# 12.14学习
# 字符串生成,列表推导式
planet = 'Pluto'
planet = [char+'! ' for char in planet]
# ['P! ', 'l! ', 'u! ', 't! ', 'o! ']
'''---------------------我是分割线啦啦啦------------------------'''
# 12.14学习
# 字符串方法
'''
1.upper()
将字符串里面所有英文大写(小写转为大写)
str.upper() --> return str
claim = "Pluto is a planet!"
claim.upper() -> 'PLUTO IS A PLANET!'
2.lower()
将字符串里面所有英文小写(大写转小写)
str.lower() --> return str
claim.lower() -> 'pluto is a planet!'
3.index()
搜索子字符串的第一个索引(即满足搜索的第一个字符串的下标,返回)
str.index('str_index')
claim.index('plan') -> 11
[因为字符串跟列表相像,所以第11个字符串元素是p所以返回11,并且后面是lan满足plan]
4.startswith()
检查字符串是否以指定的子字符串开头(输入的子字符串是否是该字符串的开头),是则True、不是则False
str.startswith('str_header')
claim.startswith('P') -> True
claim.startswith('planet') -> False
5.endswith()
检查字符串是否以指定的子字符串结尾(输入的子字符串是否是该字符串的结尾),是则True、不是则False
str.endswith()
claim.endswith('planet') -> False
claim.endswith('!') -> True
6.在字符串和列表之间切换:
6.1:.split()
str.split() 将字符串转换为较小字符串的列表,默认情况下会中断空格。
1.常用:一个大字符串转换为单词列表
words = claim.split() -> ['Pluto', 'is', 'a', 'planet!']
2.在空格以外的东西上拆分:
datestr = '1956-01-31'
year, month, day = datestr.split('-')
print(year, month, day) # 1956 01 31
6.2:.join()
str.join() 将字符串列表缝合成一个长字符串,使用调用它的字符串作为分隔符。
常用:字符串拼接、网址拼接
1.将字符串列表缝合成一个长字符串,使用/连接下一个列表元素
print('/'.join([month, day, year])) # '01/31/1956'
print('/'.join(['19','23', '1009'])) # 19/23/1009
2.使用列表推导式将列表元素取出后转为大写,在join拼接,用 👏 连接
print(' 👏 '.join([word.upper() for word in words])) # PLUTO 👏 IS 👏 A 👏 PLANET!
7.使用 .format() 构建字符串(将字符串与 + 运算符连接起来)
7.1:+号运算符
planet + ', we miss you.'
# 'Pluto, we miss you.'
7.2:str.format()
'字符串{}'.format(变量名1,变量名2,变量3....)
要插入的 Python 值用 {} 占位符表示,不必调用 str()将其他类型变量转换为str类型也可以使用变量
"{}, you'll always be the {}th planet to me.".format(planet, position)
# "Pluto, you'll always be the 9th planet to me."
7.3:
s = """Pluto's a {0}.
No, it's a {1}.
{0}!
{1}!""".format('planet', 'dwarf planet')
print(s)
Pluto's a planet.
No, it's a dwarf planet.
planet!
dwarf planet!
7.4:
pluto_mass = 1.303 * 10**22
earth_mass = 5.9722 * 10**24
population = 52910390
# 2 decimal points 3 decimal points, format as percent separate with commas
"{} weighs about {:.2} kilograms ({:.3%} of Earth's mass). It is home to {:,} Plutonians.".format(
planet, pluto_mass, pluto_mass / earth_mass, population,
)
# "Pluto weighs about 1.3e+22 kilograms (0.218% of Earth's mass). It is home to 52,910,390 Plutonians."
'''
Python-字符串
原创
©著作权归作者所有:来自51CTO博客作者youyeye的原创作品,请联系作者获取转载授权,否则将追究法律责任
下一篇:JS-三元表达式
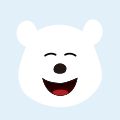
提问和评论都可以,用心的回复会被更多人看到
评论
发布评论
相关文章
-
Python-字符串处理函数
Python字符串操作函数小结
Python 字符串函数 -
Python-初学笔记之字符串
字符串的的简单笔记
字符串 占位符 百分号 -
Python-字符串的常用方法
字符串常用方法1.判断方法说明isspace()如果string中只包含空格,则返回Trueisalnum()如果stri
字符串 列表 python 正则表达式 java