import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
class BTree {
String data = null;
BTree rightChild = null;
BTree leftChild = null;
}
public class Tree {
static void preOrder(BTree root) {
if (root == null) {
return;
}
System.out.print(root.data);
preOrder(root.leftChild);
preOrder(root.rightChild);
}
static void inOrder(BTree root) {
if (root == null) {
return;
}
inOrder(root.leftChild);
System.out.print(root.data);
inOrder(root.rightChild);
}
static BTree createTree(BTree root) {
String c = null;
System.out.print("请输入一个字符型:");
BufferedReader strin = new BufferedReader(new InputStreamReader(
System.in));
try {
c = strin.readLine();
} catch (IOException e) {
e.printStackTrace();
}
if (c.equals("#")) {
root = null;
} else {
root = new BTree();
root.data = c;
root.leftChild = new BTree();
root.rightChild = new BTree();
root.leftChild = createTree(root.leftChild);
root.rightChild = createTree(root.rightChild);
}
return root;
}
static BTree createTree() {
String c = null;
System.out.print("请输入一个字符型:");
BufferedReader strin = new BufferedReader(new InputStreamReader(
System.in));
try {
c = strin.readLine();
} catch (IOException e) {
e.printStackTrace();
}
BTree root = new BTree();
if (c.equals("#")) {
root = null;
} else {
root.data = c;
root.leftChild = createTree();
root.rightChild = createTree();
}
return root;
}
static BTree createTree(String str, int current) {
char c = str.charAt(current);
BTree root = new BTree();
if (c == '#') {
root = null;
} else {
root.data = String.valueOf(c);
root.leftChild = createTree(str, current * 2 + 1);
root.rightChild = createTree(str, current * 2 + 2);
}
return root;
}
static int depth(BTree root) {
int result=0;
int left=0;
int right=0;
if (root != null) {
if (root.leftChild != null){
left = depth(root.leftChild);}
if (root.rightChild != null){
right = depth(root.rightChild);}
if (left > right) {
result= left + 1;
} else {
result= right + 1;
}
}
return result;
}
public static void main(String args[]) throws IOException {
BTree root = null;
String str = "ABCD#####";
root = createTree(str, 0);
System.out.println("-------------------------");
preOrder(root);
System.out.println("\n-------------------------");
inOrder(root);
System.out.println("\n-------------------------");
System.out.print(depth(root));
}
}
二叉树操作
原创
©著作权归作者所有:来自51CTO博客作者TechOnly的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:从顶部开始逐层打印二叉树结点数据
下一篇:hadoop源代码
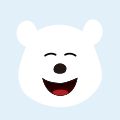
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
【数据结构】二叉树的存储结构
【数据结构】第五章——树与二叉树详细介绍二叉树的存储结构……
二叉树 数据结构 C语言 -
【二叉树】重建二叉树
题目输入某二叉树的前序遍历和中序遍历的结果请构建该二叉树并返回其根节点假设
swift 算法 二叉树 中序遍历 子树 -
【二叉树】验证二叉树
题目二叉树上有 n 个节点,按从 0 到 n - 1 编号其中节点 i 的两个子节点分别是 leftChild[i]
swift 二叉树 算法 子节点 数组 -
【二叉树】平衡二叉树
题目给你一个二叉树判断它是否是高度平衡的二叉树一棵高度平衡二叉树定义为:一
swift 算法 开发语言 ios objective-c -
【二叉树】输出二叉树
题目在一个 m*n 的二维字符串数组中输出二叉树行数 m 应当等于给定二叉树的 高度列数 n 应当总是 奇数根节点 的值(以字符串格式
swift 算法 深度优先 二叉树 子树 -
对称二叉树【二叉树】
时间复杂度:空间复杂度:
python 复杂度 Code 空间复杂度 -
【二叉树】合并二叉树
题目给定两个二叉树想象当你将它们中的一个 覆盖 到另一个上时两个二叉树的一些节点便
swift 深度优先 算法 ios objective-c -
满二叉树、完全二叉树、非完全二叉树mysql
-
二叉树的操作
二叉树的操作(递归实现)
二叉树 结点