var COREHTML5 = COREHTML5 || {}
// Constructor....................................................
COREHTML5.Progressbar = function(strokeStyle, fillStyle,
horizontalSizePercent,
verticalSizePercent) {
this.trough = new COREHTML5.RoundedRectangle(strokeStyle,
fillStyle,
horizontalSizePercent,
verticalSizePercent);
this.SHADOW_COLOR = 'rgba(255,255,255,0.5)';
this.SHADOW_BLUR = 3;
this.SHADOW_OFFSET_X = 2;
this.SHADOW_OFFSET_Y = 2;
this.percentComplete = 0;
this.createCanvases();
this.createDOMElement();
return this;
}
// Prototype......................................................
COREHTML5.Progressbar.prototype = {
createDOMElement: function () {
this.domElement = document.createElement('div');
this.domElement.appendChild(this.context.canvas);
},
createCanvases: function () {
this.context = document.createElement('canvas').
getContext('2d');
this.offscreen = document.createElement('canvas').
getContext('2d');
},
appendTo: function (element) {
element.appendChild(this.domElement);
this.domElement.style.width =
element.offsetWidth + 'px';
this.domElement.style.height =
element.offsetHeight + 'px';
this.resize(); // obliterates everything in the canvases
this.trough.resize(element.offsetWidth,
element.offsetHeight);
this.trough.draw(this.offscreen);
},
setCanvasSize: function () {
var domElementParent = this.domElement.parentNode;
this.context.canvas.width = domElementParent.offsetWidth;
this.context.canvas.height = domElementParent.offsetHeight;
},
resize: function () {
var domElementParent = this.domElement.parentNode,
w = domElementParent.offsetWidth,
h = domElementParent.offsetHeight;
this.setCanvasSize();
this.context.canvas.width = w;
this.context.canvas.height = h;
this.offscreen.canvas.width = w;
this.offscreen.canvas.height = h;
},
draw: function (percentComplete) {
if (percentComplete > 0) {
// Copy the appropriate region of the foreground canvas
// to the same region of the onscreen canvas
this.context.drawImage(
this.offscreen.canvas, 0, 0,
this.offscreen.canvas.width*(percentComplete/100),
this.offscreen.canvas.height,
0, 0,
this.offscreen.canvas.width*(percentComplete/100),
this.offscreen.canvas.height);
}
},
erase: function() {
this.context.clearRect(0, 0,
this.context.canvas.width,
this.context.canvas.height);
},
};
//..................................................
var startButton = document.getElementById('startButton'),
loadingSpan = document.getElementById('loadingSpan'),
progressbar = new COREHTML5.Progressbar(
'rgba(0, 0, 0, 0.2)', 'teal', 90, 70),
percentComplete = 0;
progressbar.appendTo(document.getElementById('progressbarDiv'));
startButton.onclick = function (e) {
loadingSpan.style.display = 'inline';
startButton.style.display = 'none';
percentComplete += 1.0;
if (percentComplete > 100) {
percentComplete = 0;
loadingSpan.style.display = 'none';
startButton.style.display = 'inline';
}
else {
progressbar.erase();
progressbar.draw(percentComplete);
requestNextAnimationFrame(startButton.onclick);
}
};
进度条Progressbar47
原创
©著作权归作者所有:来自51CTO博客作者生而为人我很遗憾的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:滑动条Slider48
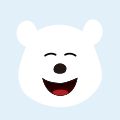
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
控制台打印进度条
控制台打印进度条,实时展示任务进度。
进度条 System Java -
Android进度条progressbar
Android进度条
ProgressBar 进度条