#include <iostream>
#include<malloc.h>
#include<cstdio>
using namespace std;
#define maxsize 50
typedef char elemtype;
typedef struct{
elemtype data[maxsize];
int length;
}sqlist;
void creatlist(sqlist* &l,elemtype a[],int n)
{
l=(sqlist*)malloc(sizeof(sqlist));
for(int i=0;i<n;i++)
{
l->data[i]=a[i];
}
l->length=n;
}
void initlist(sqlist* &l)
{
l=(sqlist*)malloc(sizeof(sqlist));
l->length=0;
}
void destroylist(sqlist* &l)
{
free(l);
}
bool listempty(sqlist *l)
{
return (l->length==0);
}
int listlength(sqlist *l)
{
return l->length;
}
void displist(sqlist* l)
{
for(int i=0;i<l->length;i++)
{
cout<<l->data[i]<<" ";
}
cout<<endl;
}
bool getelem(sqlist* l,int i,elemtype &e)
{
if(i<1||i>>l->length)
return false;
e=l->data[i-1];
return true;
}
int locateelem(sqlist *l,elemtype e)
{
int i=0;
while(i<l->length&&l->data[i]!=e)
{
i++;
}
if(i>=l->length)
return 0;
else return i+1;
}
bool listinsert(sqlist* &l,int i,elemtype e)
{
int j;
if(i<1||i<l->length)
return false;
i--;
for(j=l->length;j>i;j--)
{
l->data[j]=l->data[j-1];
}
l->data[i]=e;
l->length++;
return true;
}
bool listdelete(sqlist* &l,int i,elemtype e)
{
int j;
if(i<1||i>l->length)
return false;
i--;
e=l->data[i];
for(j=i;j<l->length;j++)
{
l->data[j]=l->data[j+1];
}
l->length--;
return true;
}
int main() {
sqlist* l;
sqlist* ll;
elemtype e;
cout<<"顺序表基本运算如下:"<<endl;
cout<<"初始化"<<endl;
initlist(l);
cout<<"依次插入abcd元素"<<endl;
listinsert(l,1,'a');
listinsert(l,2,'b');
listinsert(l,3,'c');
listinsert(l,4,'d');
cout<<"输出顺序表"<<endl;
displist(l);
printf("顺序表长度:%d\n",listlength(l));
printf("顺序表为%s\n",(listempty(l)?"空":"非空"));
getelem(l,3,e);
cout<<"第三个元素为"<<e<<endl;
printf("元素a的位置:%d\n",locateelem(l,'a'));
cout<<"在第四个元素位置插入f元素:"<<endl;
listinsert(l,4,'f');
cout<<"输出顺序表"<<endl;
displist(l);
cout<<"删除l的第三个元素"<<endl;
listdelete(l,3,e);
cout<<"输出顺序表"<<endl;
displist(l);
cout<<"释放顺序表L"<<endl;
destroylist(l);
char shuzu[4]={'1','2','3','4'};
creatlist(ll,shuzu,4);
displist(ll);
return 0;
}
输出结果:
线性表——顺序表操作详解
原创
©著作权归作者所有:来自51CTO博客作者李响Superb的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:实现二叉树各种基本操作的算法
下一篇:malloc
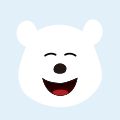
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】详细剖析线性表
【数据结构】第二章——线性表(9)总结了线性表的基本知识点,并对循序表与链表进行了比较
数据结构 C语言 线性表 顺序表 链表 -
线性表之顺序表基本操作
SqHeader.h#ifndef SQHEADER_H_INCLUDED#define SQHEADER_H_INCLU
C++ 线性表 struct 数据结构 顺序表 -
线性表的顺序存储——顺序表
之前我们讲了线性表, 本篇来阐述下线性表的顺序存储——顺序表定义线性表的顺序存储又称为顺序表, 它
数据结构 算法 顺序表 数据 sql -
线性表--顺序表--数组(三)
线性表是最基本、最简单、也是最常用的一种数据结构。线性表中数据元素之间的关系是一对一的关
数据结构 顺序表 线性表 赋值