#include "stdio.h"
void swap(int x,int y)
{
int temp;
temp=x;x=y;y=temp;
}
main()
{
int a=2,b=3;
swap(a,b);
printf("a=%d,b=%d/n",a,b);
}
很简单是吧..........
void GetMemory( char *p )
{
p = (char *) malloc( 100 );
}
void Test( void )
{
char *str = NULL;
GetMemory( str );
strcpy( str, "hello world" );
printf( str );
}
这个呢??
char *GetMemory( void )
{
char p[] = "hello world";
return p;
}
void Test( void )
{
char *str = NULL;
str = GetMemory();
printf( str );
}
这个有错在哪里呢??
void GetMemory( char **p, int num )
{
*p = (char *) malloc( num );
}
void Test( void )
{
char *str = NULL;
GetMemory( &str, 100 );
strcpy( str, "hello" );
printf( str );
}
这个呢???
未判断内存是否申请成功,应加上:
if ( *p == NULL )
{
...//进行申请内存失败处理
}
Test函数中未对malloc的内存进行释放
另外,在free(str)后未置str为空,导致可能变成一个“野”指针,应加上:str = NULL;
swap( int* p1,int* p2 )
{
int *p;
*p = *p1;
*p1 = *p2;
*p2 = *p;
}
在swap函数中,p是一个“野”指针,有可能指向系统区,导致程序运行的崩溃。在VC++中DEBUG运行时提示错误“Access Violation”。该程序应该改为:
swap( int* p1,int* p2 )
{
int p;
p = *p1;
*p1 = *p2;
*p2 = p;
}
传值调用与传值调用
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
上一篇:catch,try,throw
下一篇:树状数组求LIS模板
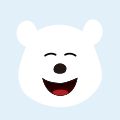
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Android Webview内嵌HTML使用Echarts画图并动态传值
Android Webview内嵌HTML使用Echarts画图并动态传值
Android Webview html echarts 动态传值 -
javascript的拷贝传值和引用传值
一 拷贝传
javascript javascript 那些事儿 -
传值调用和传址调用
传值调用和传址调用的区别
传值调用 传址调用 c语言