#include<stdio.h>
#include<malloc.h>
#define MAX_STACK_SIZE 10//静态栈向量大小
#define ERROR 0
#define OK 1
typedef int ElemType;
typedef int Status;
/*
栈的应用:
进制转换
*/
typedef struct sqstack{
ElemType stack_array[MAX_STACK_SIZE];
// int size;
int top;
int bottom;
}SqStack;
//初始化栈
void Init_Stack(SqStack *S){
S->bottom=S->top=0;
printf("\n初始化栈成功!\n");
}
//压栈(元素进栈)
Status push(SqStack *S , ElemType e){
if(S->top >= MAX_STACK_SIZE - 1){
printf("栈满!\n");
return ERROR;//栈满
}
printf("--------");
printf("当前入栈元素:%d\n",e);
// printf("入栈前S->top==%d\n",S->top);
S->top++;//位置自加
// S->size++;
// printf("size==%d",S->size);
printf("入栈后S->top==%d\n",S->top);
S->stack_array[S->top] = e;//元素入栈
return OK;
}
//弹栈(元素出栈)
Status pop(SqStack *S , ElemType e){
if(S->top == 0){
return ERROR;//栈空
}
e = S->stack_array[S->top];//先取
printf("当前应当出栈:%d\n",e);
S->top--;//自减
// S->size--;
// printf("size==%d",S->size);
return OK;
}
//遍历栈
Status StackTravel(SqStack *S){
int e;
int ptr;
ptr = S->top;
while(ptr > S->bottom){
e = S->stack_array[ptr];
ptr--;
printf("%d",e);
}
}
void conversion(int n , int d){
SqStack S;//创建栈
ElemType k;//欲进栈的元素
int temp = n;//保存n
Init_Stack(&S);//初始化栈
// printf("--入栈初始:S->top==%d\n",S.top);
while(n>0){
k = n % d;//取余
push(&S,k);//余数进栈
n = n / d;//结果取整
}
printf("将%d转化成%d进制后为:",temp,d);
StackTravel(&S);//遍历栈
}
int main(){
conversion(511, 2);
conversion(512, 2);
}
利用栈实现进制转换
原创
©著作权归作者所有:来自51CTO博客作者Shiar的原创作品,谢绝转载,否则将追究法律责任
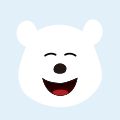
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
一场关于栈的面试----最小栈的实现
一场关于栈的面试----最小栈的实现
最小值 出栈 入栈 -
进制转换 【栈】
//将十进制转换为八进制的
hdu 数据结构 八进制 压栈 ios -
十进制数任意转换2-9进制数--栈的应用
最近看了看数据结构,空间
进制 #include 入栈 -
[数据结构][栈]进制转换
#include <iostream>#include <cstdio>using namespace std;#defin
栈 Stack #include ios