第五章 条件、循环和其它语句
原创boxvivi007 博主文章分类:python学习笔记 ©著作权
©著作权归作者所有:来自51CTO博客作者boxvivi007的原创作品,请联系作者获取转载授权,否则将追究法律责任
5.1 print 和import的更多信息
5.1.1 使用逗号输出
可以打印多个表达式
>>> print 'age:',42
age: 42
如果需要同时输出文本和变量值,却又不希望使用字符串格式化:
>>> name='test'
>>> salutation='Mr.'
>>> greeting = 'Hello'
>>> print greeting,salutation,name
Hello Mr. test
5.1.2 把某件事作为另一件事导入
从模块导入函数时:
import somemodule
或者:
from somemodule import somefunction
或者:
from somemodule import somefunction,antherfunction,yetanotherfunction
或者:
from somemodule import *
只有确定自己想要从给定的模块导入所有功能时,才应用最后一个版本,如果两个模块都有open 函数,只需要使用第一种方式导入,
然后像下面使用函数:
module1.open(...)
module2.open(...)
还有另外一个选择,可以在语句末尾加上一个as语句,在该子句后给出名字,或者为整个模块提供别名
import math as foobar
>>> foobar.sqrt(4)
2.0
也可以为函数提供别名
>>> from math import sqrt as foobar
>>> foobar(4)
2.0
对于open函数,可以像下面这样使用:
from module1 import open as open1
from module2 import open as open2
5.2赋值魔法
5.2.1 序列解包
多个赋值可以同时操作:
>>> x,y,z=1,2,3
>>> x
1
也可以交换两个变量的值:
>>> x,y=y,x
>>> x
2
这里所做的叫做序列解包:将多个值的序列解开,然后放到变量中的序列中
>>> values=1,2,3
>>> x,y,z=values
>>> x
1
例子:将字典的任意的键-值对,使用popitem方法作为元组返回,这个元组就可以直接赋值到两个变量中
>>> seq={'name':'test','age':'22'}
>>> q,w=seq.popitem()
>>> q
'age'
>>> w
'22'
解包的序列总的元素数量必须和防止在赋值符号=左边的变量数量一致
5.2.2 链式赋值
是将同一个值赋给多个变量的捷径
x=y=somefunction()
和下面的效果一样
y=somefunction()
x=y
注意上面的语句和下面的语句不一定等价
x=somefunction()
y=somefunction()
5.2.3 增量赋值
+= *= /= -= 标准运算符都适用
5.3 语句块:缩排
Python将table字符解释为到下一个tab字符位置的移动,一个tab字符是8位,推荐适用空格,每个缩进需要4个空格
5.4 条件和条件语句
5.4.1 布尔变量的作用
线面的值在作为布尔表达式的时候,会被解释器看做假、
False None 0 "" () [] {}
也就是标准的False、None和所有类型的数字0、空序列、空元祖都为假,其它的一切解释为真,也就是python的所有值都能解释为真
>>> True
True
>>> False
False
>>> False==0
True
如果某个逻辑表达式返回1或者0,实际的意思是返回True和Flase
5.4.2 条件执行和if语句
name=raw_input("what's your name:")
if name.endswith('Gumby'):
print "hello,mr.Gumby!"
what's your name:Gumby
hello,mr.Gumby!
如果条件为真,print就会执行,条件为假,就不会执行
5.4.3 else子句
name=raw_input("what's your name:")
if name.endswith('Gumby'):
print "hello,mr.Gumby!"
else:
print "hello,stranger"
如果第一个print没有被执行,就会执行下面的pting语句块
5.4.4 elif子句
如果需要检查过个条件,就可以使用elif,它是“else if”的简写,也是if 和else子句的联合使用
num=input("enter a number:")
if num > 0:
print "the number is postive"
elif num < 0:
print "the number is negative"
else:
print "the number is zero"
enter a number:3
the number is postive
enter a number:0
the number is zero
enter a number:-1
the number is negative
5.4.5 嵌套代码块
if语句里面可以嵌套使用if语句
name = raw_input("enter your name:")
if name.endswith('Gumby'):
if name.startswith('Mr.'):
print 'Hello,Mr.Gumby'
elif name.startswith('Mrs.'):
print 'Hello,Mrs.Gumby'
else:
print 'Hello,Gumby'
else:
print 'Hello stanger'
5.4.6 更复杂的条件
1、比较运算符
2、相等运算符
>>> 'test'=='test'
True
3、is 同一性运算符
>>> z=[1,2,3]
>>> x=y=[1,2,3]
>>> x==y
True
>>> x==z
True
>>> x is y
True
>>> x is z
False
is判断同一性不判断相等性
4 in 成员资格运算符
>>> s='sss'
>>> s in ['sss','yyy']
True
5 字符串和序列比较
字符串可以按照字母顺序比较
>>> 's'>'d'
True
>>> 'a'>'b'
False
如果字符串内包换大小写字母,可以忽略大小写字母,使用lower
>>> 'AAa'.lower > 'BBb.lower'
False
其它序列也可以按照同样的方式比较
6布尔运算符
如果需要检查数字是否在1-10之间
number = input('enter a number between 1 and 10:')
if 0 < number <= 10:
print 'greet'
else:
print 'false'
and 运算符可以连接两个布尔值,在两者都为真的时候返回真
另外两个还有 or 和 not
5.4.7 断言 assert
如果需要确保程序中的某个条件一定为真才能让程序正常工作的话,assert语句可以在程序中置入检查点
>>> age =1
>>> assert 1< age <100 ,'the age must be realistic'
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AssertionError: the age must be realistic
>>> assert 0< age <100 ,'the age must be realistic'
5.5 循环
5.5.1 while循环
>>> x=1
>>> while x <= 100:
print x
x+=1
一个循环就可以确保用户输入了名字
name=''
while not name:
name=raw_input('please enret your name:')
print 'hello,%s' %name
确保用户输入名字:
name=raw_input('enter your name: ')
while not name:
name=raw_input('enter your name: ')
print 'hello,%s'%name
空格也会被判断为名字:可以将while not name 改为while not name or name.isspace() 或者while not name.strip()
name=raw_input('enter your name: ')
while not name.strip():
name=raw_input('enter your name: ')
print 'hello,%s'%name
5.5.2 while语句可以用来在任何条件为真的情况下重复执行一个代码块,for 循环,例如为一个集合的每个元素都执行一个代码块
names=['my','name','is','yin','ya','liang']
for test in names:
print test
range 包含下限0 不包含上限
>>> range(10)
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> for i in range(10):
print i
5.5.3 循环遍历字典元素
d={'x':'1','y':'2','z':'3'}
for test in d:
print test,'corresponds to',d[test]
y corresponds to 2
x corresponds to 1
z corresponds to 3
d={'x':'1','y':'2','z':'3'}
for test,value in d.items():
print test,'corresponds to',value
y corresponds to 2
x corresponds to 1
z corresponds to 3
5.5.4 一些迭代工具
1、并行迭代 程序可以同时迭代两个序列
name=['age','beth','george','damon']
ages=[12,45,32,102]
for i in range (len(name)):
print name[i],'is', ages[i],'years old'
i是循环索引的标准变量名
zip函数可以用来并行迭代,可以把两个序列‘’压缩‘’在一起,然后返回一个元组的值
name=['age','beth','george','damon']
ages=[12,45,32,102]
zip(name,ages)
for name,age in zip(name,ages):
print name,'is',age,'years old'
打印zip(name,ages)
[('age', 12), ('beth', 45), ('george', 32), ('damon', 102)]
2 编号迭代
一个字符串列表中替换所有包含'xxx'的字符串
name=['wang','zhang','li','zhao']
for string in name:
if 'wang' in string:
index = name.index(string)
name[index]= '[sun]'
print name
更改的版本如下:
name=['wang','zhang','li','zhao']
index=0
for string in name:
if 'wang' in string:
name[index]= '[sun]'
index += 1
print name
还有一种方法使用enumerate模块
name=['wang','zhang','li','zhao']
for index, string in enumerate(name):
if 'wang' in string:
name[index]='[sun]'
print name
3、反转和排序迭代
>>> sorted([5,3,7,89,4,6,5,33,56,7,4])
[3, 4, 4, 5, 5, 6, 7, 7, 33, 56, 89]
>>> list(reversed('hello,world!'))
['!', 'd', 'l', 'r', 'o', 'w', ',', 'o', 'l', 'l', 'e', 'h']
>>> ''.join(reversed('hello,world!'))
'!dlrow,olleh'
5.5.5 跳出循环
1、break
跳出循环可以使用break
>>> for n in range(99,0,-1):
a=sqrt(n)
if a==int(a):
print n
break
81
2 continue 会让当前的迭代结束,×××下一轮循环的开始,也就是 : 跳出剩余的循环体,但是不结束循环
3、while True/break
>>> while True:
world=raw_input('enter your name:')
if not world:break
print 'your name is ',world
enter your name:test
your name is test
enter your name:
5.5.6 循环中的else子句
for n in range(99,81,-1):
a=sqrt(n)
if a==int(a):
print n
break
else:
print 'Didn't find it'
5.6列表推导式-轻量级循环
>>> [x*x for x in range(10)]
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
>>> [x*x for x in range(10) if x % 3==0]
[0, 9, 36, 81]
>>> [(x,y) for x in range(3) for y in range(3)]
[(0, 0), (0, 1), (0, 2), (1, 0), (1, 1), (1, 2), (2, 0), (2, 1), (2, 2)]
5.7 三人行 pass del exec
5.7.1 什么都没有发生
if name =="palph auldus melish":
print 'welcome!'
elif name =='enid':
#还没完
pass
elif name=='bill gates':
print 'access deny'
5.7.2 使用del删除
>>> a={'test':42}
>>> b=a
>>> a
{'test': 42}
>>> b
{'test': 42}
>>> a=None
>>> a
>>> b
{'test': 42}
当把b也置为None时,字典就“飘”在了内存里,python解释器会直接删除那个字典,成为垃圾收集,另外一个方法就是使用del语句,它不仅会移除对象的引用,也会移除那个名字本身
>>> a=1
>>> del a
>>> a
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'a' is not defined
python中是无法删除值的
5.7.3 使用exec和eval执行和求值字符串
有时候可以能需要动态的创造python代码,将其作为语句执行或作为表达式计算
下面学习执行存储在字符串中的python代码,这样做会有潜在的安全漏洞
>>> exec "print 'hello world'"
hello world
很多情况下给它提供一个命名空间,或者称为作用域
>>> from math import sqrt
>>> exec "sqrt = 1"
>>> sqrt(4)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'int' object is not callable
命名空间或称为作用域(scope)
>>> from math import sqrt
>>> scope={}
>>> exec 'sqrt=1' in scope
>>> sqrt(4)
2.0
>>> scope['sqrt']
1
2 eval
exec语句会执行一系列的pythone语句,而eval会计算python表达式(以字符串形式书写),并且返回结果值
>>> eval(raw_input("enter you number:"))
enter you number:2*8
16
eval(raw_input(...))相当于input(...)
和exec一样eval也可以使用命名空间,提供两个命名空间,全局和局部,全局必须是字典,局部的可以是任何形式的映射
>>> scope={}
>>> scope['x']=2
>>> scope['y']=3
>>> eval('x*y',scope)
6
>>> scope={}
>>> exec 'x=2' in scope
>>> eval('x*x',scope)
4
5.8 结
打印 print语句可以用来打印由逗号隔开的多个值,如果语句以逗号结尾,后面的print语句会在同一行内继续打印
导入 可以使用import ... as ... 语句进行函数的局部重命名
赋值 通过序列解包和链式赋值功能,多个变量赋值可以一次性赋值,通过增量赋值可以原地改变变量
块 通过缩排使语句成组的一种方法
条件 条件语句可以根据条件执行或者不执行一个语句块,几个条件可以串联使用if/elif/else
断言 简单说就是肯定某事为真,也可在后面更上这么认为的原因,如果条件为假,断言就会让程序崩溃
循环 可以为序列中的每一个元素执行一个语句块,或者在条件为真的时候继续执行一段语句。
列表推导式 可以从旧列表产生新的列表、对元素应用函数、过滤不需要的元素等等
5.8.1 本章的新函数
上一篇:第四章 当索引不好用时
下一篇:zabbix: Zabbix server is not running:the information displayed may not be current
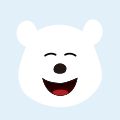
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
第五章:if语句
python
if语句 大小写 不执行 -
第五章 多态
1、如果基类的某个行为,在所有的派生类中的表现都是一样的,说明在所有的派生类中都没有必要对基类中的行为进行重新实现,那么基类中的该行为
c++ 开发语言 虚函数 运算符 友元函数 -
Transformer【第五章】
Transformer 是一个 Sequence-to-sequence 的 Model(缩写 Seq2seq) input 是 一个 se
transformer 深度学习 人工智能 语音辨识 机器翻译 -
第五章循环结构(一)
一、while循环:1.语法:while(循...
while循环 程序调试 i++