#include <iostream> #include <assert.h> using namespace std; class Date { public: Date(int year = 1900, int month = 1, int day = 1) { _year = year; _month = month; _day = day; if (!CheckDate()) { cout << "输入日期为非法日期" << endl; assert(false); } } Date(const Date& d) { _year = d._year; _month = d._month; _day = d._day; } /*~Date() { cout << "~Date()" << endl; }*/ public: bool CheckDate() { return (_year >= 1900 && _month > 0 && _month<13 && _day>0 && _day <= _GetMonthDay(_year, _month)); } bool operator==(const Date&d) { return (this->_year == d._year && this->_month == d._month && this->_day == d._day); } bool operator!=(const Date&d) { return !(*this == d); } bool operator<(const Date&d) { return (_year < d._year) || (_year == d._year&&_month < d._month) || (_year == d._year&&_month == d._month || _day < d._day); } bool operator<=(const Date&d) { return(*this == d) || (*this < d); } bool operator>=(const Date&d) { return (*this == d) || (*this > d); } bool operator>(const Date&d) { return !(*this <= d); } //日期计算器 Date operator+(int day) { Date tmp(*this); if (day<0) { return (*this - (-day)); //直接减 } tmp._day += day; while (tmp._day>_GetMonthDay(tmp._year, tmp._month)) { tmp._day -= _GetMonthDay(tmp._year, tmp._month); if (tmp._month == 12) { tmp._year++; tmp._month = 1; } else { ++tmp._month; } } return tmp; } int operator-(const Date &d) { int flag = 1; int days = 0; Date max = *this; Date min = d; if (max < min) { swap(max._year, min._year); swap(max._month, min._month); swap(max._day, min._day); flag = -1; } while (min != max) // 直接将小的日期累加到等于大的日期,计算累加天数 { ++min; ++days; } return days*flag; } Date& operator+=(int day) { *this = *this + day; return *this; } Date operator-(int day) { if (day < 0) { return *this + (-day); } Date tmp(*this); tmp._day -= day; while (tmp._day <= 0) { if (tmp._month == 1) //一月特殊 { tmp._year--; tmp._month = 12; } else { --tmp._month; } tmp._day += _GetMonthDay(tmp._year, tmp._month); } } Date& operator-=(int day) { *this = *this -day; return *this; } Date & operator++() { *this += 1; return *this; } Date operator++(int) { Date tmp(*this); *this += 1; return tmp; } Date & operator--() { *this -= 1; return *this; } Date operator--(int) { Date tmp(*this); *this -= 1; return tmp; } void display() { cout << this->_year << "," << this->_month << "," << this->_day << endl; } private: bool _IsLeapYear(int year) { if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) { return true; } else return false; } int _GetMonthDay(int year, int month) //每月天数 { int monthArray[13] = { 0,31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 }; int day = monthArray[month]; if (month == 2 && _IsLeapYear(year)) //闰年二月29天 { day += 1; } return day; } friend ostream & operator << (ostream &out, Date &d); friend istream & operator>>(istream &in, Date &d); private: int _year; int _month; int _day; }; ostream & operator << (ostream &out, Date &d) { out << d._year <<"-"<< d._month<<"-" << d._day; return out; } istream & operator>>(istream &in, Date &d) { in >> d._year >> d._month >> d._day; return in; } void Test1() { Date d1(2016, 12, 25); d1 += 5; d1.display(); d1 += 20; d1.display(); } void Test2() { Date d1(2016, 12, 25); d1 -= 5; d1.display(); d1 -= 20; d1.display(); } void Test3() { Date d1(2016, 12, 25); Date d2(2015, 1, 8); cout << d1 - d2 << endl; cout << d2 - d1 << endl; } void Demo() { Date d1, d2; int days; while (1) { cout << "1.推后x天;" << endl; cout << "2.计算日期相隔天数" << endl; cout << "0.退出查询" << endl; int in; cin >> in; if (in == 1) { cout << "请依次输入一个日期的年、月、日" << endl; cin >> d1; while (!d1.CheckDate()) { cout << "非法日期,请重新输入"<<endl; cin >> d1; } cout << "请输入推后的天数" << endl; cin >> days; cout << d1 + days << endl; } else if (in == 2) { cout << "请依次输入第一个日期的年、月、日" << endl; cin >> d1; while (!d1.CheckDate()) { cout << "非法日期,请重新输入" << endl; cin >> d1; } cout << "请依次输入第二个日期的年、月、日" << endl; cin >> d2; while (!d2.CheckDate()) { cout << "非法日期,请重新输入"; cin >> d2; } cout << "相隔天数为:"<<endl; cout << d1 - d2<<endl; } else if (in == 0) { return; } else { cout << "选择错误,请重新输入序号" << endl; } } }
C++类和对象 日期类运算(万年历)
原创
©著作权归作者所有:来自51CTO博客作者睿蕤芮的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:C++ String 增删查改
下一篇:C++ String 写时拷贝
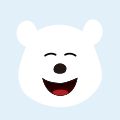
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
万年历(calendar)
nqi.com/2019/01/
日历 万年历 calendar -
java万年历
最近一直在努力补因为ACM而耽误的专业课,o(︶︿︶)o 唉 其实我也很喜欢C语言的
java万年历 万年历 日历 java 事件源