typedef char TElemType;
typedef struct BiTree {
TElemType Data;
struct BiTree* LChild, * RChild;
}BiTNode, * BiTree;
bool Search(BiTree T, TElemType key, BiTree f, BiTree& p)
{
if (!T) { p = f; return false; }
else {
if (T->Data == key) { p = T; return true; }
else if (T->Data < key)Search(T->RChild, key, T, p);
else Search(T->LChild, key, T, p);
}
}
bool Insert(BiTree& T, TElemType Item)
{
BiTree p;
if (!Search(T, Item, NULL, p)) {
BiTree NewPTree = (BiTree)malloc(sizeof(BiTNode));
NewPTree->Data = Item;
NewPTree->LChild = NewPTree->RChild = NULL;
if (!p) {
T = NewPTree;
}
else {
if (Item < p->Data)p->LChild = NewPTree;
else p->RChild = NewPTree;
}
}
else return false;
}
bool Delete(BiTree& T, TElemType Item)
{
BiTree p;
Search(T, Item, NULL, p);
if (!p->LChild) {
BiTree q = p;
p = p->RChild;
free(q);
}
else if (!p->RChild) {
BiTree q = p;
p = p->RChild;
free(q);
}
else {
BiTree s = p->LChild, q = p;
while (s->RChild) {
q = s;
s = s->RChild;
}
p->Data = s->Data;
if (q != p)q->RChild = s->LChild;
else q->LChild = s->LChild;
}
}
二叉排序树的查找、插入、删除
转载
include <stdio.h>
include<stdlib.h>
include<stdbool.h>
本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
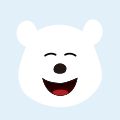
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
二叉排序树的构造,插入,遍历,查找,删除
#include<iostream.h>typedef char dataType; class Node{public: dataType data; Node* lchild; Node* rchild; Node(){ lchild=NULL; rchild=NULL; } Nod
二叉排序树的构造 二叉排序树的插入 二叉排序树的中序遍历 二叉排序树查找结点 二叉排序树删除结点 -
二叉排序树创建,查找,删除
代码public class Main { public static void main(String[] args) {
java 子节点 父节点 二叉排序树 -
二叉排序树的查找与删除
构造一颗二叉排序树的目的,其实并不是为了排序,而是为了提高查找和插入删除关键字的速度。
二叉排序树的查找与删除 结点 二叉排序树 子树