<!DOCTYPE html> <html lang="en" ng-app="myapp"> <head> <meta charset="UTF-8"> <title>Document</title> <style> body, html{ width:100%; height:100%; margin:0; padding:0; white-space:nowrap; font-family:Arial,"Hiragino Sans GB","微软雅黑","宋体";} ul, li, h4, p{ margin:0; padding:0; } li{ list-style:none; } h4{ margin-bottom:6px; } p{ margin-bottom:6px; } #bg{ width:100%; height:100%; background:#FAFB98; display:inline-block; } #owrap{ width:800px; height:460px; position:relative; left:calc(50% - 400px); top:calc(50% - 230px); border-radius:30px; box-shadow:0 10px 28px #E0D272; transition:all ease 0.3s; -webkit-transition:all ease 0.3s; -moz-transition:all ease 0.3s; -ms-transition:all ease 0.3s; background:#fff; } #obox{ width:100%; height:460px; position:relative; transition:all ease 0.3s; -webkit-transition:all ease 0.3s; -moz-transition:all ease 0.3s; -ms-transition:all ease 0.3s; opacity:1; } #oul{ width:100%; overflow:auto; position:relative; display:inline-block; } #oul a{ width:18px; height:18px; color:#909696; line-height:18px; border:1px solid #909696; margin-bottom:6px; text-align:center; text-decoration:none; display:inline-block; } #oul span{ margin:0 5px 0 5px; } #oul li div{ width:110px; height:22px; border-radius:6px; text-align:center; line-height:22px; font-size:14px; cursor:pointer; background:#FF6F3C; color:#fff; } #oul .li_adds{ border:5px dotted #FF6F3C; } #oul .have_adds{ background:#FCDD68; } #oul li{ width:130px; height:116px; padding:10px 0 0 20px; list-style:none; border:5px dotted #DBE3E4; float:left; margin:20px 20px 0 20px; border-radius:20px; } .oall{ width:200px; height:50px; line-height:50px; text-align:center; font-size:20px; letter-spacing:10px; display:inline-block; position:absolute; left:50px; bottom:30px; transition:all ease 0.3s; -webkit-transition:all ease 0.3s; -moz-transition:all ease 0.3s; -ms-transition:all ease 0.3s; } .oconfirm{ width:200px; height:50px; line-height:50px; text-align:center; font-size:25px; letter-spacing:10px; background:#FF6F3C; color:#fff; display:inline-block; text-decoration:none; border-radius:25px; position:absolute; left:550px; bottom:30px; transition:all ease 0.3s; -webkit-transition:all ease 0.3s; -moz-transition:all ease 0.3s; -ms-transition:all ease 0.3s; } #items{ width:100%; overflow:hidden; padding-top:20px; } #items li{ width:calc(100% - 40px); height:30px; line-height:30px; color:#677A7A; padding:0 20px 0 20px; position:relative; } #items li .each_all{ width:200px; display:inline-block; position:absolute; left:150px; } .even{ background:#DBE3E4; } </style> </head> <body> <div id="bg" ng-controller="ctrler"> <div id="owrap"> <div id="obox" ng-view> </div> <span id="ospan" class="oall" ng-bind-template="总金额:{{ all_cal.num }}元"></span> <a href="#go_buy" ng-click="go_cal( $event )" class="oconfirm" ng-click="go_cal()">~去结算~</a> </div> </div> <script src="http://cdn.bootcss.com/angular.js/1.3.15/angular.min.js"></script> <script src="http://cdn.bootcss.com/angular.js/1.3.15/angular-route.min.js"></script> <script type="text/javascript"> var m1 = angular.module("myapp", ['ngRoute']); m1.config(['$routeProvider', function( $routeProvider ){ $routeProvider .when("/", { template : "<ul id='oul'>\ <li ng-repeat='fruit in ifruit' ng-controller='eachCtrl' class='`li_border`'>\ <h4 ng-bind='fruit.name'></h4>\ <p>单价:<span ng-bind='fruit.money'></span></p>\ 数量:<a href='javascript:;' ng-click='oreduce()'>-</a>\ <span ng-bind='fruit.num'></span>\ <a href='javascript:;' ng-click='oadd()'>+</a>\ <div class='`have_click`' ng-click='addTo( $event )' ng-bind='ocon'></div></li></ul>" }) .when("/go_buy", { template : "<ul id='items'>\ <li ng-repeat='ofruit in new_arr' ng-class='{ even : $even ? true : false }' ng-hide='ofruit.num == 0'>\ <span ng-bind-template='{{ ofruit.name }}:{{ ofruit.money }} x {{ ofruit.num }} '></span>\ <span class='each_all' ng-bind-template=' ************************ {{ ofruit.money * ofruit.num }} '></span></li></ul>" }) }]); m1.controller("ctrler", ["$scope", "$timeout", function($scope, $timeout){ $scope.all_cal = { num : 0 }; $scope.arr_fruit = [ {name:"香蕉", money:5, num:1, buy:false}, {name:"苹果", money:9, num:1, buy:false}, {name:"雪梨", money:6, num:1, buy:false}, {name:"西瓜", money:10, num:1, buy:false}, {name:"火龙果", money:8, num:1, buy:false}, {name:"荔枝", money:11, num:1, buy:false}, {name:"榴莲", money:18, num:1, buy:false}, {name:"哈密瓜", money:8, num:1, buy:false} ]; //点击去结算 $scope.ifruit = $scope.arr_fruit; $scope.oturn = true; $scope.go_cal = function( event ){ $scope.new_arr = []; //筛选出已加入购物车的水果 for( var i=0; i<$scope.arr_fruit.length; i++ ){ if( $scope.arr_fruit[i].buy == true ){ $scope.new_arr.push( $scope.arr_fruit[i] ); } } if( $scope.oturn ){ event.target.href = "#go_buy"; event.target.innerHTML = "返回"; setStyle( event.target, {'height':'30px', 'left':'calc(50% - 100px)', 'fontSize':'18px', 'lineHeight':'30px'} ); setStyle( event.target.parentNode, {'width':'400px', 'borderRadius':'0', 'left':'calc(50% - 200px)'} ); setStyle( event.target.parentNode.children[0], {'opacity':0}); setStyle( event.target.parentNode.children[1], {'left':'calc(50% - 100px)', 'bottom':'60px'});//不知道为什么这里不能用childNodes[1]无效 $scope.oturn = false; $scope.ifruit = []; } else{ event.target.href = "#/"; event.target.innerHTML = "~去结算~"; setStyle( event.target, {'height':'50px', 'left':'550px', 'fontSize':'25px', 'lineHeight':'50px'} ); setStyle( event.target.parentNode, {'width':'800px', 'borderRadius':'30px', 'left':'calc(50% - 400px)'} ); setStyle( event.target.parentNode.children[1], {'left':'50px', 'bottom':'30px'}); $scope.oturn = true; //设置延迟出现以防瞬移突兀 $timeout(function(){ $scope.ifruit = $scope.arr_fruit; }, 400); } }; }]); m1.controller("eachCtrl", ["$scope", function($scope){ var oindex = $scope.$index; if( $scope.arr_fruit[oindex].buy ){ $scope.li_border = "li_adds"; $scope.ocon = "取消购买"; $scope.have_click = "have_adds"; } else{ $scope.li_border = ""; $scope.ocon = "添加到购物车"; $scope.have_click = ""; } //点击 - 号时 $scope.oreduce = function(){ if( $scope.arr_fruit[oindex].num == 1 ){ return; } $scope.arr_fruit[oindex].num = $scope.arr_fruit[oindex].num - 1; if( $scope.arr_fruit[oindex].buy ){ $scope.all_cal.num = $scope.all_cal.num - $scope.fruit.money ; } }; //点击 + 号时 $scope.oadd = function(){ if( $scope.arr_fruit[oindex].num == 99 ){ return; } $scope.arr_fruit[oindex].num = $scope.arr_fruit[oindex].num + 1; if( $scope.arr_fruit[oindex].buy ){ $scope.all_cal.num = $scope.all_cal.num + $scope.fruit.money ; } }; //点击添加到购物车时 $scope.addTo = function( event ){ if( $scope.arr_fruit[oindex].buy ){ $scope.ocon = "添加到购物车"; $scope.all_cal.num = $scope.all_cal.num - $scope.fruit.money * $scope.arr_fruit[oindex].num; $scope.arr_fruit[oindex].buy = false; $scope.li_border = ""; $scope.have_click = ""; } else{ $scope.ocon = "取消购买"; $scope.all_cal.num = $scope.all_cal.num + $scope.fruit.money * $scope.arr_fruit[oindex].num; $scope.arr_fruit[oindex].buy = true; $scope.li_border = "li_adds"; $scope.have_click = "have_adds"; } }; }]); function setStyle( obj, json ){ for( attr in json ){ obj.style[attr] = json[attr]; } } </script> </body> </html>
angularJS制作的购物车功能
精选 转载下一篇:动态设置ng-model
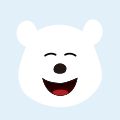
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
购物车的实现(未登录时也可以使用)
平时工作的踩坑记录
redis List 拦截器 -
AngularJS实现购物车
用AngularJS实现购物车,具体代码如下:<!DOCTYPE html><html ng-app="app"><head> <meta charset="utf-8"> <link&nb
购物车 AngularJS Angular ng-repeat ng-click -
javasession购物车 java购物车功能
本篇文章讲的是如何使用javaweb相关知识模拟购物车功能(web练手小项目)使用到的相关知识(部分知识点在文章中简单涉及到): html cs javascript jsp servlet ajax jQuery Mysql M
javasession购物车 mybatis java mysql servlet