file_ex = open('data.txt', 'w')
如果文件已存在,完全清除现有内容。如果不存在,则创建。
追加到文件,使用 a。
读和写,不清除,用 w+
if 'file_ex' in locals():
file_ex.close()
list相加:
>>> [1,2,3] +[4,5,6]
[1, 2, 3, 4, 5, 6]合情合理,因为str也是list。连个str拼接也是 +。
>>> [1,2,3] +[4,5,6]
[1, 2, 3, 4, 5, 6]但是:
>>> [1,2,3]+"qwer"
Traceback (most recent call last):
File "<pyshell#11>", line 1, in <module>
[1,2,3]+"qwer"
TypeError: can only concatenate list (not "str") to list上面报错。尽管两者都是list,但是列表和字符串无法拼接
但是:
>>>[1,2,3] +[4,'tr',6]+['gg']
[1, 2, 3, 4, 'tr', 6, 'gg']
这样就没有问题
extend修改原先,+ 创建新的。extend 效率高
>>> p='rw'
>>> 'w' in p
True
>>> 'x' in p
False
>>> >>> num=[100,34,678]
>>> len(num)
3
>>> max(num)
678
>>> min(num)
34
>>> >>> num=['22','gg','vb']
>>> ''.join(num)
'22ggvb'
>>> >>> num
['22', 'gg', 'vb']
>>> del num[2]
>>> num
['22', 'gg']
>>> ['to', 'be', 'to'].count('to')
2
>>> x=[[1,2], 1, 1, [2, 1, [1, 2]]]
>>> x.count(1)
2
>>> x.count([1,2])
1
>>>
index 找第一个
>>> ['to', 'be', 'to'].index('to')
0
>>> ['to', 'be', 'to'].index('be')
1
>>>
>>> num = [1,2,3]
>>> num.insert(2, 'four')
>>> num
[1, 2, 'four', 3]
>>> pop默认是最后一个
>>> num
[1, 2, 'four', 3]
>>> num.pop(2)
'four'
>>> num
[1, 2, 3]
>>> num.pop()
3
>>> num
[1, 2]
>>>
remove第一个匹配项
>>> x
['to', 'be', 'to']
>>> x.remove('to')
>>> x
['be', 'to']
>>> remove没有返回值,但pop有
>>> x
['be', 'to']
>>> x.reverse()
>>> x
['to', 'be'] 无返回值
>>> reversed(x)
<list_reverseiterator object at 0x00000000037BCFD0>
>>> list(reversed(x))
['be', 'to']
>>> reversed不返回列表,返回迭代器iterator。要使用list转换成列表。
sort不返回值。sorted 返回。好像加ed 的都返回,不加的不返回。。
x.sort().reverse() 不行,因为sort返回值是None。
>>> x.sort().reverse()
Traceback (most recent call last):
File "<pyshell#53>", line 1, in <module>
x.sort().reverse()
AttributeError: 'NoneType' object has no attribute 'reverse'
>>> sorted(x).reverse()可以
>>> sorted(x).reverse()
>>> x
['be', 'to']
>>> >>> x=['aardvasr','abaddg','asd','dd']
>>> x.sort(key=len)
>>> x
['dd', 'asd', 'abaddg', 'aardvasr']
>>> >>> x.sort(key=len, reverse=False)
>>> x
['dd', 'asd', 'abaddg', 'aardvasr']# 怎么没反过来呢???
>>> x.sort(key=len, reverse=True)
>>> x
['aardvasr', 'abaddg', 'asd', 'dd']#这样可以,默认值是False,很合理!
python 写文件
原创
©著作权归作者所有:来自51CTO博客作者tingtang13的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:UVM:7.7.4 扩展位宽
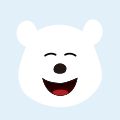
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
python写文件 Python写文件追加
文件的写和追加操作
python写文件 python 文件写入 打开文件 -
python在文件后续写 python with 写文件
python小课堂39 - 用 with 优雅的读写文件前言本篇来介绍一下 Python 中的关键词 with 的小技巧。但是在了解 with 之前,需要先了解一下如何使用 Python 对文件进行读写操作。在了解基本的文件读写操作后,在使用 with 对其进行优雅的操作。写出符合 Pythonic 的代码。对文件的读写操作1. 读文件 在 Python 中,有一个函数 ope
python在文件后续写 python exit()什么意思 python exit()没有定义 python open encoding python open函数 -
python 2.7 写文件 python如何写文件
Python(读写文件)
python 2.7 写文件 1024程序员节 python 打开文件 编码方式