ConfigParser(py2)模块在python3中修改为configparser该类的作用是使用配置文件生效,
配置文件的格式和windows的INI文件的格式相同
该模块的作用 就是使用模块中的RawConfigParser()、ConfigParser()、 SafeConfigParser()
这三个方法(三者择其一),创建一个对象使用对象的方法对指定的配置文件做增删改查 操作。
配置文件有不同的片段组成和Linux中repo文件中的格式类似:
[section]
name=value
或者
name: value
"#" 和";" 表示注释
[DEFAULT]
ServerAliveInterval = 45
Compression = yes
CompressionLevel = 9
ForwardX11 = yes
[bitbucket.org]
User = hg
[topsecret.server.com]
Port = 50022
ForwardX11 = no
如果想用python生成一个这样的文档怎么做呢?
>>> import configparser
>>> config = configparser.ConfigParser()
>>> config["DEFAULT"] = {'ServerAliveInterval': '45',
>>> 'Compression': 'yes',
>>> 'CompressionLevel': '9'}
>>> config['bitbucket.org']={'User':'lb'}
>>> config['topsecret.server.com'] = {}
>>> topsecret = config['topsecret.server.com']
>>> topsecret['Host Port'] = '50022' # mutates the parser
>>> topsecret['ForwardX11'] = 'no' # same here
>>> config['DEFAULT']['ForwardX11'] = 'yes'
>>> with open('example.ini', 'w') as configfile:
>>> config.write(configfile)
filesnames是一个列表,
需要从文件加载初始值的应用程序应该在调用read()之前使用readfp()加载所需的文件或文件。
查
>>> config.read('example.ini') # 不会输出默认的 DEFAULT 需要特定方法获取
>>> print(config.sections()) #['bitbucket.org', 'topsecret.server.com']
>>> print(config.defaults()) #OrderedDict([('serveraliveinterval', '45'), ('forwardx11', 'yes')])
>>> print('topsecret.server.com' in config) # False 检查是否存在 不存在返回False
>>> print(config['bitbucket.org']['User']) # lb 取值 和字典一样
***************
>>> for key in config:
>>> print(key) # lb DEFAULT bitbucket.org topsecret.server.com
>
>>> for key in config['bitbucket.org']: #DEFAULT 总是跟着
>>> print(key) #lb user serveraliveinterval compression compressionleve forwardx11
****************
>>> print(config.options('bitbucket.org'))
> #['user', 'serveraliveinterval', 'compression', 'compressionlevel', 'forwardx11']
print(config.items('bitbucket.org'))
>#[('serveraliveinterval', '45'), ('compression', 'yes'), ('compressionlevel', '9'), ('forwardx11', 'yes'), ('user', 'lb')]
>>> print(config.get('bitbucket.org','compression')) #yes
>>> sec = config.has_section('topsecret.server.com') #检查是否存在 不存在返回False
>>> print(sec)
删
*****************
>>> config.write(open('i.cfg', "w"))
> #为什么要写这个操作 一个文件内容被定死绝对不会被修改 该操作内容取出是重新生成一个文件
>>> config.write(open('example.ini', "w")) #同名 是先把之前内容清除重新写一遍 不是修改是覆盖
****************
>>> config.remove_option('bitbucket.org','user')
>>> config.write(open('example.ini', "w")) #同名 是先把之前内容清除重新写一遍 不是修改是覆盖
改
**********
>>> sec = config.set('bitbucket.org','22','asss') #一值“模块” 二值“键” 三值“值” 当键不存在 新生成
>>> config.write(open('i.cfg', "w"))
> #为什么要写这个操作 一个文件内容被定死绝对不会被修改 该操作内容取出是重新生成一个文件
*************
pythopn configparser 模块(配置)
原创
©著作权归作者所有:来自51CTO博客作者lb沫的原创作品,请联系作者获取转载授权,否则将追究法律责任
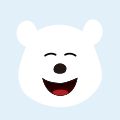
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Windows配置C++的GDAL模块:Visual Studio环境
本文介绍在Visual Studio软件中配置、编译C++ 环境下GDAL库、SQLite环境与PROJ库的详细方法~
C++ GDAL 开发环境 VS Windows -
python模块--Telnetlib模块
telnet模块
ci 用户名 for循环 -
configparser模块简介
configparser模块简介 该模块适用于配置文件的格式与win
配置文件 实例化 查找文件