// innerclasses/Callbacks.java
// Using inner classes for callbacks
package innerclasses;
interface Incrementable{
void increment();
}// Very simple to just implement the interface
class Callee1 implements Incrementable {
private int i = 0;
public void increment() {
i++;
System.out.println(i);
}
}
class MyIncrement {
public void increment() {System.out.println("Other operation");}
static void f(MyIncrement mi) {mi.increment();}
}
// If your class must implement increment() in
// some other way, you must use an inner class:
class Callee2 extends MyIncrement {
private int i=0;
public void increment() {
super.increment();
i++;
System.out.println(i);
}
private class Closure implements Incrementable {
public void increment() {
// Specify outer-class method, otherwise
// you'd get an infinite recursion
Callee2.this.increment();
}
}
Incrementable getCallbackReference() {
return new Closure();
}
}
class Caller {
private Incrementable callbackReference;
Caller(Incrementable cbh) {callbackReference = cbh;}
void go() {callbackReference.increment();}
}
public class Callbacks {
public static void main(String[] args) {
Callee1 c1 = new Callee1();
Callee2 c2 = new Callee2();
MyIncrement.f(c2);
//Other operation
//1
Caller caller1 = new Caller(c1);
Caller caller2 = new Caller(c2.getCallbackReference());
caller1.go();
//1
caller1.go(); //2
caller2.go(); //Other operation
//2 caller2.go(); //Other operation
//3
}
}
// innerclasses/Callbacks.java // Using inner classes for callbacks
转载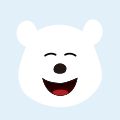
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Android编译解决:Missing classes detected while running R8.
Android编译相关问题解决
Android 代码混淆 配置文件 -
jquery09--Callbacks : 回调对象
无标题文档 无标题文档 无标题文档
html jquery 作用域 观察者模式 不执行