继承
构造函数 多重继承 Python -
JS- this
其实,this的取值,分四种情况。我们来挨个看一下。在此再强调一遍一个非常重要的知识点:在函数中this到底取何值
JS this 函数 构造函数 jquery -
JS-函数
JS-函数
函数 JS 闭包 -
JS-入门
作用:其实也就是JAVAscript漏洞BEEF中hook.js是一个钩子-木马学习路线:正式学习JavaScript输出使用 window.alert() 弹出警告框。使用 document.write()
javascript html5 html 字符串 数组 -
Javascript知识【JS-全局函数对象&JS-事件】
JS-全局函数对象&JS-事件
html 前端 javascript 弹出窗口 键位 -
JS-面向对象
话说,再次看完这个实例后的我,开始怀疑面向对象和JSON的区别。。。并开始怀疑这是面向对象的真实性
Javascript 学习笔记 案例实现 html 面向对象 -
js-惰性函数js
-
JS-语法
1、全等于 2、不等于 !==
html 其他