http://blog.csdn.net/wei801004/archive/2006/06/08/780317.aspx #include <iostream> //http://blog.csdn.net/wei801004/archive/2006/06/08/780317.aspx class vehicle class RoadVehicle : public vehicle class AutoVehicle : public RoadVehicle class AirCraft : public vehicle class Helicopter : public AirCraft ///下面就是代理类了,通过这个类来访问不同对象的方法。 class VehicleSurrogate 在这个程序中,存储到容器中的对象都是原来对象的一个副本(即原对象的一个拷贝),一定要复制这些对象吗?答案是否定的,我们可以存储这些对象的句柄,使用句柄同样可以保持原来的对象的多态行为,却是我们减少了不必要的拷贝,何乐而不为呢?
using namespace std;
//http://blog.csdn.net/wei801004/archive/2006/06/09/782290.aspx
#define TEST_1
{
public:
virtual ~vehicle(void) {}
virtual double weight(void) const = 0;
virtual void start(void) = 0;
virtual vehicle* copy(void) const = 0;
};//vehicle是一个抽象类,不可以实例化
{
public:
RoadVehicle(void) {}
~RoadVehicle(void) {}
double weight(void) const
{
cout<<"RoadVehicle weight"<<endl;
return 0;
}
void start(void)
{
cout<<"RoadVehicle start"<<endl;
}
//主要是这里,因为没有RoadVehicle(vehicle)这样的构造函数
vehicle* copy(void) const
{
//调用了默认的拷贝构造函数
return new RoadVehicle(*this);
}
};
{
public:
AutoVehicle(void) {}
~AutoVehicle(void) {}
double weight(void) const
{
cout<<"AutoVehicle weight"<<endl;
return 0;
}
void start(void)
{
cout<<"AutoVehicle start"<<endl;
}
vehicle* copy(void) const
{
//调用了默认的拷贝构造函数
return new AutoVehicle(*this);
}
};
{
public:
AirCraft(void) {}
~AirCraft(void) {}
double weight(void) const
{
cout<<"AirCraft weight"<<endl;
return 0;
}
void start(void)
{
cout<<"AirCraft start"<<endl;
}
vehicle* copy(void) const
{
//调用了默认的拷贝构造函数
return new AirCraft(*this);
}
};
{
public:
Helicopter(void) {}
~Helicopter(void) {}
double weight(void) const
{
cout<<"Helicopter weight"<<endl;
return 0;
}
void start(void)
{
cout<<"Helicopter start"<<endl;
}
vehicle* copy(void) const
{
//调用了默认的拷贝构造函数
return new Helicopter(*this);
}
};
{
public:
VehicleSurrogate(void) : vp(0) {}
//调用对象的copy函数,即构造对象的一个副本,即使是局部变量,也不用怕了。
VehicleSurrogate(const vehicle &v) : vp(v.copy()) {
cout<<endl<<"调用了代理类VehicleSurrogate的Vehicle引用构造函数!"<<endl;
};//构造不同的实例,在程序的编译期间是不知道会是个什么样的对象
~VehicleSurrogate(void)
{
delete vp;
}
//如果对象是代理类,也是可以复制其代理的对象的副本。
VehicleSurrogate(const VehicleSurrogate &v) : vp(v.vp ? v.vp->copy() : 0) {
cout<<endl<<"调用了代理类VehicleSurrogate的复制构造函数!"<<endl;
}
VehicleSurrogate& operator=(const VehicleSurrogate &v)
{
if (this != &v)
{
delete vp;
vp = (v.vp ? v.vp->copy() : 0);
}
return *this;
}
double weight(void)
{
if (vp == 0)
{
cout<<"weight vp is NULL"<<endl;
}
return vp->weight();
}
void start(void)
{
if (vp == 0)
{
cout<<"start vp is NULL"<<endl;
}
return vp->start();
}
private:
vehicle *vp;
};
int main(int argc, char * argv[])
{
VehicleSurrogate parking_lot[2];
Helicopter x;
AirCraft y;
//注意分析这里的执行过程。 调用的是什么函数。
//默认拷贝函数,又是怎么回事儿~。
Helicopter xx = x;
parking_lot[0] = x;
parking_lot[1] = y;
#ifdef TEST_1
VehicleSurrogate x_2;
VehicleSurrogate y_2 = x_2; //调用了复制构造函数 还是 重载的= ? //复制构造函数,所以可以用句柄。不用复制。
#endif
parking_lot[0].weight();
parking_lot[1].start();
return 0;
}
C++沉思录 代理类
精选 转载上一篇: 不知不觉中调用的默认拷贝构造函数
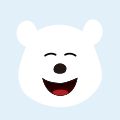
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章