//头文件 #pragma once #define NAME_SIZE 10 #define PASSWD_SIZE 10 #define NAME "农文彤" #define PASSWD "123456" #define TRUE 1 #define CONTINUE(CH) {\ printf("%s", CH); \ getchar();\ } typedef struct player { char _name[NAME_SIZE]; char _passwd[PASSWD_SIZE]; int _total; int _victory; }player_t,*pStrPlayer; /*************************************************/ /*1.函数作用:生成玩家结构体 /*2.函数参数:空 /*3.返回值:pStrPlayer /*************************************************/ pStrPlayer create_player(); /*************************************************/ /*1.函数作用:释放玩家结构体 /*2.函数参数:空 /*3.返回值:空 /*************************************************/ void destroy_player(); /*************************************************/ /*1.函数作用:展示游戏界面 /*2.函数参数:空 /*3.返回值:空 /*************************************************/ void menu(); /*************************************************/ /*1.函数作用:循环控制游戏界面 /*2.函数参数:空 /*3.返回值:空 /*************************************************/ void contrl_menu(); /*************************************************/ /*1.函数作用:玩家选择出拳 /*2.函数参数:空 /*3.返回值:int /*************************************************/ int player_choice(); /*************************************************/ /*1.函数作用:电脑出拳 /*2.函数参数:空 /*3.返回值:int /*************************************************/ int computer_choice(); /*************************************************/ /*1.函数作用:展示每局结果 /*2.函数参数:int com,int pla /*3.返回值:空 /*************************************************/ void show_record(int com,int pla); /*************************************************/ /*1.函数作用:展示游戏总结果 /*2.函数参数:空 /*3.返回值:空 /*************************************************/ void show_all_record(); //函数文件 #include<stdio.h> #include<stdlib.h> #include<string.h> #include<malloc.h> #include<windows.h> #include<time.h> #include"guess_finger.h" pStrPlayer player=NULL; pStrPlayer create_player() { pStrPlayer tmpPlayer = (pStrPlayer)malloc(sizeof(player_t)); if (tmpPlayer == NULL) return NULL; strcpy(tmpPlayer->_name, NAME); strcpy(tmpPlayer->_passwd, PASSWD); tmpPlayer->_total = 0; tmpPlayer->_victory = 0; return tmpPlayer; } void destroy_player() { free(player); } void menu() { printf("******************Welcome To Play Finger_Guess Game!********************\n"); printf(" 1.Rock(石头) 2.Scissors(剪刀) 3. Paper(布) 0.end(结束)\n"); printf("========================================================================\n\n"); } void contrl_menu() { int playerChoice=0; int sub = 0; int computerChoice = 0; player = create_player(); while (TRUE) { system("cls"); menu(); playerChoice = player_choice(); if (playerChoice == 0) { if (player->_total == 0) { destroy_player(); return; } else { show_all_record(); destroy_player(); return; } } computerChoice = computer_choice(); sub = playerChoice - computerChoice; switch (sub) { case -1: case 2: ++(player->_total); ++(player->_victory); printf("Congratulations You Win!\n"); show_record(computerChoice, playerChoice); break; case 0: ++(player->_total); printf("A Dead Heart!\n"); show_record(computerChoice, playerChoice); break; default: ++(player->_total); printf("It's a pity that you lost!\n"); show_record(computerChoice, playerChoice); break; } } } int player_choice() { int input = 0; do { scanf("%d", &input); fflush(stdin); } while (input<0 || input>3); system("cls"); return input; } int computer_choice() { int i = 3; for (; i >= 0; --i) { printf("The computer is thinking! __%d__", i); fflush(stdout); Sleep(1000); system("cls"); } srand((unsigned int)time(NULL)); return rand()%3+1; } void show_record(int com,int pla) { if (1 == com) printf("Computer use Rock!\n"); else if (2==com) printf("Computer use Scissors!\n"); else printf("Computer use Paper!\n"); if (1 == pla) printf("Player use Rock!\n"); else if (2 == pla) printf("player use Scissors!\n"); else printf("player use Paper!\n"); CONTINUE("Press any key to continue ..."); } void show_all_record() { printf("name passwd total v/t \n"); printf("%-10s%-10s%-10d%-10f\n",player->_name,player->_passwd,player->_total,\ (double)(player->_victory)/(player->_total)); CONTINUE("Press any key to continue ..."); } //主函数 #include<stdio.h> #include"guess_finger.h" int main() { contrl_menu(); return 0; }
c 语言 猜拳小游戏
原创
©著作权归作者所有:来自51CTO博客作者霜柒染的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:c 语言 单链表的操作 易考点
下一篇:c++ 复数类
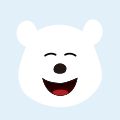
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
c/c++小游戏
入门小游戏-提高思维陆续把以前无聊时写tdl
c/c++ c++ #include 随机数