var Sprite = function (name, painter, behaviors) {
if (name !== undefined) this.name = name;
if (painter !== undefined) this.painter = painter;
if (behaviors !== undefined) this.behaviors = behaviors;
return this;
};
Sprite.prototype = {
left: 0,//左上角X坐标
top: 0,//左上角Y坐标
width: 10,//宽度
height: 10,//高度
velocityX: 0,//水平速度
velocityY: 0,//垂直速度
visible: true,//是否可见的标志位
animating: false,//是否正在执行动画效果的标志位
painter: undefined, // 精灵对象绘制器object with paint(sprite, context)
behaviors: [], // 包含精灵行为对象的数组,在执行更新逻辑时,此数组中的各行为对象会被用于此精灵(objects with execute(sprite, context, time)
paint: function (context) {//绘制操作,具体绘制过程由外面的代码决定,这也是一个解耦的过程
if (this.painter !== undefined && this.visible) {
this.painter.paint(this, context);
}
},
update: function (context, time) {//执行精灵的行为,执行的顺序是这些行为被加入精灵的顺序,一般定义不同的行为来更改精灵的属性或外观
for (var i = this.behaviors.length; i > 0; --i) {
this.behaviors[i-1].execute(this, context, time);
}
}
};
//...............................................................................
var context = document.getElementById('canvas').getContext('2d'),
RADIUS = 75,
ball = new Sprite('ball',
{
paint: function(sprite, context) {//这里的sprite是在上面对象中定义的,在设计上有个代理过程,在后面过程中这个绘制器可以用一个专门的对象来完成
context.beginPath();
context.arc(sprite.left + sprite.width/2,
sprite.top + sprite.height/2,
RADIUS, 0, Math.PI*2, false);
context.clip();//注意此方法的使用
context.shadowColor = 'rgb(0,0,0)';
context.shadowOffsetX = -4;
context.shadowOffsetY = -4;
context.shadowBlur = 8;
context.lineWidth = 2;
context.strokeStyle = 'rgb(100,100,195)';
context.fillStyle = 'rgba(30,144,255,0.15)';
context.fill();
context.stroke();
}
}
);
ball.left = 120;//设置其它属性
ball.paint(context);//如果把此条语句放在其它方法中通用,通过改变外部的环境参数和精灵的参数,就可以用一个Sprite来绘制多个同样的对象,因为canvas绘制后就丢弃了内存,但如果想自己缓存精灵对象时,还是要创建多个对象。大大节省了对象的个数;
Sprite对象25
原创
©著作权归作者所有:来自51CTO博客作者生而为人我很遗憾的原创作品,请联系作者获取转载授权,否则将追究法律责任
上一篇:如何成为一名合格的软件架构师
下一篇:精灵Sprite绘制器26
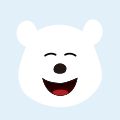
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
承前启后,Java对象内存布局和对象头JVM Java
-
25、TS内置对象
TypeScript 核心库的定义文件中定义了所有浏览器环境需要用到的类型,并且是预置在 TypeScript 中的。上面的例子中,Math.pow 必须接受
ts typescript TS内置对象 内置对象 Math -
harmonyos自定义组件如何导入
一.自定义组件使用(1)自定义组件创建 JS UI框架支持自定义组件,用户可根据业务需求将已有的组件进行扩展,增加自定义的私有属性和事件,封装成新的组件,方便在工程中多次调用,提高页面布局代码的可读性。1.创建新的模板2. 修改首页,通过C:\Users\86156\DevEcoStudioProject
harmonyos自定义组件如何导入 javascript 开发语言 ecmascript 自定义组件