LeetCode刷题(66题、67、69、70、71)
原创
©著作权归作者所有:来自51CTO博客作者心兰相随的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:LeetCode刷题(57、58、59、61、62)
下一篇:函数进阶习题
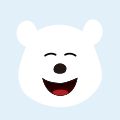
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【leetcode刷题】67 .除数博弈——Java版
dp/数学
leetcode java 算法 动态规划 数学 -
LeetCode刷题——70. 爬楼梯
题目假设你正在爬楼梯。需要 n 阶你才能到达楼顶。每次你可以爬 1 或 2 个台阶。你有多少种不同的方法可以爬到楼顶呢
动态规划 leetcode 70.爬楼梯 递归 斐波那契数列 -
【leetcode刷题】71.数组拆分 I——Java版
排序
java leetcode 算法 排序 数组 -
leetcode刷题六
leetcode刷题六题目叙述已知一个长度为 n 的数组,预先按照升序排列,经由 1 到 n 次 旋转 后,
leetcode 算法 数据结构 数组 升序 -
leetcode刷题七
leetcode刷题七题目叙述给你两个按 非递减顺序 排列的整数数组 nums1 和 nums2,另有两
leetcode 算法 职场和发展 数组 函数返回 -
leetcode刷题八
leetcode刷题八题目叙述峰值元素是指其值严格大于左右相邻值的元素。给你一个整数数组
leetcode 算法 动态规划 数组 时间复杂度