C++编写api一例
; Com.Xp.VCT.def文件
;------------------------------
; Com.Xp.VCT.def : 定义模块参数。
LIBRARY "Com.Xp.VCT"
EXPORTS
;VCT相关操作方法
OpenVCT @1
GetFileHandle @2
ReSetVCT @3
ReadNextLine @4
CloseVCT @5
Test @6
Input_Output @7
//Com.Xp.VCT.cpp 文件
#include "stdafx.h"
#include "resource.h"
#include "dllmain.h"
#include "VCT.h"
#include "IO.h"
#include "stdio.h"
#include <fcntl.h>
#include <stdlib.h>
#include <share.h>
//-------------------------------------------
VCT m_vct;
STDAPI_(bool) OpenVCT(char* FileName)
{
bool rbc=false;
rbc=m_vct.Open(FileName);
return rbc;//
};
STDAPI_(int) GetFileHandle()
{
int fh=m_vct.m_FileHandle;
return fh;
}
STDAPI_(bool) ReSetVCT()
{
bool rbc=false;
rbc=m_vct.ReSet();
return rbc;//
};
STDAPI_(char *) ReadNextLine()
{
char* tt;
tt=m_vct.ReadNextLine();
return tt;//
};
STDAPI_(bool) CloseVCT()
{
bool rbc=false;
rbc=m_vct.Close();
return rbc;//
};
STDAPI_(char*) Test()
{
char* tt="hello world test";
return tt;
};
STDAPI_(char*) Input_Output(char* msg)
{
char* tt=msg;
int fh1 = _open(msg, _O_RDONLY );
//if(_sopen_s(&this->m_FileHandle,FileName,_O_RDONLY, _SH_DENYNO, 0))
if( fh1 != -1 )
{
tt="opened file!";
_close( fh1 );
}
else
{
tt="no open file";
}
return tt;
}
//VCT.h文件
//-------------------------------------
/******************************************
功能:定义VCT头文件的读取方法的类VCT.h
vp:hsg
create date:2009-07-15
*******************************************/
class VCT
{
public:
VCT(void);
~VCT(void);
//打开VCT文件
bool Open(char* FileName);
//重新设置指针到首地址
bool ReSet();
//读取下一行字符串
char* ReadNextLine();
char* ReadNextLine_1();
//查询
//bool Seek();
//关闭VCT文件
bool Close();
//属性
char* m_ErrorMessage;
int m_FileHandle;
};
//VCT.cpp文件
//-------------------------------------
#include "StdAfx.h"
#include <string>
#include <fcntl.h>
#include <stdlib.h>
#include <share.h>
#include "VCT.h"
#include "io.h"
#include "stdio.h"
VCT::VCT(void)
{
}
VCT::~VCT(void)
{
}
//打开VCT文件
bool VCT::Open(char* FileName)
{
bool rbc=false;
int fh1;
fh1 = _open(FileName, _O_RDONLY );
//if(_sopen_s(&this->m_FileHandle,FileName,_O_RDONLY, _SH_DENYNO, 0))
if( fh1 != -1 )
{
//this->m_FileHandle=open(FileName,1);
m_FileHandle =fh1;
rbc=true;
}
else
{
rbc=false;
}
return rbc;
};
bool VCT::ReSet(){
return false;
};
//读取下一行字符串
char* VCT::ReadNextLine()
{
int fh=m_FileHandle;
char* ret="";
char* tt="";
char buffer[1];
unsigned int nbytes = 1, bytesread;
if(!_eof(fh))
{
//getline(
bytesread=_read(fh,buffer,nbytes);
if(bytesread>0)
{
tt=buffer;//new char[0];
}
else
{
tt="";
}
}
return tt;
};
//读取下一行字符串
char* VCT::ReadNextLine_1()
{
int fh=m_FileHandle;
char* ret;
char* tt;
char buffer[1000];
unsigned int nbytes = 1000, bytesread;
if(!_eof(fh))
{
bytesread=_read(fh,buffer,nbytes);
tt=buffer;//new char[0];
}
return tt;
};
//close
//关闭VCT文件
bool VCT::Close()
{
bool rbc=false;
if(m_FileHandle>0)
{
_close(m_FileHandle);
rbc=true;
}
return rbc;
};
.Net C# 测试代码
public class Com_Xp_VCT_API
{
//-------------X.dll----------------------
public const string XDLL = "Com.Xp.VCT.dll";
[DllImport(XDLL, CallingConvention = CallingConvention.StdCall, CharSet = CharSet.Ansi, EntryPoint = "OpenVCT")]
public static extern bool OpenVCT(string FileName);
//GetFileHandle
[DllImport(XDLL, CallingConvention = CallingConvention.StdCall, CharSet = CharSet.Ansi, EntryPoint = "GetFileHandle")]
public static extern long GetFileHandle();
[DllImport(XDLL, CallingConvention = CallingConvention.StdCall, CharSet = CharSet.Ansi, EntryPoint = "ReadNextLine")]
public static extern string ReadNextLine();
[DllImport(XDLL, CallingConvention = CallingConvention.StdCall, CharSet = CharSet.Ansi, EntryPoint = "CloseVCT")]
public static extern bool CloseVCT();
[DllImport(XDLL, CallingConvention = CallingConvention.StdCall, CharSet = CharSet.Ansi, EntryPoint = "ReSetVCT")]
public static extern bool ReSetVCT();
[DllImport(XDLL, CallingConvention = CallingConvention.StdCall, CharSet = CharSet.Ansi, EntryPoint = "Test")]
public static extern string Test();
[DllImport(XDLL, CallingConvention = CallingConvention.StdCall, CharSet = CharSet.Ansi, EntryPoint = "Input_Output")]
public static extern string Input_Output(string msg);
}
private void button2_Click(object sender, EventArgs e)
{
string tt = Com_Xp_VCT_API.Test();
MessageBox.Show(tt.ToString(), "读取值Test");
tt = Com_Xp_VCT_API.Input_Output(Application.StartupPath+"//tt.txt");
MessageBox.Show(tt.ToString(), "读取值Input_Output");
bool rbc = Com_Xp_VCT_API.OpenVCT(Application.StartupPath + "//tt.txt");
MessageBox.Show(rbc.ToString(), "读取值OpenVCT");
long fh=Com_Xp_VCT_API.GetFileHandle();
MessageBox.Show(fh.ToString(), "读取值GetFileHandle");
string line = Com_Xp_VCT_API.ReadNextLine();
while (line != "")
{
this.textBox3.AppendText(line);
line = Com_Xp_VCT_API.ReadNextLine();
}
//MessageBox.Show(line, "读取值ReadNextLine");
Com_Xp_VCT_API.CloseVCT();
}
C++编写api一例
转载本文章为转载内容,我们尊重原作者对文章享有的著作权。如有内容错误或侵权问题,欢迎原作者联系我们进行内容更正或删除文章。
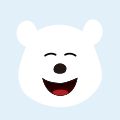
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
API测试用例的编写
API测试用例编写的规范
接口自动化测试 软件测试 自动化测试 API测试