1.操作mysql代码; # -*- coding: utf-8 -*- import MySQLdb def get_conn(): host = "192.168.xxx.xx" port = 3306 db = "Scier" user = "root" password = "123456" conn = MySQLdb.connect(host, user, password, db, port, charset='utf8') return conn class User(object): def save(self,type, name, url, create_time, create_founder): self.type = type self.name = name self.url = url self.create_time = create_time self.create_founder = create_founder conn = get_conn() cursor = conn.cursor() sql = ("insert into naming (type,name,url,create_time,create_founder) VALUES (%s,%s,%s,%s,%s)") data = (self.type, self.name, self.url, self.create_time, self.create_founder) try: cursor.execute(sql, data) conn.commit() cursor.close() conn.close() except: cursor.close() conn.close() return "Error: unable to table naming" def Query_naming(self,type1): conn = get_conn() cursor = conn.cursor() self.type1 = type1 # SQL 查询语句命名规范名称和url地址字段####### sql = "SELECT NAME,url FROM naming WHERE TYPE='%s'" % self.type1 try: # 执行SQL语句 cursor.execute(sql) # 获取所有记录列表 results = cursor.fetchall() cursor.close() conn.close() return results except: cursor.close() conn.close() return "Error: unable to fecth data" name = User() abc = name.Query_naming('发布规范类') abc = name.Query_naming('环境治理类')
Python 原生模块 操作Mysql
原创
©著作权归作者所有:来自51CTO博客作者breaklinux的原创作品,请联系作者获取转载授权,否则将追究法律责任
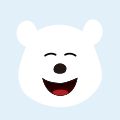
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
python模块--Telnetlib模块
telnet模块
ci 用户名 for循环 -
05 go操作mysql(原生)
01go操作mysql.go 02go使用sqlx操作mysql.go
sql mysql 数据 -
python中操作mysql的pymysql模块详解
pymsql是Python中操作MySQL的模块,其使用方法和MySQLd
pymysql mysql sql 数据 -
python qpy模块 python操作qq 模块
Python3控制QQ窗口这里写一个简单的控制,其他的窗口也一个样 EnumWindows (需要回调的函数,参数): 该函数枚举所有屏幕上的顶层窗口, 并将窗口句柄传送给应用程序定义的回调函数。 回调函数返回FALSE将停止枚举, 否则EnumWindows函数继续到所有顶层窗口枚举完为止。 GetWindowText(hwnd):获得窗口句柄hwnd指向的窗口标题。import win32gu
python qpy模块 Python3控制QQ窗口 2.控制窗口的大小和位置 1.显示和隐藏窗口(以QQ为例) GetWindowText