LeetCode刷题(57、58、59、61、62)
原创
©著作权归作者所有:来自51CTO博客作者心兰相随的原创作品,请联系作者获取转载授权,否则将追究法律责任
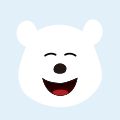
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【LeetCode】39. 组合总和
【LeetCode】39. 组合总和
leetcode 数据结构与算法 C++ -
【LeetCode】705. 设计哈希集合
【LeetCode】705. 设计哈希集合
leetcode 数据结构与算法 C++ -
LeetCode刷题day61
LeetCode刷题day61
leetcode 动态规划 c++ 回文子串 子序列 -
LeetCode刷题day59
LeetCode刷题day59
leetcode c++ 动态规划 算法刷题 数组 -
LeetCode刷题day58
LeetCode刷题day58
java 设计模式 leetcode 数组 初始化 -
LeetCode刷题day57
LeetCode刷题day57
leetcode 算法 动态规划 数组 递归 -
LeetCode刷题——62. 不同路径
题目一个机器人位于一个 m x n 网格的左上角 (起始点在下图中标记为“Start” )。机器人每次只能向下或者向右移动一下 -&g
leetcode 动态规划 62.不同路径 递归 记忆化搜索 -
【leetcode刷题】58.移除元素——Java版
爽
leetcode java 算法 双指针 原力计划 -
【leetcode刷题】62.验证回文串——Java版
双指针
leetcode java 数组 字符串 双指针 -
【leetcode刷题】59.错误的集合——Java版
差点超时
leetcode java 算法 哈希表 原力计划