- import javax.microedition.midlet.*;
- import javax.microedition.lcdui.*;
- /**
- * 该类是应用程序的主类,控制应用程序的生命周期。
- */
- publicclass CalcMIDlet extends MIDlet implements CommandListener {
- //
- private CalcForm calcForm;
- private Command cmdExit = new Command("退出", Command.EXIT, 1);
- publicvoid startApp() {
- Display display = Display.getDisplay(this);
- calcForm = new CalcForm();
- calcForm.addCommand(cmdExit);
- calcForm.setCommandListener(this);
- display.setCurrent(calcForm);
- }
- publicvoid pauseApp() {
- //
- }
- publicvoid destroyApp(boolean unconditional) {
- //
- }
- publicvoid commandAction(Command cmd, Displayable d) {
- if(cmd == cmdExit) {
- notifyDestroyed();
- }
- }
- }
Java代码
- import javax.microedition.lcdui.*;
- /**
- * 该类描述了计算器。
- * 实现了计算器的界面,及加、减、乘、除等计算功能。
- */
- publicclass CalcForm extends Form implements CalcKeyboardListener { //
- private CalcScreen showArea; //计算器的显示区
- private CalcKeyboard ckeyboard; //计算器键盘
- privateboolean hasNewOperand = false; //有新的操作数
- privateboolean numInputing = false;
- privatedouble acc = 0.0; //累加器
- private String operator = ""; //运算符
- privatedouble operand = 0.0; //操作数
- public CalcForm() {
- super("计算器");
- showArea = new CalcScreen(); //创建计算器的显示区对象
- ckeyboard = new CalcKeyboard(4, 5); //创建计算器的键盘
- ckeyboard.setCalcKeyboardListener(this); //
- //布局
- showArea.setLayout(Item.LAYOUT_2|Item.LAYOUT_CENTER|Item.LAYOUT_NEWLINE_AFTER);
- append(showArea);
- append(new Spacer(this.getWidth(), 5));
- ckeyboard.setLayout(Item.LAYOUT_2|Item.LAYOUT_CENTER);
- append(ckeyboard);
- reset();
- }
- //按钮单击事件处理方法
- //如果设备支持触摸屏功能,当用户使用笔在按钮上单击后,
- //注册在键盘上的监视器将调用下面的方法,对单击事件进行处理。
- publicvoid actionPerformmed(CalcKeyboard btn, String symbol) {
- if(symbol == CalcKeyboard.NUM_ZERO || symbol == CalcKeyboard.NUM_ONE || symbol == CalcKeyboard.NUM_TWO ||
- symbol == CalcKeyboard.NUM_THREE ||symbol == CalcKeyboard.NUM_FOUR ||symbol == CalcKeyboard.NUM_FIVE ||
- symbol == CalcKeyboard.NUM_SIX ||symbol == CalcKeyboard.NUM_SEVEN ||symbol == CalcKeyboard.NUM_EIGHT ||
- symbol == CalcKeyboard.NUM_NINE ) {
- //
- inputNum(symbol);
- }
- elseif(symbol == CalcKeyboard.SYMBOL_DOT
- && showArea.getText().indexOf(CalcKeyboard.SYMBOL_DOT) == -1) {
- //
- inputNum(symbol);
- }
- elseif(symbol == CalcKeyboard.BACKSPACE) {
- String text = showArea.getText();
- if(text.length() > 0) {
- text = text.substring(0, text.length()-1);
- showArea.setText(text);
- }
- }
- elseif(symbol == CalcKeyboard.CE) {
- showArea.setText("0.");
- }
- elseif(symbol == CalcKeyboard.C) {
- //计算器归零
- reset();
- }
- elseif(symbol == CalcKeyboard.ADD || symbol == CalcKeyboard.MINUS ||
- symbol == CalcKeyboard.MULT || symbol == CalcKeyboard.DIVIDE ||
- symbol == CalcKeyboard.EQUALS) {
- //
- numInputing = false;
- String s = showArea.getText();
- double d = Double.parseDouble(s);
- jisuan(d, symbol);
- showArea.setText(String.valueOf(acc));
- }
- elseif(symbol == CalcKeyboard.SYMBOL_MINUS) {
- String str = showArea.getText();
- if(str.charAt(0) == '-') {
- showArea.setText(str.substring(1, str.length()));
- }
- else {
- showArea.setText("-" + str);
- }
- }
- }
- privatevoid jisuan(double exp, String oper) {
- if(operator.equals("")) {
- acc = exp;
- operand = exp;
- }
- else {
- if(hasNewOperand) { //新的操作数
- operand = exp;
- if(operator.equals(CalcKeyboard.ADD)) {
- acc += operand;
- }
- elseif(operator.equals(CalcKeyboard.MINUS)) {
- acc -= operand;
- }
- elseif(operator.equals(CalcKeyboard.MULT)) {
- acc *= operand;
- }
- elseif(operator.equals(CalcKeyboard.DIVIDE)) {
- acc /= operand;
- }
- }
- else {
- if(oper.equals(CalcKeyboard.EQUALS)) {
- if(operator.equals(CalcKeyboard.ADD)) {
- acc += operand;
- }
- elseif(operator.equals(CalcKeyboard.MINUS)) {
- acc -= operand;
- }
- elseif(operator.equals(CalcKeyboard.MULT)) {
- acc *= operand;
- }
- elseif(operator.equals(CalcKeyboard.DIVIDE)) {
- acc /= operand;
- }
- if(!oper.equals(CalcKeyboard.EQUALS)) {
- operator = oper;
- }
- }
- }
- }
- if(!oper.equals(CalcKeyboard.EQUALS)) {
- operator = oper;
- }
- hasNewOperand = false;
- }
- privatevoid reset() {
- hasNewOperand = false;
- numInputing = false;
- acc = 0.0;
- operator = "";
- showArea.setText("0.");
- }
- privatevoid inputNum(String str) {
- if(numInputing) {
- showArea.setText(showArea.getText() + str);
- }
- else {
- showArea.setText(str);
- numInputing = true;
- }
- hasNewOperand = true;
- }
- }
Java代码
- import javax.microedition.lcdui.*;
- /**
- * 该类描述了计算器键盘。提供了直观的图形用户界面,该类支持触摸屏功能。
- */
- publicclass CalcKeyboard extends CustomItem {
- publicstaticfinal String BACKSPACE = "<-";
- publicstaticfinal String CE = "CE";
- publicstaticfinal String C = "C";
- publicstaticfinal String SYMBOL_MINUS = "+/-";
- publicstaticfinal String NUM_ZERO = "0";
- publicstaticfinal String NUM_ONE = "1";
- publicstaticfinal String NUM_TWO = "2";
- publicstaticfinal String NUM_THREE = "3";
- publicstaticfinal String NUM_FOUR = "4";
- publicstaticfinal String NUM_FIVE = "5";
- publicstaticfinal String NUM_SIX = "6";
- publicstaticfinal String NUM_SEVEN = "7";
- publicstaticfinal String NUM_EIGHT = "8";
- publicstaticfinal String NUM_NINE = "9";
- publicstaticfinal String SYMBOL_DOT = ".";
- publicstaticfinal String ADD = "+";
- publicstaticfinal String MINUS = "-";
- publicstaticfinal String MULT = "*";
- publicstaticfinal String DIVIDE = "/";
- publicstaticfinal String EQUALS = "=";
- privatestaticfinalint PRESSED = 0;
- privatestaticfinalint RELEASED = 1;
- private CalcKeyboardListener ckListener; //指针动作监视器
- private Font textFont;
- privateint col; //列
- privateint row; //行
- privateint btnWidth; //按键宽
- privateint btnHeight; //按键高
- privateint hSpace = 4; //按键水平间距
- privateint vSpace = 4; //按键垂直间距
- privateint keyState = RELEASED;
- private String[] keyLabel = {
- BACKSPACE, CE, C, SYMBOL_MINUS,
- NUM_SEVEN, NUM_EIGHT, NUM_NINE, DIVIDE,
- NUM_FOUR, NUM_FIVE, NUM_SIX, MULT,
- NUM_ONE, NUM_TWO, NUM_THREE, MINUS,
- NUM_ZERO, SYMBOL_DOT, EQUALS, ADD
- };
- public CalcKeyboard(int col, int row) {
- super(null);
- textFont = Font.getFont(Font.FACE_SYSTEM, Font.STYLE_BOLD, Font.SIZE_LARGE);
- this.col = col;
- this.row = row;
- btnHeight = textFont.getHeight() + 4;
- btnWidth = btnHeight + 10;
- }
- protectedint getMinContentHeight() {
- return row * (btnHeight + vSpace) - vSpace;
- }
- protectedint getMinContentWidth() {
- return col * (btnWidth + hSpace) - hSpace;
- }
- protectedint getPrefContentHeight(int width) {
- return getMinContentHeight();
- }
- protectedint getPrefContentWidth(int height) {
- return getMinContentWidth();
- }
- protectedvoid paint(Graphics g, int w, int h) {
- for(int i=0; i<keyLabel.length; i++) {
- drawButton(g, keyLabel[i], i%col * (btnWidth+hSpace), i/col*(btnHeight+vSpace), btnWidth, btnHeight);
- }
- }
- privatevoid drawButton(Graphics g, String str, int x, int y, int w, int h) {
- g.setColor(160, 160, 255);
- g.drawRect(x, y, w-1, h-1);
- if(keyState == RELEASED) {
- g.setColor(240, 240, 255);
- }
- elseif(keyState == PRESSED) {
- g.setColor(210, 210, 255);
- }
- g.fillRect(x+2, y+2, w-4, h-4);
- g.setColor(0, 0, 0);
- g.setFont(textFont);
- g.drawString(str, x+w/2, y+h, Graphics.BOTTOM|Graphics.HCENTER);
- }
- privateint getIndex(int x, int y) {
- int j = x / (btnWidth+hSpace);
- int i = y / (btnHeight+vSpace);
- return (col*i)+j;
- }
- //指针事件处理方法
- //如果当前设备支持触摸屏功能,当用户使用笔在屏幕上按下时,
- //系统将调用该方法。
- protectedvoid pointerPressed(int x, int y) {
- keyState = PRESSED;
- int ax = x - x % (btnWidth+hSpace);
- int ay = y - y % (btnHeight+vSpace);
- repaint(ax, ay, btnWidth, btnHeight);
- }
- //指针事件处理方法
- //如果当前设备支持触摸屏功能,当用户使用笔在屏幕上按下,然后释放时,
- //系统将调用该方法。
- protectedvoid pointerReleased(int x, int y) {
- keyState = RELEASED;
- int ax = x - x % (btnWidth+hSpace);
- int ay = y - y % (btnHeight+vSpace);
- repaint(ax, ay, btnWidth, btnHeight);
- if(ckListener != null) {
- int index = getIndex(x, y);
- ckListener.actionPerformmed(this, keyLabel[index]);
- }
- }
- //为当前计算器键盘设置监视器
- publicvoid setCalcKeyboardListener(CalcKeyboardListener ckListener) {
- this.ckListener = ckListener;
- }
- }
- /**
- * 该接口描述了计算器键盘的监视器,定义了计算器键盘按钮单击动作
- * 的处理方法。
- */
- publicinterface CalcKeyboardListener {
- //指针单击动作的处理方法。
- //如果设备支持触摸屏功能,当用户使用笔单击屏幕上计算盘键盘上的按钮
- //时,监视该键盘的监视器将回调该方法,处理单击动作。
- //参数ck表示被监视的计算器键盘,symbol表示键盘上的按键。
- publicvoid actionPerformmed(CalcKeyboard ck, String symbol);
- }
Java代码
- import javax.microedition.lcdui.*;
- /**
- * 该类描述了计算器的显示区,用于显示输入的操作数及计算结果。
- */
- publicclass CalcScreen extends CustomItem {
- private String text;
- private Font showFont;
- public CalcScreen() {
- super(null);
- showFont = Font.getFont(Font.FACE_SYSTEM, Font.STYLE_BOLD, Font.SIZE_LARGE);
- text = "";
- }
- protectedint getMinContentHeight() {
- return showFont.getHeight() + 4;
- }
- protectedint getMinContentWidth() {
- return showFont.stringWidth("012345678901234.-") + 4;
- }
- protectedint getPrefContentHeight(int width) {
- return getMinContentHeight();
- }
- protectedint getPrefContentWidth(int height) {
- return 150;
- }
- protectedvoid paint(Graphics g, int w, int h) {
- g.setColor(160, 160, 255);
- g.drawRect(0, 0, w-1, h-1);
- g.setColor(210, 210, 255);
- g.drawRect(2, 2, w-5, h-5);
- g.setColor(0, 0, 0);
- g.setFont(showFont);
- g.drawString(text, w-10, h-3, Graphics.BOTTOM|Graphics.RIGHT);
- }
- publicvoid setText(String text) {
- this.text = text;
- repaint();
- }
- public String getText() {
- return text;
- }
- }
JAVA计算器【源码】
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
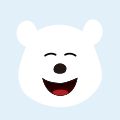
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Java实现一个简单的计算器
Java编写一个简单的计算器。
System 运算符 字符串 -
计算器的实现 源码
Android 计算器的实现
计算器 Java -
Java开源科学计算库 java计算器源码
本计算器仅供交流使用,如有错误,敬请谅解。其中通过自己的理解修改和加入了一些功能
Java开源科学计算库 java 控件 ci